Python Pro Bootcamp Zero to Hero
- Description
- Curriculum
- FAQ
- Reviews
Course Description:
Welcome to the Master Python Programming Bootcamp, an all-inclusive course designed to transform you from a complete beginner to a proficient Python programmer. Whether you’re just starting your coding journey or looking to elevate your skills to the next level, this Bootcamp is meticulously crafted to guide you through every step of learning Python.
Course Overview:
Python is one of the most versatile and widely-used programming languages in the world, known for its simplicity, readability, and powerful capabilities. This Bootcamp will take you on a comprehensive journey through Python, covering everything from basic syntax and foundational concepts to advanced programming techniques, real-world applications, and industry best practices.
What You’ll Learn:
1. Introduction to Python:
– Overview of Python and its applications in various industries.
– Setting up your development environment with Python and popular IDEs.
– Understanding Python syntax, variables, and data types.
2. Control Structures:
– Mastering conditional statements (if, else, elif) and loops (for, while).
– Writing efficient and readable code with control flow techniques.
– Practical exercises to solidify your understanding.
3. Functions and Modules:
– Creating and using functions to write modular code.
– Exploring Python’s built-in functions and creating custom functions.
– Understanding scope, recursion, and lambda functions.
– Importing and utilizing modules and libraries.
4. Data Structures:
– In-depth exploration of lists, tuples, dictionaries, and sets.
– Advanced data structure manipulation techniques.
– Working with complex data structures in real-world scenarios.
5. Object-Oriented Programming (OOP):
– Core concepts of OOP: classes, objects, inheritance, and polymorphism.
– Designing and implementing classes and objects in Python.
– Leveraging OOP to write clean, efficient, and reusable code.
– Real-world examples and projects to apply OOP concepts.
6. File Handling:
– Reading from and writing to files in Python.
– Handling different file formats: text, CSV, JSON, and more.
– Practical applications of file handling in data processing tasks.
7. Exception Handling:
– Writing robust code with Python’s exception handling mechanism.
– Understanding and handling different types of exceptions.
– Implementing try-except blocks, raising exceptions, and custom exception classes.
Why This Course is Different:
– Comprehensive Curriculum: This Bootcamp covers every aspect of Python programming, ensuring you gain a complete understanding of the language.
– Project-Based Learning: Engage in numerous real-world projects that reinforce your learning and build a strong portfolio.
– Expert Instruction: Learn from an experienced instructor who brings industry knowledge and practical insights to the table.
– Lifetime Access: Enjoy lifetime access to the course materials, allowing you to revisit and review content at your own pace.
– Supportive Community: Join a community of fellow learners, participate in discussions, and receive support throughout your learning journey.
Who This Course is For:
– Beginners with no prior programming experience who want to learn Python from scratch.
– Intermediate learners looking to deepen their understanding of Python and explore advanced topics.
– Professionals seeking to enhance their skills for career advancement or transitioning into a Python-focused role.
– Students preparing for technical interviews and looking to build a strong foundation in Python programming.
Course Requirements:
– No prior programming experience is required. A passion for learning and a computer with internet access are all you need to get started!
-
1Course IntroductionVideo lesson
Python master Bootcamp
-
2VS code installationVideo lesson
-
3How to run your first code in pythonVideo lesson
-
4Why to learn python language and its featuresVideo lesson
-
5Issues in pythonVideo lesson
-
6Python usecase to understand importance of pythonVideo lesson
-
7Python introduction and Basics QuizQuiz
Test your grasp of Python fundamentals in this comprehensive quiz. From basic syntax and data types to control structures and functions, you'll demonstrate your understanding of Python's core concepts. Tackle questions on variables, operators, loops, lists, dictionaries, and file handling. Perfect for beginners aiming to solidify their Python knowledge and prepare for more advanced programming challenges.
-
8Variables and ConstantsVideo lesson
-
9Naming of variables in pythonVideo lesson
-
10Dynamic binding and dynamic typingVideo lesson
-
11Keywords and identifiersVideo lesson
-
12CommentsVideo lesson
-
13Indentation in pythonVideo lesson
-
14Type conversion ,type castingVideo lesson
-
15Print fucntionVideo lesson
-
16Input functionVideo lesson
-
17String formatingVideo lesson
-
18Eval functionVideo lesson
-
19Python variables QuizQuiz
Python variables are named containers for storing data values. They're created when you assign a value using the "=" operator. Variables can hold various data types like numbers, strings, or complex objects. Python is dynamically typed, so you don't need to declare the type explicitly. Variable names are case-sensitive and must follow certain naming conventions.
-
20Operators in pythonVideo lesson
-
21Arithmetic operatorsVideo lesson
-
22Assignment operators and comparsion operatorsVideo lesson
-
23Logical and bitwiseVideo lesson
-
24Membership and identity operatorsVideo lesson
-
25Python Operators QuizQuiz
Python operators are symbols or keywords that perform operations on values or variables. They include arithmetic (+, -, *, /), comparison (==, !=, <, >), logical (and, or, not), assignment (=, +=), bitwise (&, |, ^), identity (is), and membership (in) operators. These allow you to perform calculations, make comparisons, combine conditions, and manipulate data efficiently in your Python programs.
-
26If , else , elif , finaly keywordVideo lesson
-
27Nested if else statementVideo lesson
-
28Practice question condtional statementVideo lesson
-
29Conditional statement in python QuizQuiz
Conditional statements in Python, such as `if`, `elif`, and `else`, control the flow of execution based on logical conditions. They allow the program to execute specific code blocks when certain conditions are true or false, enabling decision-making and branching in the code.
-
30For loopsVideo lesson
-
31Nested for loopsVideo lesson
-
32While loopVideo lesson
-
33BreakVideo lesson
-
34Continue statementVideo lesson
-
35PassVideo lesson
-
36For loop exercise 1Video lesson
-
37While loop exercise 2Video lesson
-
38Loops in python QuizQuiz
Python offers two main types of loops: 'for' and 'while'. 'For' loops iterate over a sequence (like a list or range) for a set number of times. 'While' loops continue as long as a condition is true. Both allow you to repeat code blocks, process data collections, and create iterative algorithms efficiently.
-
39Data types in pythonVideo lesson
-
40String introductionVideo lesson
-
41String oerprationVideo lesson
-
42String functions and methodsVideo lesson
-
43Escape Sequence characterVideo lesson
-
44Regular expressionsVideo lesson
-
45String interview theory questionsVideo lesson
-
46String interview coding questionsVideo lesson
-
47Data types (string) QuizQuiz
In Python, a `string` is a sequence of characters enclosed in quotes (single, double, or triple). Strings are immutable, meaning their content cannot be changed once created. They support various operations like concatenation, slicing, and methods for manipulation, such as `lower()`, `upper()`, and `replace()`.
-
48List createing , slicing and indexingVideo lesson
-
49List operationsVideo lesson
-
50List methodsVideo lesson
-
51Zip and Enumerate functionVideo lesson
-
52List comprehensionVideo lesson
-
53Del vs None typeVideo lesson
-
54List theory interview questionsVideo lesson
-
55List coding interview questionsVideo lesson
-
56Data types(List) QuizQuiz
Lists in Python are ordered, mutable sequences that can store multiple items of any type. They're created using square brackets [] or the list() constructor. Lists support indexing, slicing, and various methods like append(), remove(), and sort(). They're versatile for storing and manipulating collections of data in Python programs.
-
57Tuples creating and featuresVideo lesson
-
58Tuples packing and unpacking , slicing and indexingVideo lesson
-
59List vs tuplesVideo lesson
-
60Tuple methods , functons and operatorsVideo lesson
-
61Tuple theory questionsVideo lesson
-
62Tuple coding questionsVideo lesson
-
63Data types (tuples) QuizQuiz
Tuples in Python are ordered, immutable sequences that can store multiple items of any type. They're created using parentheses () or the tuple() constructor. Tuples support indexing and slicing, but not item assignment or deletion. They're often used for grouping related data and can be elements in sets or dictionary keys.
-
64Sets introductionVideo lesson
-
65Sets use caseVideo lesson
-
66Sets methods , functions and operationVideo lesson
-
67Set comprehensionVideo lesson
-
68Frozen setVideo lesson
-
69Set theoretical questionsVideo lesson
-
70Set coding questionsVideo lesson
-
71Dictionary creation and featuresVideo lesson
-
72Data types (set) QuizQuiz
Sets in Python are unordered collections of unique elements. They're created using curly braces {} or the set() constructor. Sets don't allow duplicates and support mathematical operations like union, intersection, and difference. They're mutable but can only contain immutable elements. Sets are useful for removing duplicates and testing membership efficiently.
-
73Dictionary methodsVideo lesson
-
74Dictionay function and operatorsVideo lesson
-
75Nested DictionaryVideo lesson
-
76Dictionary comprehensionVideo lesson
-
77Dictionary practice questionsVideo lesson
-
78Data types( dictionary ) QuizQuiz
A dictionary in Python is a versatile data structure that stores key-value pairs. It's unordered, mutable, and uses curly braces {}. Keys must be unique and immutable (e.g., strings, numbers, tuples), while values can be of any type. Dictionaries offer fast lookups and are ideal for representing structured data.
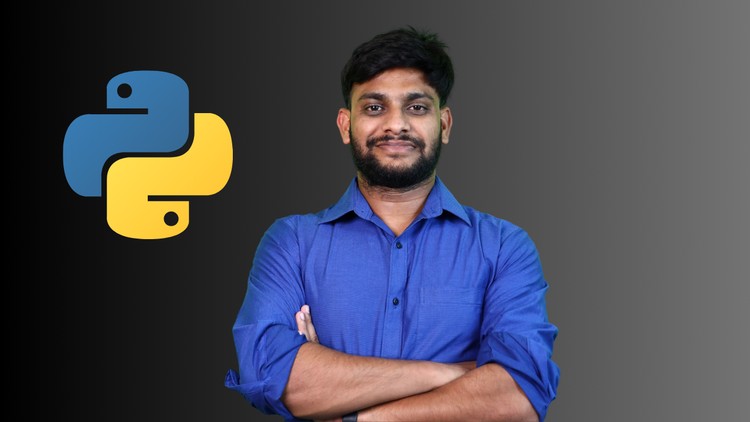
External Links May Contain Affiliate Links read more