URL Shortener: TypeScript, PostgreSQL, Koa.Js, REST and MVC
- Description
- Curriculum
- FAQ
- Reviews
Dive into modern web development as you build a feature-rich URL Shortening Service from the ground up. This comprehensive course is designed to provide you with a thorough understanding of TypeScript, PostgreSQL, Koa.js , REST, Knex.Js, and the MVC design pattern, as well as practical experience in applying these technologies and concepts to a real-world project.
In this course, you will start by setting up your development environment and configuring the required tools for an optimal workflow. You will then dive into database configuration and management using PostgreSQL, learning how to create and execute migrations to set up your database schema. The course also covers the use of TypeScript to create efficient and scalable models that interact with the database, as well as the implementation of services to handle URL-related operations and other application logic.
As you progress through the course, you’ll explore user authentication and authorization, covering topics such as user registration, password hashing, and JWT-based authentication for secure access to protected resources. You will also learn how to develop a RESTful API using the Koa web framework and Koa Router, creating routes and middleware for various application functionalities.
Throughout the course, you will be guided by hands-on examples and practical exercises that will reinforce your understanding of the topics covered. By the end of this course, you’ll have developed a solid foundation in modern web development technologies, enabling you to create efficient and robust applications using TypeScript, PostgreSQL, Koa, and the MVC design pattern. With a completed URL Shortening Service project to showcase, you’ll be well-prepared to tackle future web development challenges with confidence and expertise
-
4Database ConfigurationVideo lesson
Introduction to the database and its configuration, as well as setting up the connection.
-
5Environment VariablesVideo lesson
Learn about environment variables and how to use them for database configuration.
-
6Migrations OverviewVideo lesson
Overview of migrations and their role in managing the database schema.
-
7Users MigrationVideo lesson
Creating a migration for the Users table and defining its schema.
-
8URLs and Visits MigrationsVideo lesson
Creating migrations for the URLs and Visits tables and defining their schemas.
-
9Testing KnexVideo lesson
Learn how to test and ensure the database is set up correctly.
-
10TypeScript ModelsVideo lesson
Introduction to TypeScript models and how they are used to represent data and inject them into Knex.
-
11URL Service Part 1Video lesson
Building the URL service for managing URL-related functionality.
-
12URL Service Part 2Video lesson
Further development of the URL service, including additional methods and error handling.
-
13Validation Part 1Video lesson
Introduction to input validation and implementing basic validation rules.
-
14Validation Part 2Video lesson
Further development of validation rules and custom validators.
-
15Validation Part 3Video lesson
Finalizing validation rules and incorporating them into the application.
-
16Exceptions ThrowingVideo lesson
Learn how to throw and handle custom exceptions in the application.
-
17Visits ServiceVideo lesson
Learn how to manage and track visits to the URLs.
-
18User RegistrationVideo lesson
Implementing user registration and storing user data in the database.
-
19Password HashingVideo lesson
Learn about password hashing and how to securely store user passwords.
-
20User LoginVideo lesson
Implementing user login.
-
21JWT (JSON Web Tokens)Video lesson
Introduction to JWT and how to use it for token-based authentication.
-
22Koa IntroductionVideo lesson
Introduction to the Koa framework and its features.
-
23Koa RouterVideo lesson
Setting up the Koa router and defining application routes.
-
24Authentication RouterVideo lesson
Implementing the authentication router and its endpoints.
-
25Authentication MiddlewareVideo lesson
Building the authentication middleware to handle user authorization.
-
26URLs RouterVideo lesson
Implementing the URLs router and its endpoints.
-
27Visits and RedirectVideo lesson
Managing visits and implementing URL redirection
-
28Koa V.S ExpressVideo lesson
Why we used Koa and the differences between Koa and Express
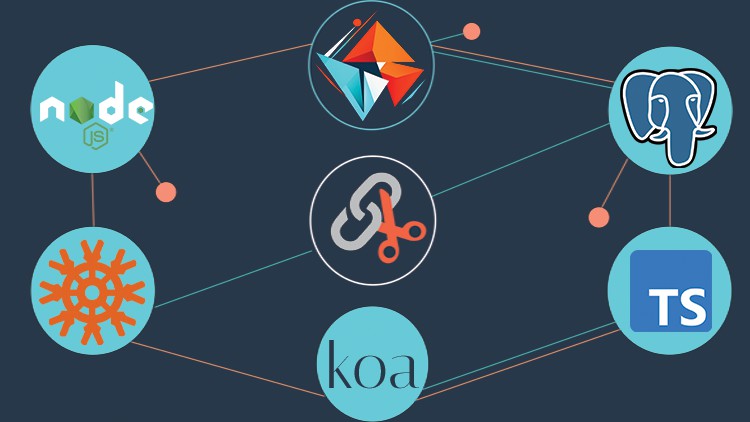
External Links May Contain Affiliate Links read more