The Complete Python 3 Course: Beginner to Advanced!
- Description
- Curriculum
- FAQ
- Reviews
If you want to get started programming in Python, you are going to LOVE this course! This course is designed to fully immerse you in the Python language, so it is great for both beginners and veteran programmers! Learn Python as Nick takes you through the basics of programming, advanced Python concepts, coding a calculator, essential modules, creating a “Final Fantasy-esque” RPG battle script, web scraping, PyMongo, WebPy development, Django web framework, GUI programming, data visualization, machine learning, and much more!
We are grateful for the great feedback we have received!
“This course it great. Easy to follow and the examples show how powerful python can be for the beginner all the way to the advanced. Even if the RPG may not be your cup of tea it shows you the power of classes, for loops, and others!”
“Good course even for non-programmers too.”
“It’s really well explained, clear. Not too slow, not too fast.”
“Very thorough, quick pace. I’m learning A TON! Thank you :)”
“Good explanation, nice and easy to understand. Great audio and video quality. I have been trying to get into Python programming for some time; still a long way to go, but so far so good!”
The following topics are covered in this course:
-
Programming Basics
-
Python Fundamentals
-
JavaScript Object Notation (JSON)
-
Web Scraping
-
PyMongo (MongoDB)
-
Web Development
-
Django Web Framework
-
Graphical User Interface (GUI) Programming (PyQt)
-
Data Visualization
-
Machine Learning
This course is fully subtitled in English!
Thank you for taking the time to read this and we hope to see you in the course!
-
1Introduction to PythonVideo lesson
Hello everyone and welcome to the first video of this Python tutorial series. This tutorial series is going to be aimed at somebody who may have basic knowledge about what a programming language is, but this would be your first programming language.
Python is a great programming language to start with! It does have it's limitations, and sometimes it is not the right tool for the job, but Python offers people who have no experience programming a great way of understanding what a programming language is and how to use one.
Throughout this series of videos we will discuss what Python is, how to get it installed, how to run a Python script, the basic syntax, and then get into the language itself. We will finish by writing a fully functional program. I hope everyone enjoys this Python tutorial series and finds it useful!
-
2Mac/Linux installationVideo lesson
The first thing that we need to do to get into programming with Python is installing it. In this video we will discuss the three different methods for installing Python on your system. First, we will discuss MacOS because that is the system I will be using. So, what we're going to do is open up the terminal and run a Ruby command to download and install Homebrew:
/usr/bin/ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)"
Homebrew is a package manager that enables you to install various programs without having to search for a source on the internet. If you have used Linux, using a package manage should be very familiar to you. You can find more information on Homebrew at https://brew.sh/. Next, we will run the following command in terminal:
brew install python3
MacOS does ship with a version of Python 2 pre-installed, but we will be using Python 3 in this tutorial series. To verify that Python 3 was installed, run the follow command:
python3 --version
If you are using Ubuntu, or some other Debian based Linux distribution, you are going to open up a terminal window and run the following command:
sudo apt-get install python3
Similar to Mac, you can type python3 --version to verify that it was installed correctly. Fedora now comes with Python 3 as a system dependency, and if you are using RHEL/CentOS you will type the following command in terminal to install Python 3.6.1:
sudo yum install python36u
If nothing seems to happen or if you receive an error message, check to see if Python 3 is already installed on your system. Lastly, to install Python 3 on Windows you will need to go to https://www.python.org/downloads/. You will download either the 32 or 64 bit version depending on your operating system, and proceed through the installation. I will cover how to install Python 3 on Windows 10 in the next video.
-
3Windows setupVideo lesson
So, we have learned about installing and using Python within MacOS and Linux, and those two operating systems have a similar process because they're both derived from the UNIX operating system. However, setting up Python on Windows is a bit different, so in this video we will walk-through the process. First, go to https://www.python.org/downloads/ and select the latest Python 3 version. If you need to check whether you have a 32 or 64 bit system, right-click on the Windows icon on the bottom left of your desktop, and select system. Once you have downloaded the file, open up the installer and make you check the box to add Python to path, and then click install now. This procedure should work for Windows 7, 8, and 10.
I go into great detail covering how to verify that the PATH is set to the correct version of Python, and installing new packages using pip in the command prompt, but I think it is much easier to show the steps in the video.
-
4Interpreted vs. compiled programming languagesVideo lesson
In this video we are going to talk about the difference between an interpreted programming language and a compiled programming language. Now, this may be a bit adept for the novice programmer, but just stick with us. So, first, with a compiled programming language you write your code, you save it into a file, and it is not yet executable. For example, let's say you are writing C++ code, you write a script, and if you try to open that file with the .cpp extension (which is for C++ files), it is just going to open that in a text editor or code editor. What you need to do with a compiled programming language, is once you save your file you need to compile it into a language that the computer can read, so binary (zeros and ones), and by compiling this filing into and executable file, then you can double click it and it will run. This is where you will get the .exe file for Windows.
So, with Python you can write a script and you can instantaneously run that script without having to compile it into binary. When you run Python scripts you're going to run it with the Python command, and then the name of the file, and what happens is you're running the program Python which is interpreting your code in real-time. So, it does compile your code into binary, but it does what is called just-in-time compilation. The interpreter is great to use if you want to test something quickly, or if you want to debug a few lines of code.
-
5Creating and running our first Python scriptVideo lesson
Let's create a Python script and learn how to run it. I'm going to be doing this within terminal, and you can follow along on MacOS, Windows, or Linux. First, I am going to change into my "pycharm projects" directory and there's nothing really here, so I'm going to create a file using the nano text editor. If you are Windows, you will not have access to nano in the command prompt. I'm just going to call the file test.py. All Python scripts need to have the .py file extension. We will use the classic programming example of print("Hello World"). I will save the file, and if we just type "test.py" you will see that BASH doesn't know what to do with this command since test.py is not an installed program. What we need to do is run:
python3 test.py
and it will return the result of anything within the script. So, that was a quick video on how to run Python scripts in terminal, in the next video we will setup our integrated development environment.
-
6Choosing an integrated development environment (IDE)Video lesson
What kind of working environment do we need to be efficient at programming with Python? Feel free, if you would like, to open up a basic text editor, write a script, and then go to the terminal or command prompt and run it. That would be very inefficient for a variety of reasons, and so we're going to be using an integrated development environment (IDE). The IDE we will be using in this tutorial series is called PyCharm, and it was built by a company called JetBrains. The community version is completely free and you can download it here:
https://www.jetbrains.com/pycharm/download/
PyCharm utilizes Java, so if it isn't already installed you can find it here:
Let's take a quick tour around PyCharm and then get into the language itself.
-
7How to share your code with us and get help with errorsVideo lesson
If you need help feel free to ask us questions within the course discussion forum. If you need help with your code you can share it via Pastebin and use the Python syntax highlighting tool.
-
8Basic types - numbersVideo lesson
Before we actually get into functional programming with Python we need to discuss some basic types, and then variables, and some other stuff. So, in this video we 're going to be covering numbers. So, what is a number? Well, I'm sure everyone knows what a number is. We have all been through grade grade school. For our purposes, there are two types of numbers - integers and floating points. A floating point is basically any number that has a decimal included in it. In this video we will write some numbers on the screen, perform a few mathematical operations, and convert numbers into strings.
-
9Basic types - stringsVideo lesson
Alright, so what is a string? A string is any text that you want to be treated as text within a program. This is a string because it's wrapped in quotations:
"Hello String"
You can also use single quotations to create a string. In this video we will write a few different string variations which include quotes and apostrophes. In the next video we will continue with string manipulation.
-
10Basic string manipulationVideo lesson
What are some of the fun and useful things that we can do with strings in Python? Go ahead and open up a either your IDE, terminal, or command prompt and follow along. I find that if you're simply told to do something you may not remember the procedure next time. However, if you actually perform that actions yourself, it is much more likely to stick. So, the first thing we are going to cover is concatenating strings. Concatenating is essentially gluing two things together. Below is an example of concatenating two strings together:
"Hello, " + "Nick"
As you can see, you concatenate two strings by using the "+" operator. You can also use the string function to convert an integer to a string like so:
"This costs" + str(6) + "dollars
You can also perform mathematical operations within the string function parameter.
"This costs" + str(6+5) + "dollars"
So, how do we do the opposite of concatenation? We can split strings by using the ":" operator.
"Hello:Nick"
This all may not seem very exciting, and you may or may see how this could be useful, but you will see later on why concatenating is useful when coding.
-
11Basic types - Boolean operatorsVideo lesson
In this video we're going to be talking about Boolean operators. So, what is a Boolean? This is a general programming concept, and a Boolean consists of two items - true and false. Basically, truth checking. So, let's have a look at some of the ways we can generate a true or false. Let's start with 5=5. In math terms this is true, but a single equal sign is used to assign a value to a variable in Python. When comparing two numbers or strings you will need to use double equal signs like so.
5==5
Python will return True. If we try 5==4, Python will return False. We can also write the following:
5 is 5
Python will return True. If we were to write 5 is 4, Python will return False. Similarly, 5 is not 4 will return True. We can also compare strings.
"I like pizza"=="I like pizza"
Python will return true. In the next video we will cover Python's version of arrays which are called lists.
-
12Lists (arrays)Video lesson
In this video we are going to discuss lists and what they are in Python. So, if you have experience with programming in other languages, let's say PHP, you know to create an array, and what an array is. For those who do not have experience programming, an array is a way of keeping data organized and within a single construct. For single-dimensional arrays, we can implement it as a list in Python. To create a list in Python we use square brackets:
["Movies", "Games", "Python"]
This becomes a list that has 3 indexes. To call the first item in the list you write the following:
["Movies", "Games", "Python"][0]
Remember, when programming the first ID of the item in a list or an array will be 0. We can also concatenate a list item with a string.
print("I Like" + ["Movies", "Games", "Python"][0])
If we were to change the index to number to 1 it would print out "I Like Games". So, that is what a list is in Python. It's just a way to create a collection under one variable. In the next video we will cover dictionaries.
-
13DictionariesVideo lesson
In this video we will cover dictionaries in Python. In the previous video when we created a list we used square brackets and comma separated values. To create a dictionary we will use curly brackets instead of the square brackets. In a previous video I may have mentioned that we do not use curly brackets in Python, and that was a lie. What I meant is that we don't need to wrap code blocks in curly brackets like PHP or JavaScript. So, let's go ahead and define a dictionary.
{"name": "Nick", "age": is 27, "hobby": "code"}
As you can see, instead of just comma separated values we used in the list, the dictionary uses a key and a value separated by a colon. You can access an item in the dictionary like so:
{"name": "Nick", "age": is 27, "hobby": "code"}{"name"}
Python will then print out "Nick." Dictionaries will be very important when we get to the JSON part of the course, because we will need to parse though a ton of data.
-
14VariablesVideo lesson
In this video we are going to cover variables in Python, and as you may have guessed, a variable is an object that is variable in nature. So, why do we use variables in our code? Well, when we explicitly write strings they are not reusable. If I were to simply write "This is a sting," I would be unable to reuse that line of code later on in my script. So, one reason we use variables is for re-usability. Another reason we use variables is for passing values into functions, but we will get into that in a later video. The nice thing about Python is that we don't need to declare or define the type of variable. Creating a variable is as simple as this:
greeting = "hello world"
When you hit enter in your terminal or command prompt, Python will not output a response. However, when we call our new variable, Python will print out "hello world"
print(greeting)
Now that the greeting variable is stored in memory, we can make changes to it.
greeting = greeting.split (" ")[0]
If we print(greeting) now it will returns hello. We can write the following to return hello nick:
print(greeting + " Nick")
In the next video we're going to be talking about some of the built-in functions available to you in Python.
-
15Built-in functionsVideo lesson
In this video we are going to talk about a few of the built-in functions available in Python, and then we're going to get into defining our own functions, and the different parts of a function. So, these are some basic (very basic) functions that we need to learn about. We are not going to get into any specific modules like the math module, or anything like that, I'm just going to demo a few basic functions. A few of these we have already use earlier in the tutorial series.
print
str
int
bool
len
sortedIn the next video we will begin building our own functions.
-
16User-defined functionsVideo lesson
Let's get into user-defined functions. Now, every programming language has functions, however they are going to look different depending on the language. If you have any experience with PHP or JavaScript, functions in Python are going to look similar. So, we are now past the need to use terminal, and from here on out we are going to use our IDE. In addition to functions, we are going to cover a few of the PEP guidelines. PEP is basically a style guide for the Python language, and the first thing we need to do is drop down two lines from the top of the script. To begin defining a function in Python, all you need to do is type the word "def". When naming functions we must be mindful of the PEP guidelines. With Python you will be using snake case, words separated with underscores, when naming your function.
def my_function():
Within the parenthesis we can pass parameters into our function, then we end the line with a colon. So, now we have a function but it doesn't perform any actions, yet.
def my_function():
print("This is my function!")
print("A second string.")my_function()
Now our function will print out two strings. Notice that our IDE automatically indents 4 spaces. When you indent in Python you are creating block of code. When we skip a line and remove the indentation, we are telling Python that our function is completed. Lastly, we will call our function. In the next video we will talk about arguments and how use them inside your function.
-
17Adding arguments to a functionVideo lesson
In this video we're going to expand on functions, and we're going to learn how to implement arguments into our functions. The following function is completely static.
def my_function():
print("This is a function!")
print("A second string.")my_function()
We could call this function 10 times and each time it's going to print out the same strings. So, how can we make our functions more dynamic? The way to do this is by adding arguments, and arguments go in the parenthesis at the end of the function name. So, let's add two arguments in to our function.
def my_function(str1, str2):
print(str1)
print(str2)my_function()
Now if we try to run this we would get an error. Python is looking for the values str1 and str2 and they are undefined. Let's go ahead and define them.
def my_function(str1, str2):
print(str1)
print(str2)my_function("This is argument 1", "This is the second argument which is also a string.")
Now when we call my_function it will print out the two strings. Let's call the function again and print out two different strings.
def my_function(str1, str2):
print(str1)
print(str2)my_function("This is argument 1", "This is the second argument which is also a string.")
my_function("Stringy", "Hello World")As you can see, Python will print out all four strings.
-
18Default argumentsVideo lesson
In this video we were going to discuss arguments a bit further, and we were going to move on to keyword arguments. Before we do that however, it is going to make more sense to cover default arguments first. So, let's go ahead and write a function.
def print_something(name, age):
print("My name is ", name, + " and my age is " + age)print_something("Nick", 27)
This won't run. Remember, when we concatenate strings we must convert integers to strings as well. While we could use the str function to fix our code, it is much easier to separate the four pieces of data with commas.
def print_something(name, age):
print("My name is", name, "and my age is", age)print_something("Nick", 27)
The results will be the same as if we converted the integer to a string and concatenated the four strings together. So, what happens if we only want to pass our function 1 of the 2 values? This is where we can use default arguments.
def print_something(name = "Someone", age = "Unknown"):
print("My name is", name, "and my age is", age)print_something("Nick")
When we run this bit of code, Python will print out My name is Nick and my age is Unknown. As you can see, Python will default to the argument we provided it if no data is given.
-
19Keyword argumentsVideo lesson
Alright! So, let's talk about keyword arguments. The first keyword, or boolean, that we are going to cover is None. Basically, this is the equivalent to null in other languages. So, let's go ahead and write our previous function.
def print_something(name = "Someone", age = "Unknown"):
print("My name is", name, "and my age is", age)print_something(None, 27)
There isn't a way to pass in an argument that isn't first one being used unless we use keyword arguments. We can also define the variable when we call the function.
def print_something(name = "Someone", age = "Unknown"):
print("My name is", name, "and my age is", age)print_something(age = 27, name = "Nick")
By using keyword arguments, we can specify which value is supposed to go in a particular variable.
-
20Infinite argumentsVideo lesson
In this video we are going to discuss passing an infinite number of arguments in to a function. So, let's go ahead and write a function.
def print_people(*people):
So, here we have a function called print_people, and the asterisk tells this argument that it's going to be an array of all the arguments that are passed in to the function. This may result in 1, 100, 1,000, etc. arguments, and we will see how this works in a moment. With an array we need some way to loop over it, and so we're going to be using a for statement.
for person in people:
print("This person is", person)Basically, the for loop allows us to iterate over the people list and pass the values into our function as arguments. Again, if you're familiar with other programming languages this is not a new concept, it's just a slightly different way of doing it. Let's call the print_people function and pass it some names.
print_people("Nick", "Dan", "Jack", "King", "Smiley")
What we're doing here is passing 5 arguments. Now if we knew we were always going to expect 5 arguments, we could accept each individual argument as it's own variable. However, if we don't know the total number of values, we are going to create an array stored in the variable called people. So, that's how to include an infinite, or flexible, number of parameters. In the next tutorial we will cover return values.
-
21Return values from functionsVideo lesson
Welcome back everyone. This is the final tutorial in the subsection for functions, and we're going to be talking about return values from functions. Again, this may not be new to some of you as it is a fairly basic concept. So, in the past videos we have been using functions to print something out, but what if we want to return that value and do something else with it? Let's go ahead and define a function.
def do_math(num1, num2):
return num1 + num2do_math(5, 7)
Now when we run this bit of code, it's not going to print anything in our Python console because we didn't tell it to. Let's add on to this.
def do_math(num1, num2):
return num1 + num2math1 = do_math(5, 7)
math2 = do_math(11, 34)print("First sum is", math1, "and the second sum is", math2)
When we run this we get the First sum is 12 and the second sum is 45. So, again, this a very simple example, but we will expand on this when we build our calculator.
-
22If, elif, else statementsVideo lesson
In this tutorial we are going to be learning about the conditional statements available to you in Python. So, we're going to be learning about the if-else statement, and this is basically provides us a way to evaluate if something is true or false, and then perform something whether or not the condition is met. Let's write an if-else statement.
check = True
if check == False:
print("It is false")
else:
print("It is actually equal to True")This is going to print out "It is actually equal to True" in the console. First, Python is going to check if the variable is equal to false. Else, it's going to continue through the script and print, or perform, whatever we tell it to do. This is great if we only have two conditions, but what if we want to use more than two? Between the if and else we can use an elif statement, and here we can supply an additional condition.
check = Hamburger
if check == False:
print("It is false")
elif check == "Hamburger":
print("Yummm, hamburgers")
else:
print("It is actually equal to True")When we run this script it's going check to see if the first condition is true, if not it will check the next condition, if not it will print the else statement. So, that's what an if-statement is, and these are necessary for the project we are about to begin working on.
-
23For/while loopsVideo lesson
In this tutorial we are going to cover the two loops types in Python. The first one is a for loop. A for loop will allow you to iterate over a list in Python. In other words, you can do something for each item in the list. So, let's go ahead and create a list.
numbers = [1, 2, 3, 4, 5]
for item in numbers
print(item)When we run this each number in our list will be printed out in the console. Let's add names to our list instead of numbers.
names = ["Nick", "Someone", "Another Person"]
for item in names
print("This persons name is", item)That is a for loop, and basically the second parameter is to access the list, and then the first parameter is what you want each item in the list to be called while inside it's little block of code. Now we're going to learn about a while loop.
run = True
current = 1while run:
if current == 100:
run = False
else:
print(current)
current += 1In this bit of code we are creating two variables, while, and then we write what we want to happen while the program is running. In this case, we are going to check to see if current = 100. If not, we are going to add 1 to the total, and we are starting from 1. Once the total hits 100 the program will stop running. We will be using loops quite a bit throughout this course, so make sure you've mastered this concept.
-
24Importing libraries into a scriptVideo lesson
In this tutorial we are going to learn how to import different modules into a Python script. So, what is a module? A module is an external library that you can include and use in your project, without having to write the additional functionality yourself. Let's import the regex library.
import re
Re is included with Python, so there's nothing that we need to install in order to use it. Regex is basically a mini-programming language that you can use within most other languages. Regex gives us a way to match certain characters and then do something based on that. Let's cover some basic re usage.
string = "'I AM NOT YELLING', she said. Though we knew it to not be true."
print(string)
This particular string has capital letters, lower-case letters, a comma, a period, and quotations. Let's play around with this a bit.
new = re.sub('[A-Z]', '', string)
What we're going here is instantiating the re object that we imported at the top of the script, and we're calling the sub, or substitute, function on the re object. So just like calling any other function, we need to provide parameters to the object. We haven't discussed classes or objects yet, and we will get to them later, but this is what we need to know for the sake of this video. The three parameters that this substitute function will take is the matches we want to make, what we want to replace them with, and then string that we're going to manipulate. Take note that rules in regex are contained within square brackets.
print(new)
As you can see, we took the capital letters A-Z and replaced them with blank space. There are all sorts of different applications for regex, and we will be using it when creating a calculator in the next video.
-
25Project #1 - Building a calculatorVideo lesson
Welcome back everyone! We are on the last video of this tutorial series which means you now have a basic understanding of Python. You actually have enough knowledge right now to start building basic programs. We have covered some of the core concepts, as well as the language syntax, and we currently know how to create loops, if-elif-else statements, variables, etc. So, we are going to finish off the series with a project, specifically building a calculator in Python. Let's go ahead an open up our ide.
import re
print("Our Magical Calculator")
print("Type 'quit to exitn'")previous = 0
run = Truedef performMath():
global run
global previous
equation = ""
if previous == 0:
equation = input("Enter equation:")
else:
equation = input (str(previous))if equation == 'quit':
print("Goodbye, human.")
run = false
else:
equation = re.sub('[a-zA-Z,.:()" "]', ' ', equation)if previous == 0:
previous = eval(equation)
else:
previous = eval(str(previous) + equation)while run:
performMath()At the top of the script we are going to import the regex library, write a print statement welcoming our user, and inform our user how to exit the program. Next, we will define the previous and run variables. The previous variable will define the default value upon starting the program, and the run variable will determine whether the program is running or not.
Next, we will get into the meat of our calculator program. We will begin by defining the performMath function. Since the run and previous variables do not exist within our function, we will need to import them as global variables. Finally, we will define the equation variable.
Now that the performMath function is created, we will need to tell it what to do. The first if-else statement will request an input from the user. The second if-else statement will tell the program how to handle the user input. If the user types "quit", the program will end and print "Goodbye, human." Otherwise, the program will run a regex request on the input. Remember from the previous tutorial, we can use the regex library to identify and replace different sets of characters. We do not want the user inputting anything other than basic math. In the same block of code, we are going to run the eval function on the input from the user. If the user has already run a calculation, our program will take that result and add it to any additional calculations.
Lastly, we will create a while loop to run our performMath function. This is a very basic program and I would be interested to see any additions you make to it! Thank you to everyone who followed along with this tutorial series. I hope you found the information valuable!
-
26PEP guidelinesVideo lesson
Now that we have covered the basics of programming, we can get into some more advanced Python concepts. Let's begin with the PEP guidelines, and this is because throughout this course you're not only going to have to get use to the syntax of Python, but you're going to have to get used to writing it properly.
-
27Breaking out of while loopsVideo lesson
In this lecture we're going to talk about some while loop additional functions, or additional handlers.
-
28Continuing while loopsVideo lesson
Let's talk about the continue statement which is the opposite of break.
-
29Classes & objectsVideo lesson
A class is a blueprint for objects. So rather than having to create manually the same object over, and over, and over, we can use a class to set certain variables and functions that are going to be the same across all instance instantiations of this class called an object.
-
30Instance variablesVideo lesson
Let's take a look at instance variables and how we can implement them in our project.
-
31Class & instance variablesVideo lesson
An instance variable is a variable that is different or can be different for every instance of the class.
-
32How to add comments to your codeVideo lesson
Including comments in your code is not only important for keeping track of what you are doing, but for other developers who you share your code with as well.
-
33Importing modules from relative pathsVideo lesson
Importing modules that we define ourselves from relative paths will help keep our code readable.
-
34RPG setupVideo lesson
Let's get started on our 2nd project in this course, a RPG battle script game.
-
35Creating our characterVideo lesson
Continuing on with our game.
-
36Additional utility classesVideo lesson
Continuing along.
-
37Enemy instantiationVideo lesson
Let's create an enemy to battle in our RPG.
-
38Using magicVideo lesson
An infinite battle isn't very fun, let's determine the winner and loser.
-
39Turning our magic into a classVideo lesson
Let's turn our magic system into a class.
-
40Healing our playerVideo lesson
In this video we will create spells to heal our player.
-
41Adding itemsVideo lesson
Let's add items to our RPG.
-
42Implementing different item typesVideo lesson
In this video we will be implementing different item types into our RPG.
-
43Better HP viewVideo lesson
Let's code an easier to read HP view for when we have multiple party members in our RPG.
-
44Adding members to our partyVideo lesson
In this tutorial we will be adding additional members to our party.
-
45Working HP barsVideo lesson
Let's setup working HP bars for our party.
-
46White space in HP & MPVideo lesson
Let's continue by creating dynamic HP/MP bars that display missing health.
-
47Enemy HP barVideo lesson
Let's setup the enemy HP bar.
-
48Multiple enemies pt.1Video lesson
In this tutorial we will create multiple enemies (pt.1/2)
-
49Multiple enemies pt.2Video lesson
In this tutorial we will create multiple enemies (pt.2/2)
-
50Enemy artificial intelligenceVideo lesson
In this video we will add an enemy artificial intelligence (AI) system.
-
51Reading and writing files in PythonVideo lesson
Let's get into a form of data persistence, and what I mean by that is we want the ability to open and read and write files in Python.
-
52JavaScript Object Notation (JSON)Video lesson
In this video we're going to be learning about reading and writing files, as well as manipulating existing file contents.
-
53Using Virtualenv to create a virtual environmentVideo lesson
Setting up a virtual environment will allow us to use packages for a project without installing them globally.
-
54The Python Package Index (PyPI)Video lesson
-
55Introduction to RequestsVideo lesson
Let's cover essential modules and packages to extend the programming language of Python. The first package we're going to cover is Requests which is an HTTP library.
-
56HTTP GET variablesVideo lesson
In this lecture we're going to learn about parameters and how to pass GET variables.
-
57Pillow the image processing library (PIL)Video lesson
Let's take a look at the Python Imaging Library (PIL) and how it adds image processing capabilities to your Python interpreter.
-
58Posting dataVideo lesson
In this video we will cover how to post specific data to a URL.
-
59Posting JSONVideo lesson
What we're going to do now is get a JSON response from requests, and it's basically the same as just parsing regular JSON.
-
60HeadersVideo lesson
The last part of the Requests package that we're going to be discussing is headers.
-
61Beautiful SoupVideo lesson
This module will help you get started with web scraping tasks using Beautiful Soup.
-
62Parsing our soupVideo lesson
Now that we have our soup, we will learn how to extract relevant data from it.
-
63Directional navigationVideo lesson
Let's continue on with Beautiful Soup and learn how to properly navigate web pages.
-
64Image scraperVideo lesson
For this project we are going to combine the Beautiful Soup, Requests, and Pillow packages to scrape images off of the internet.
-
65Improvements to our web scraperVideo lesson
Let's finish up our web scraper and make some improvements to it.
-
66Introduction and setupVideo lesson
Let's install PyMongo a Python binding for the program MongoDB. MongoDB is a NOSQL relational database driver.
-
67Inserting documentsVideo lesson
Let's setup another script to connect to our Mongo database and insert a document into a collection.
-
68Bulk insertsVideo lesson
In this video I will show you how to insert multiple documents at the same time.
-
69Counting documentsVideo lesson
Counting documents in our database.
-
70Multiple find conditionsVideo lesson
In this video we're going to just talk about how to use multiple different fields and values to grab objects.
-
71Datetime and keywordsVideo lesson
Using datetime with Pymongo to capture the date & time of objects put into our database.
-
72IndexesVideo lesson
Indexes are essential for scalability with database management.
-
73Introduction and simple exampleVideo lesson
What is Web.py and how can we use it? Web.py is a framework in Python which can serve up web content using the HTTP protocols and it runs it's own server. You write code in Python to define routes based on URL's and then you run the application, and you can do all your logic in Python.
-
74HTML templatesVideo lesson
Let's learn how to create HTML templates.
-
75Building a MVCVideo lesson
Throughout this section we are going to be build a website that has dynamic data, and we're going to be setting our project as a model view controller (MVC). This first entry to our app that defines the routes is going to be the controller, and the controller is going to go and access other files called the model, and it's going to collect the data that's going to be generated onto the page, and then once it has all the data it's going to that to send all that data to the view or the HTML template.
-
76Importing static filesVideo lesson
Let's talk about re-usability and not duplicating efforts. So what that means is if you are writing a static HTML page and all the pages had the same header bar or nav bar, you'd have to copy and paste them into each file. In this video we will create our MainLayout to reuse HTML.
-
77Setting up a register formVideo lesson
Let's begin building our register form.
-
78Posting data to web.pyVideo lesson
In this video we are going to get into form submitting in Web.py.
-
79Creating usersVideo lesson
In this lecture we are going to create a register model so that users can create an account on our site.
-
80Hashing passwordsVideo lesson
Let's use bcrypt to hash the passwords in our Mongo database. Stored passwords should always be encrypted.
-
81Login logicVideo lesson
In this lecture we're going to build out the outworking login system.
-
82Web.py sessionsVideo lesson
A session is a way of keeping persistent data synced up between a client, or an individual user, and the server.
-
83Logout functionalityVideo lesson
Not that users are able to login, let's build the logout function.
-
84Posting microblogsVideo lesson
Let's being work on our Posts model.
-
85Retrieving post objectsVideo lesson
Now that we've got our posts in the database they're entirely useless to us because we can't see them on the front end. So what we're going to do is we're going to turn this little static code right here into a dynamic repeating content based on all posts that we bring out of the database.
-
86User settings and updating MongoVideo lesson
in this video we're going to be learning about how to modify user settings on our site, and how to store settings using PyMongo.
-
87Relative datetimesVideo lesson
Let's talk about relative datetimes because they're so important in today's day and age. People don't like to see full datetimes printed out, like in Unix time or anything, they want to see relative dates. So instead of seeing September 15, 2016 at 11:18 am, what they would like to see is posted 1 minute ago.
-
88Making our post dates prettyVideo lesson
In this lecture we're going to be adding a relative timestamp to each one of our posts.
-
89Adding post commentsVideo lesson
In this video we will give users the ability to comment on the posts on our site.
-
90Image uploads and avatarsVideo lesson
This is the last video in the Webpy section, and we're going to be learning how to upload files, store them onto a database, store the file path in the database, and then use those images as the avatar and background images for a user.
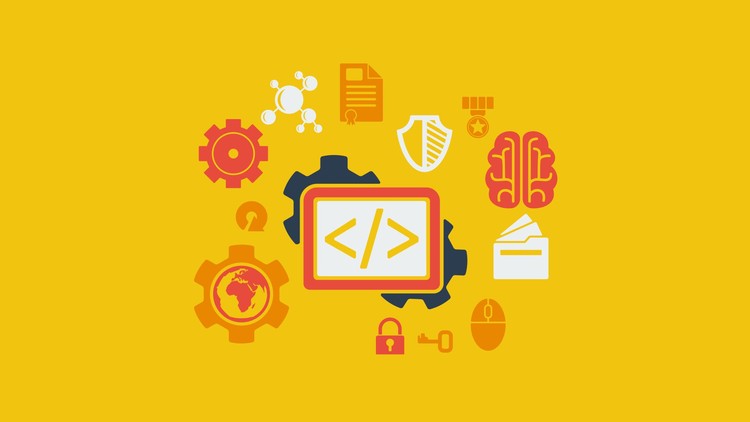
External Links May Contain Affiliate Links read more