Python Programming - For Every Beginners
- Description
- Curriculum
- FAQ
- Reviews
This course is aimed at offering the fundamental concepts of core Python programming language to the all levels of learners.
You will learn through videos, visual organizers and practice exercises. For a great hands-on learning experience, this course is packed with assignments, assessment tests, code challenges, quizzes, and exercises.
This course is divided into two parts:
Part-1: Core Python Programming Basics
Part-2: Core Python Programming Advanced Concepts
Part-1: Core Python Programming Basics– starts with the basics of Python programming concepts like introduction, history & versions, features, uses, applications of python, data types, operators and control flow statements.
Part-2: Core Python Programming Advanced Concepts – starts with strings; Python Data Structures-lists, sets, tuples and dictionaries; functions; object oriented programming concepts; exceptions, files; and modules.
The objective of this course is to enable the learners to develop the applications using the concepts of Python.
Upon completion of the course, the learners will be able to achieve the following outcomes:
Part-1: Core Python Programming Basics:
********************************************
1. Understand the basic concepts in Python programming.
2. Learn how to write, debug and execute Python program.
3. Develop algorithmic solutions to simple computational problems.
4. Develop simple Python programs for solving problems.
5. Apply the conditional execution of the program.
Part-2: Core Python Programming Advanced Concepts:
************************************************************
1. Create, run and manipulate Python Programs using core data structures like Lists, tuples and Dictionaries.
2. Demonstrate proficiency in handling Strings and File Systems.
3. Design solutions for real time problems using object oriented concepts in Python.
4. Implement database and GUI applications.
5. Use and apply the different libraries available in python.
5. Use various applications using python.
-
1AV on PythonVideo lesson
Outline:
********
Overview
Categories of Programming Languages
What is Python?
Python History & Versions
Uses of various programming languages
Uses of Python
Applications of Python
Static & Dynamic typed programming languages
-
2Introduction to PythonVideo lesson
Outline:
********
Overview
What is Python?
History and versions of Python
Features of Python
Applications for Python
Who uses Python Today
Programming Paradigms
-
3Python Installation & Getting StartedVideo lesson
Outline:
********
Python Environments [Editors]
Python Installation in Windows
Python Installation in Unix/Linux/Ubuntu Operating Systems
Flavors of Python
Writing and Executing First Python Program
Determining Python Version
-
4Print Function in PythonVideo lesson
Outline:
********
Python print() function
print() function syntax
print() function parameters
print() function demo in Python
-
5Comments, Indentation and Docstrings in pythonVideo lesson
Outline:
********
Overview
Comments in Python
Indentation in Python
Docstrings in Python
Summary
-
6Keywords, Identifiers, Variables & Built-in Functions in pythonVideo lesson
Outline:
********
Keywords in Python
Identifiers in Python
Variables in Python
Multiple Assignment
Constants in Python
Backslash Character ()
Built-in Functions in Python
Summary
-
7Data Types in pythonVideo lesson
Outline:
********
Variable Type
Variable Assignment
Data Types
Basic Data Types
********************
integer (int)
float
int & float live demo in Python 3.10.1
complex
complex live demo in Python 3.10.1
boolean (bool)
boolean (bool) live demo in Python 3.10.1
string (str)
string (str) live demo in Python 3.10.1
bytes
bytearray
range
none
Container Types (Overview):
*********************************
list example demo
tuple example demo
set example demo
dictionary (dict) example demo
-
8Arithmetic OperatorsVideo lesson
Outline:
********
Introduction to Operators
Types of Operators
Arithmetic Operators
Arithmetic Operators demo in Python 3.10.1
Summary
-
9Relational / Comparison OperatorsVideo lesson
Outline:
********
Introduction to Relational/Comparison Operators
Various Relational/Comparison Operators
Example calculations of Relational/Comparison Operators
Relational/Comparison Operators demo in Python 3.10.1
Summary
-
10Logical OperatorsVideo lesson
Outline:
********
Introduction to Logical Operators
Various Logical Operators
Example calculations of Logical Operators
Logical Operators demo in Python 3.10.1
Summary
-
11Bitwise OperatorsVideo lesson
Outline:
********
Introduction to Bitwise Operators
Various Bitwise Operators
Example calculations of Bitwise Operators
Bitwise Operators demo in Python 3.10.1
Summary
-
12Assignment OperatorsVideo lesson
Outline:
********
Introduction to Assignment Operators
Various Arithmetic Assignment Operators
Example calculations of Arithmetic Assignment Operators
Various Bitwise Assignment Operators
Arithmetic Assignment Operators demo in Python 3.10.1
Bitwise Assignment Operators demo in Python 3.10.1
Summary
-
13Membership Operators | Identity Operators | Precedence of operatorsVideo lesson
Outline:
********
Introduction to Special Type of Operators
Membership Operators
Membership Operators -Example Python programs
Identity Operators
Identity Operators-Example Python program
Precedence of Operators
Summary
-
14introduction to input functionVideo lesson
Outline:
********
Reading data from keyboard
Introduction to input() function
Syntax of input() function in python
input() function - Example Python programs
type casting in input function - Example Python programs
Summary
-
15input() function Python Programs - LIVE DEMOVideo lesson
Outline:
********
Program-1: Addition of two numbers
Program-2: Finding area of rectangle
Program-3: Finding area of circle
Program-4: Finding area of triangle
Program-5: Students Marks and average
Program-6: Customer Current bill calculation
Summary
-
16Conditional Statements-1: if | if-else | if-elifVideo lesson
Outline:
********
Introduction to conditional control statements
if statement
if-else statement
if-elif statement
Summary
-
17Conditional Statements-2: if-elif-else | nested if | single line ifVideo lesson
Outline:
********
if-elif-else statement
nested if statement
single line if statement
Summary
-
18while loopVideo lesson
Outline:
********
Introduction to Iterative Statements / Loops
while loop syntax
while-else syntax
Usage of while loop
while loop example python programs
while-else example python programs
-
19for loopVideo lesson
Outline:
**********
for loop - introduction
range() function
for loop syntax
for loop example python programs using range()
for loop example python programs using string, list, tuple, set and dictionary
for-else statement
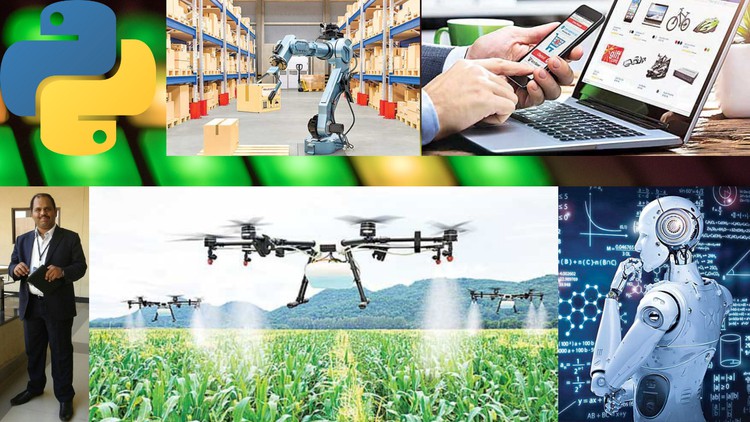
External Links May Contain Affiliate Links read more