Modern C++20: Advanced Multithreading and Concurrency Design
- Description
- Curriculum
- FAQ
- Reviews
Learn about memory models, atomics, and synchronization primitives in C++ and how to use them to write correct, efficient, and high-performance concurrent programs.
This intermediate-level course is designed for experienced C++ developers who want to deepen their understanding of concurrency and memory models in C++. You will learn about the C++ memory model, including the Sequential Consistency-Data Race Free (SC-DRF) guarantee, and how to use it to reason about the behavior of concurrent programs. You will also learn about different memory barriers and how to use them to enforce ordering constraints between memory accesses.
In addition, you will learn about atomics and how to use them to implement efficient synchronization mechanisms in your programs. You will explore the different types of atomics available in C++, including lock-free and wait-free algorithms, and learn how to use them effectively.
The course also covers false sharing in caches, which can lead to significant performance degradation in multi-threaded programs. You will learn how to identify and avoid false sharing in your code.
This course will help you dive deep into designing and implementing efficient concurrent data structures using the latest C++ features and best practices. These are skills that tools like ChatGPT will take years to develop.
The course expects the students to implement the discussed code independently. The course targets self-starters and intermediate-skilled programmers who are interested in nuances of design beyond copy-pasting assignments.
Concurrency is crucial in today’s software development landscape, and creating data structures that multiple threads can safely and efficiently access is essential. In this course, you’ll:
-
Grasp the fundamentals of concurrency and the challenges of designing concurrent data structures.
-
Explore various concurrent data structures and select the most suitable one for your use case.
-
Learn about synchronization techniques and mechanisms in C++ for ensuring thread safety, including mutexes, condition variables, and atomics.
-
Understand different memory models, lock-free and wait-free data structure design, and principles of memory reclamation and garbage collection.
-
Work on practical examples and hands-on exercises to reinforce your knowledge, and design and implement concurrent data structures like queues, stacks, hash tables, and trees using the latest C++ features.
By the end of this course, you’ll be well-equipped to design and implement high-performance concurrent data structures in C++ that can scale on multi-core systems and handle high levels of concurrency.
Discover the benefits of learning concurrency with C++20:
-
Standardized support: Utilize C++20’s new features and enhancements for concurrent programming, including parallel algorithms, atomic operations, and memory models.
-
High-performance: Harness C++’s high-performance capabilities to create fast and efficient concurrent programs.
-
Memory model improvements: Leverage C++20’s new memory model to reason about concurrent program behavior and prevent subtle bugs.
-
Enhanced type safety: Take advantage of improved type safety in concurrent programs with features like the atomic_ref class.
-
Practical applications: Boost your competitiveness in the job market and develop high-performance software that can handle a high degree of concurrency.
Finally, the course provides an overview of performance analysis tools such as perf, Valgrind, Intel Vtune, Google Orbit, and gdb, which can be used to profile, debug, and optimize your code.
Throughout the course, you will work on practical examples and can pursue hands-on exercises independently to reinforce your understanding of the material. By the end of this course, you will have solid experience with memory models and concurrency in C++ and be able to write correct, efficient, high-performance concurrent programs.
-
1Course Structure - Most lectures have code walkthroughs or tool demosVideo lesson
The instructor begins by welcoming students to a niche course focusing on a specific aspect of C++ introduced from C++11 onwards. Students are informed about the organization of the curriculum and advised to set expectations accordingly. The course is conceptual, emphasizing deep understanding rather than quick problem-solving.
Key points covered:
1. The course will delve into a specialized area of C++, starting with basic concurrency concepts.
2. The first section introduces simple concurrency-related topics that are suitable for intermediate-level students but also cater to beginners.
3. Quizzes will be interspersed throughout the sections to test understanding.
4. The course will explore the C++ memory model introduced in C++11, examining its existence and purpose.
5. Students will learn about behind-the-scenes optimizations in code, especially concerning concurrency.
6. The instructor will address the importance of understanding memory barriers in concurrency for code correctness.
7. Performance considerations, atomics, and common misconceptions will be discussed.
8. The course will cover the compare-and-swap feature in modern C++ and its significance in higher-level language abstractions.
9. Memory ordering, essential for coding accuracy, will be explored in-depth.
10. Tools and utilities beneficial for high-performance ecosystems will be demonstrated, focusing on Linux but mentioning availability on other operating systems.
11. Emphasis on the importance of these tools in optimizing server costs, especially with the growing expenses in AI, as many servers run on C++.
12. A practice test will be provided to evaluate students' understanding, with solutions available.
13. The course is positioned as foundational for those pursuing a career as a C++ developer.
The instructor concludes by expressing the depth of the course's focus on concurrency and its significance for a C++ developer's career.
-
2[BEGINNER] Introduction to simplified hardware model used through the course.Video lesson
Dive into the heart of hardware processing and memory management in this insightful lecture, perfect for those looking to broaden their understanding of the underlying principles of C++. We begin with a comprehensive overview of the hardware perspective, breaking down how the cores in a machine's processor function. You'll learn about hyperthreading and gain clarity on logical cores.
Key Takeaways: Hardware Perspective, Cores, Processor, Hyperthreading, Logical Cores, Memory Model in C++, Cache Coherence, MSI Protocol, Cache Inconsistency.
-
3Section QuizQuiz
-
4Memory Model guaranteesVideo lesson
Dive deep into the world of C++ atomics with this comprehensive course. Master the art of dealing with race conditions and default atomics while ensuring your code executes as expected. Learn to discern between hardware and programming problems, a critical skill for every seasoned developer. This course also provides insights into the potential pitfalls of using atomics over standard mutexes or thread-safe models.
Discover why leaning towards atomics can invite complexity, leading to potential bugs impacting the production environment. Moreover, understand the scenarios where atomics are indispensable and where they are not required, thus promoting a less convoluted software engineering culture. This course teaches one to sidestep unnecessary complications and stick to the standards to avoid potential programming mishaps.
This course is not just about learning; it's about imparting a more pragmatic approach to programming in C++, which ultimately paves the way for delivering cleaner, more efficient code.
Key takeaways: C++ Atomics, Race Conditions, Default Atomics, Mutexes, Thread Safety, Hardware and Software Issues, Software Engineering Culture
-
5External factors affecting the program execution workflow of a C++ programVideo lesson
Get to grips with the intricacies of compiler behavior and code execution in this insightful lecture. Discover how your machine executes your written code, and grasp how certain 'ghost' bugs can emerge due to the lack of understanding about how the system interprets and runs your code.
Our session highlights the significant role compiler optimizations play in shaping the final assembly code and the profound impact of Out-of-Order and speculative execution techniques employed by the processor for optimal operations. Learn how the kernel scheduler and cache coherency contribute to the overall program behavior and how overlooking these aspects can lead to concurrency-related issues and runtime errors.
Ultimately, this lecture is aimed at helping you understand the potential blind spots in your programming knowledge and become more adept at handling and avoiding common yet complex problems in your code.
Key Takeaways
Compiler Optimizations
Out-of-Order Execution
Speculative Execution
Cache Coherency
Kernel Scheduler
-
6Sequential consistency definition in multithreaded applicationsVideo lesson
In this insightful Udemy course lecture, you will explore the fundamental concept of sequential consistency in programming, initially defined by Leslie Lamport in 1979. Sequential consistency signifies that the order of operations in your high-level programming language will be preserved during execution across multiple processors, which is a guarantee that the hardware needs to provide. Interestingly, sequential consistency isn't a default property of the hardware but an optional one. You will discover throughout the course how the C++ memory model can impose sequential consistency on the hardware, emphasizing that it must be communicated by the software to be executed in that specific order.
Key Takeaways:
Sequential Consistency
Leslie Lamport
High-level Programming Language
C++ Memory Model
Hardware and Software Communication
-
7Quick CheckQuiz
-
8Race condition in concurrency with regard to sequential consistencyVideo lesson
Embark on a journey to understand race conditions and memory locations in C++ programming in this comprehensive Udemy lecture. Learn how race conditions, where a memory location or variable can be concurrently accessed by two threads, with at least one being a writer, can potentially lead to corrupt data. Explore the irregular occurrence of race conditions and understand why they are considered errors that must be eliminated to prevent fatal flaws in your program. We delve deeper into memory locations, their relationship with machine word size, and their importance in considering memory protection for data structures like arrays. The lecture also elucidates operation ordering in C++ to avoid race conditions, illustrating it with practical examples.
Key Takeaways:
Race Conditions
Memory Locations
Concurrent Access
Machine Word Size
Operation Ordering in C++
-
9SC-DRF : Sequential Consistency (Data Race Free)Video lesson
In this enlightening Udemy lecture, delve into the heart of Sequential Consistency Data (SCD) Free constraints and their impact on the interaction between software and hardware in C++ programming. Gain insights into how software must inform hardware about its specific requirements, including those involving sequential consistency, to prevent hardware and compilers from blindly optimizing for performance, potentially leading to incorrect logic or race conditions. Grasp why you, as a software developer, have to take responsibility for specifying the right constraints using atomic operations and memory models in your program. The lecture also throws light on the Computer Scientists-Defined (CDF) contract between software and hardware and the importance of a standardized synchronization model across various languages, including C++ and Java, for avoiding chaos and promoting scalability in the programming world.
Key Takeaways:
Sequential Consistency
Compiler-Hardware Interaction
Memory Models
Software-Hardware Contract
Standardization in Programming Languages
-
10Role of Modern C++ memory model in guarantee of SC-DRFVideo lesson
In this comprehensive Udemy lecture, we explore the series of transformations your source code undergoes from when it is submitted to its execution on a server or target device. We will discuss the initial transformations at the compiler or JIT level, including subexpression elimination, register allocation, and software transactional memory, which are part of the compiler's optimization and program restructuring techniques.
The lecture also covers transformations at the processor and hardware level, such as instruction prefetching and speculative execution, along with the role of the cache in ordering program execution at runtime.
Understanding the role of memory models in C++ programming is crucial, and this course offers insight into the guarantees provided by the memory model and the necessity of using it correctly. By the end of the lecture, you will have a firm grasp of the order of loads and stores at the hardware level and how to use C++ syntax to ensure ordered execution.
Key Takeaways:
Code Transformations
Compiler Optimization Techniques
Processor and Hardware Level Transformations
Role and Usage of Memory Models in C++
Sequential Consistency
-
11Quick CheckQuiz
-
12Simple optimization exampleVideo lesson
Dive into the world of concurrent programming with this insightful Udemy lecture on atomic operations and thread synchronization. This course explores how seemingly trivial code can become problematic in a concurrent environment. Learn about the initialization and set of atomic flags and their role in multithreaded applications, especially when threads want to access the same critical section simultaneously. The lecture elaborates on issues arising from unordered atomic operations and suggests methods to resolve these.
Discover the recommended approach to using atomic variables and understand the importance of sequential consistency. Grasp the utility of the atomic syntax provided by C++ and the trade-offs between readability and performance when choosing between system locks and atomics. We also touch upon the riskier method of using raw memory barriers and emphasize the importance of avoiding unnecessary complexity for future code maintenance.
This lecture is designed for intermediate to advanced programmers familiar with C++ and eager to explore the intricacies of concurrent programming, atomic operations, and thread synchronization.
Key Takeaways:
Atomic operations in a concurrent environment
Thread synchronization
Issues with unordered atomic operations
Sequential consistency in multithreading
Atomic syntax in C++
Trade-offs between system locks and atomics
Risks of using raw memory barriers
Importance of avoiding manufactured complexity
-
13Code walkthrough of issue with the concurrent execution of multithreaded codeVideo lesson
Take a deep dive into the intricacies of concurrent execution in hardware in this comprehensive Udemy lecture. Learn about potential problems in a shared memory environment, such as the risk of simultaneous access to critical sections.
This lecture explores the steps taken by multiple processors accessing global memory, with an emphasis on how 'writes' to the store buffer and flushing to the global memory operate. Understand how the asynchronous nature of memory flushing can create complications in thread synchronization.
You'll also understand how 'reads' are allowed to pass the store buffer and how this can lead to both threads erroneously entering the critical section simultaneously, despite no errors in the code. This lecture will highlight the circumstantial nature of such concurrent scenarios and the need for well-planned thread synchronization.
Designed for those with basic knowledge of hardware and concurrency, this course will provide a deeper understanding of the complex dynamics of concurrent execution.
Key Takeaways:
Concurrent execution in hardware
Shared memory environment
Writing to and flushing from the store buffer
The asynchronous nature of memory operations
Risks of simultaneous access to critical sections
The circumstantial nature of concurrency issues
-
14Optimization example with single threaded codeVideo lesson
Learn about the intriguing world of compiler optimizations in single-threaded code in this comprehensive Udemy lecture. Uncover how to simplify code execution, enhance efficiency, and improve the overall performance of your programs by leveraging the compiler's optimization capabilities.
The lecture offers in-depth insights into how compilers can streamline code, such as eliminating redundant assignments and interchanging the order of operations for variables that aren't used sequentially. Understand how compilers can minimize the number of assembly instructions runs, reducing unnecessary execution and enhancing program speed.
Get introduced to techniques like using a temporary variable to minimize global memory access, which can significantly boost performance. Delve into examples showcasing how code reordering can maintain sequential consistency, providing a vital foundation for transitioning to a multithreaded environment.
However, while these optimizations work perfectly in a single-threaded context, they can lead to complications in multithreaded environments. Be prepared to avoid pitfalls while scaling your code.
Key Takeaways:
Compiler optimizations in single-threaded code
Eliminating redundant assignments
Code reordering for maintaining sequential consistency
Use of temporary variables for minimizing global memory access
Awareness of potential pitfalls in a multithreaded environment
-
15Summary and general tips around optimization of concurrent code in modern C++Video lesson
Get ready to level up your coding capabilities in our new Udemy lecture, which encapsulates the essential knowledge for optimizing code from a holistic perspective. This lecture is about learning how to identify operations on mutable or shared locations and streamline your code around these shared variables.
Dive deep into the intricacies of the compiler and understand the instances when it can autonomously optimize your code and when it might fall short due to visibility issues. Master more subjective optimization techniques required when the compiler lacks the necessary context.
Explore various tools and design decisions, and understand the memory model better to make upfront decisions, saving you from catching them later in production across different languages like C++, Java, and Golang.
The lecture underscores the importance of understanding the sequentially consistent data race-free (SCDRF) model - a cornerstone for composing your programs correctly. Learn how to write programs that fulfill the correct ordering requirements and deliver the correct promises to the hardware.
The course will further elucidate the criticality of synchronization in concurrent ecosystems using practical examples, equipping you to avoid pitfalls like Dekker's algorithm.
Key Takeaways:
A holistic approach to code optimization
Understanding operations on mutable or shared locations
Subjective optimization techniques
Tools, design decisions, and memory models
Sequentially consistent data race-free (SCDRF) model
Composing programs correctly
Importance of synchronization in concurrent ecosystems
-
16Section QuizQuiz
-
17Thinking in TransactionsVideo lesson
In this illuminating Udemy lecture, we dive into the key aspects of the C++ memory model and the transactional nature it offers to memory-related operations. Grasp the importance of maintaining invariants during data operations and understand the permissible reorderings that the memory model allows, ensuring that the desired outcome remains intact.
Discover the role of atomic constructs in the C++ Memory module, guaranteeing either complete data change or no modification at all, leaving no room for partial changes or corruption. Comprehend how the hardware implements and supports these guarantees, thus leading to a platform and hardware specificity.
Uncover how the memory model ensures data consistency and atomicity and appreciate the necessity of ordering sequential atomic operations correctly to prevent compromising data consistency. Learn to utilize memory model facilities accurately per the underlying atomic guarantees.
Explore the concept of isolation provided by the C++ memory module in handling changes during concurrent executions on the same shared data. Understand how controlling atomic guarantees allows programmers to maintain this isolation while sequencing events according to their specific logic.
Finally, draw parallels between these memory model principles and the ACID (Atomicity, Consistency, Isolation, Durability) database transactions, providing valuable insight into one of the fundamental pillars of writing databases.
Key Takeaways:
Understanding the C++ memory model and its transactional nature
Maintaining invariants in data operations
Atomic constructs and their guarantees
Implementing atomic guarantees with hardware support
Importance of data consistency and atomicity
Using memory model facilities in conjunction with atomic guarantees
Ensuring isolation in concurrent executions
The concept of ACID transactions concerning the C++ memory model
-
18The concept of critical section in concurrent and multithreaded applicationsVideo lesson
Unlock the secrets of the C++ memory model in this advanced programming lecture. With a focus on the C++ memory model's three primary mechanisms for transaction isolation, you'll gain valuable insights into ensuring the secure execution of critical regions.
This lecture introduces the concept of critical regions as transactions and presents in-depth discussions on how C++ provides transactional guarantees for your programs. It begins by examining the use of mutexes for protecting variables and showcasing the implementation of a critical section with a mutex.
The discussion then shifts to ordered atomics, where you will learn how to leverage atomic syntax for variable protection. The concept of atomic acquisition and release within a critical section is illustrated to understand this approach clearly.
Lastly, the lecture briefly introduces the concept of transactional memory, a more robust approach under consideration for future C++ standards.
This lecture is ideal for advanced programmers seeking to further their understanding of the C++ memory model, transactional isolation, and the critical importance of maintaining data integrity.
Key Takeaways:
Understanding critical regions and transactions in C++
Mechanisms for transaction isolation in the C++ memory model
Mutexes and their Role in protecting variables
Using ordered atomics for finer control
The Future of transactional memory in C++
-
19Concurrency considerations while moving code out of critical sectionsVideo lesson
This lecture forms part of an advanced C++ programming series, focusing on the critical acquire and release semantics inherent to the language. We dissect simple code examples and explore the mechanisms and implications of locking and unlocking mutexes in the C++ memory model.
The lecture explains how mutex locking initiates a critical section, setting variables, and release, or unlock, an operation that concludes it. We delve into system optimizations and potential transformation issues, demonstrating why specific sequences of operations must remain intact.
Furthermore, we highlight the compiler's role in operation reordering and the potential problems of changing these sequences, such as race conditions. By studying potential compiler missteps, you will better understand why the specific syntax is introduced into the language to ensure correct operation sequences.
Ultimately, you will understand why codes cannot be moved out of the critical section by optimization or transformation primitives. This lecture is perfect for intermediate to advanced programmers seeking a deeper understanding of C++ memory semantics.
Key Takeaways:
Understanding acquire and release semantics in C++
The role and function of mutex locking and unlocking
The importance of operation sequence in C++
The role of the compiler in operation reordering
Understanding why codes cannot be moved out of the critical section
-
20Concurrency considerations while moving code inside of critical sectionsVideo lesson
This detailed lecture provides an in-depth look into the manipulations possible within the critical sections of C++ code. Building on the previously discussed concepts of variables (X, Y, and Z), we introduce the effects of lock and unlock operations on these variables. We expand on what code can be moved into the critical section, highlighting the potential for code reordering.
You will gain insight into the conditions under which variables can be moved inside the critical section and understand how the code can be reordered. We further illustrate how certain variables' position changes don't affect the overall function of the code after the unlock operation.
However, the session also has an essential caveat: the code cannot be reordered around the critical section. This is due to the compiler's lack of knowledge regarding the lifetimes and scopes of variables outside the section. You will learn to ensure the compiler does not reorder around the critical section, which could lead to potential bugs.
Key Takeaways:
Understanding the movement of code within critical sections in C++
Understanding code reordering in critical sections
Insight into the impacts of code movement and reordering on the function of the code
Knowledge about potential compiler issues and bugs related to code reordering
-
21Quick CheckQuiz
-
22Concept of acquire and release barriers in concurrency memory modelsVideo lesson
This lecture delves deep into the fundamental concepts of acquire and release barriers in C++ concurrency, presented pictorially for easier comprehension. You will explore the release store's role in making its prior accesses visible to a thread performing an acquire load.
We further break down the acquire barrier's operation, likening it to a lock, where code can move past the barrier but not ahead of it. Similarly, we discuss the constraints around the release barrier, explaining its ability to move certain operations before it but preventing the reverse.
The necessary consistency guarantee that the release barrier provides is a key focus, as it ensures all code changes between the barriers are visible to all threads. The lecture also emphasizes the paired nature of acquire and release operations in maintaining consistency in multithreaded code.
Key Takeaways:
In-depth understanding of acquire and release barriers in C++ concurrency
Insight into the operations of the acquire barrier
Comprehension of the release barrier's role and its consistency guarantee
The concept of code reordering around acquire and release barriers
Importance of acquire-release pairs in multithreaded code
-
23Considerations while choosing memory barriers while desiging for multithreadingVideo lesson
In this lecture, we continue our journey into the realm of C++ concurrency, focusing on fences, also known as barriers, in combination with the acquire and release operations. We stress the importance of using acquire and release operations together to publish and read consistent data.
We delve into the stricter, error-prone methodology involving a straightforward barrier, a construct that does not allow any operations across it. Contrasting it with the acquire barrier, we explain how it blocks any code movement during optimization.
The lecture also discusses the indirect benefits of using barriers, especially when uncertain about the semantics of acquire and release operations. However, it is noted that full barriers can potentially hinder optimization in high-performance code, making it suboptimal.
Lastly, we shed light on the balance between starting with full barriers and later converting them to acquire and release barriers based on the situation while also cautioning about the difficulty of achieving a robust testing suite. We stress that getting these semantics correct leads to high-performance code with the lowest execution footprint, but the consequences can lead to significant issues in production.
Key Takeaways:
Understanding of fences or barriers in C++ concurrency
Knowledge of using acquire and release operations together
Comprehension of a straightforward barrier and its constraints
Insights into the circumstantial benefits and limitations of full barriers
Awareness of the balance and challenges in transitioning from full barriers to acquire-release barriers
-
24A closer look at barriers and their relation to sequential consistencyVideo lesson
In this lecture, we further explore C++ concurrency by examining the order of operations around fences or barriers, focusing specifically on acquire-release barriers. We compare two models: the plain acquire-release model, where hardware does not comply with any order, and the sequentially consistent model, where the programmer explicitly sets the expected ordering.
In both models, the acquire barrier cannot surpass a release barrier - they can converge at most but cannot cross each other. The behavior in both models is very similar, and the code cannot cross an acquire-release barrier for a specific variable.
However, things change when an acquire barrier follows a release barrier. The two barriers can pass each other in a plain acquire-release scenario without any provided ordering, leading to potential problems. In a sequentially consistent acquire-release model, the two barriers cannot cross each other, thus maintaining an important guarantee of sequential consistency.
The lecture concludes by emphasizing the predictability of the memory model in concurrent programming due to sequential consistency. The sequentially consistent model ensures that the release of a certain set of instructions will never be superseded by acquiring the next set of instructions, thus preventing a mix-up in order.
Key Takeaways:
Understanding of barriers in two models: plain acquire-release and sequentially consistent acquire-release
Insights into the behavior of acquire and release barriers in different situations
Knowledge about the implications of having a release barrier followed by an acquire barrier in both models
Understanding of the guarantee of sequential consistency in the memory model of concurrent programming
Awareness of the importance of predictability and order maintenance in concurrent programming
-
25Summary of memory barriers in concurrent applicationsVideo lesson
This insightful lecture deepens our understanding of the C++ memory model, focusing on barriers or fences that facilitate memory synchronization. The lecture underlines the delicate relationship between memory synchronization, which aligns with the programmer's intent, and hardware optimizations. While these two aspects might seem to be working against each other, they are merely trying to establish certain constraints to balance performance and correctness.
The lecture also emphasizes the potential risks of switching from locks to atomics for performance gains without considering the role of cache coherence. While hardware is designed for high performance and throughput, incorrect use of programming constructs can unintentionally slow it down. The instructor advises learners to avoid unnecessary changes unless they can measure and guarantee performance improvement.
Later, the lecture introduces the concurrent data structure design concept and highlights the differences between wait-free, lock-free, and obstruction-free programming. The discussion underscores the importance of keeping these nuanced aspects separate from the code used by multiple developers due to their steep learning curve and runtime error detection.
Further, the lecture explains how hardware tries to keep memory pipelines full to reduce costs and ensure efficiency. It does so through branch predictions, speculative execution, and prefetching of instructions. The lecture concludes with a simple yet effective formula: "Concurrency is bandwidth into latency," reminding learners that reducing pipeline latency and having multiple pipelines will ensure efficient execution.
Key Takeaways:
Understanding of barriers and fences in the C++ memory model
Insights into the interplay between memory synchronization and hardware optimizations
Introduction to wait-free, lock-free, and obstruction-free programming
Understanding of hardware performance optimizations such as branch predictions, speculative execution, and prefetching of instructions
Introduction to the formula for concurrency: "Concurrency is bandwidth into latency."
-
26Impact of external optimizations on concurreny in modern C++ applicationsVideo lesson
This Udemy lecture dives deep into the optimization strategies to maximize computing power and improve code performance. It elaborates on the intricate relationships between bandwidth, latency, and concurrency, providing valuable insights into these factors' significant role in code execution.
The lecture begins with a discussion on parallelization, stressing the importance of leveraging the available compute power and hardware threads. It further highlights techniques for parallelization, such as pipeline execution and multithreading. The concept of executing multiple instructions concurrently to keep the pipeline busy and improve code efficiency is introduced, emphasizing the importance of scheduling memory-intensive operations first.
The lecture then transitions into caching strategies and how they can enhance the capacity usage of each core. It explains various levels of data caches and their roles in ensuring lightweight instructions instead of heavyweight memory access instructions. The lecture also highlights the importance of understanding and optimally utilizing cache lines for writing high-performance code.
The next strategy discussed is speculative execution, which combines bandwidth and computing power to improve the efficiency of code execution. Topics such as branch prediction and optimistic execution are detailed, with an acknowledgment of how hardware manufacturers' analysis impacts these strategies.
Key takeaways include understanding the role of parallelization, caching, and speculative execution in optimizing code performance. Awareness of pipeline execution, instruction caches, data caches, and hardware threads is also emphasized.
-
27Quick checkQuiz
-
28Considerations while making performace measurementsVideo lesson
This informative lecture dispels some common myths about the use of atomics and locks in programming. We stress the importance of measuring performance before making assumptions and that these measurements should not be generalized as they are highly specific to the hardware and compiler versions. The lecture dives into the discrepancies that may arise between local and cloud-based systems due to variances in vCPUs. By comparing atomics to other threadsafe alternatives like locks, we aim to understand their performance and validity better. We highlight that although such comparisons are useful in their context, they cannot be directly applied to production due to many possible variations. The crux of this lecture is to help learners understand that performance improvements will not miraculously occur by changing atomic operations and to encourage them to carry out their experiments in code composition.
Key Takeaways:
Importance of measuring performance before making assumptions.
Hardware and compiler specificity in performance measurements.
Discrepancies between local and cloud-based systems.
The comparison of atomics and threadsafe alternatives like locks.
The necessity of experimenting with code composition.
-
29Code experiment recommended to be performed by students.Video lesson
In this practical lecture, we guide students through an engaging experiment that compares runtimes between different thread access scenarios. The experiment examines the performance difference when multiple threads increment the same variable versus when they work on individual elements within an array. While theory suggests that threads accessing individual elements should result in faster execution, this lecture encourages learners to validate this claim through direct experimentation. The hands-on nature of this exercise underscores the need to question assumptions and verify theory through practical application.
Key Takeaways:
Comparison of runtimes in different thread scenarios.
Importance of validating theoretical claims through hands-on experimentation.
Understanding of thread performance when accessing shared versus individual variables.
-
30Code demonstartion of behavior of atomic variables in practiceVideo lesson
This insightful lecture delves into the complex topic of byte alignment and how it can affect the speed of atomic operations in coding. The lecture introduces a code that utilizes the basic atomic header and the IOSTREAM header, illustrating five different structures with varying elements and sizes. The discussion further focuses on cache alignment and lock-free programming, highlighting their significance and potential misconceptions surrounding them. The lecture uses an engaging approach to guide learners through writing and running the code, drawing attention to the potential discrepancies in outcomes across different systems.
Key Takeaways:
Understanding the impact of byte alignment on the speed of atomic operations.
Hands-on coding experience with different data structures.
Deep dive into the concept of cache alignment and its significance.
Insight into lock-free programming and its potential misconceptions.
Introduction to static functions with atomics, highlighting variations across different platforms.
-
31Do atomic variables wait for each other?Video lesson
Explore the intricacies of atomic operations in this detailed Udemy lecture, focusing on performance considerations and common misconceptions. The course begins by debunking the widespread myth that atomic operations are instant, highlighting their dependence on hardware and specific limitations. It emphasizes the need for good algorithms as the primary aspect of achieving high performance. The course then delves into the nature of 'wait-free' systems, discussing how the concurrent execution of smaller operations alongside larger ones can increase overall throughput. Real-world examples, such as Dekker's Algorithm, illustrate how atomic operations might need to wait for each other, especially in write operations. Lastly, the lecture explores how atomic operations could slow down when data is laid out contiguously.
Key Takeaways:
The misconception about the instantaneity of atomic operations.
The role of solid algorithms in performance optimization.
The concept of 'wait-free' systems in concurrent operations.
The potential bottlenecks in atomic operations, such as in write operations.
The impact of data layout on the performance of atomic operations.
-
32False sharing in concurrency and multithreadingVideo lesson
In this advanced Udemy lecture, the focus shifts to the subtleties of atomic operations and the impact on cache line access in high-performance code. The course emphasizes the importance of these factors during code-writing and notes that compilers can't detect these complexities but can be addressed through language syntax. It introduces the 'MESI cache protocol,' underlining its cost on shared data to prevent race conditions. The course elaborates on the problem of 'false sharing,' where two memory locations on the same cache line can cause delays for each other. A solution proposed is data alignment to separate cache lines, particularly on Non-Uniform Memory Access (NUMA) machines. Contradictions with competitive programming hacks that aim to pack as much data as possible into a single memory location are also discussed. The lecture ends with the highlight that while data alignment can lead to wastage, one has to understand the trade-off and decide accordingly, implying there's no one-size-fits-all solution.
Key Takeaways:
Importance of atomic operations in writing high-performance code.
The impact of 'false sharing' on runtime performance.
Techniques to avoid 'false sharing,' like data alignment to separate cache lines.
The trade-off between data packing and cache coherence in a multi-core environment.
Consideration of potential wastage and trade-offs when aligning data per separate cache line.
-
33Section QuizQuiz
-
34Introduction to compare and swapVideo lesson
In this advanced Udemy lecture, the instructor discusses the vital role of the Compare-and-Swap (CAS) operation in most lock-free algorithms. Using C++ atomic primitives, the lecture specifically delves into the 'compare_exchange_strong' function. This function enforces sequential consistency, a higher restriction than weaker sequential consistency models. The function compares an expected value with the current value of an atomic variable. The atomic variable is swapped with a new provided value if the comparison holds. This operation ensures that the variable hasn't changed and that expectations are met. If the comparison fails, indicating that another operation has changed the value, the function returns the current value and a false boolean indicator. This functionality can act as a lock and a condition, effectively acting as a synchronization barrier in lock-free programming. A simple example illustrates these principles.
Key Takeaways:
The crucial role of the Compare-and-Swap operation in lock-free algorithms.
Functioning of 'compare_exchange_strong' in C++ atomic primitives.
Use of this operation as a lock, condition, and synchronization barrier.
Handling of true and false outcomes of the 'compare_exchange_strong' operation.
-
35Example of compare and swapVideo lesson
This lecture continues the previous discussion about the Compare-and-Swap (CAS) operation in lock-free programming. It highlights how CAS can be found in most lock-free algorithms, including those provided by the library or third-party library implementations. The lecture emphasizes that CAS is not enough to achieve lock-free programming; its optimal use in the right places is necessary for successful implementation. Misusing CAS under the assumption of achieving lock-free programming can lead to significant mistakes.
The lecture then covers atomic increments in languages for integer data types, explaining that the operators will behave atomically when using an atomic INT. For operations not supported by library syntax out-of-the-box, such as increments of doubles or multiplication of integers, CAS can be utilized.
The instructor provides a code example demonstrating the use of CAS with an atomic integer variable, suggesting the possibility of trying other data types, such as long double. The example emphasizes the necessity of checking the outcome when using the compare-and-exchange function.
Key Takeaways:
The extensive use of Compare-and-Swap in lock-free algorithms.
The importance of optimal CAS usage in the correct contexts for successful lock-free programming.
The atomic behavior of operators in languages for integer data types.
The role of CAS in operations unsupported by library syntax, such as increments of doubles or multiplication of integers.
The necessity of outcome verification when using the compare-and-exchange function.
-
36Pseudo code implementation of compare and exchange strongVideo lesson
This lecture explores the causes of spurious reads in the Compare-and-Swap (CAS) operation. The discussion is carried out via pseudocode to help explain the concept. The lecture begins with explaining strong implementation before transitioning to weak implementation. The return type of the CAS operation is revealed to be boolean, and it accepts a reference of type T old value and the new value, passed by value.
The lecturer explains that the first step in the operation is to acquire a lock for exclusive access. However, mentioning the lock here is notional and doesn't denote a specific syntactical entity. Once this lock is acquired, the current value can be read and compared with the old value provided by the user.
The operation then checks if the value in the memory is the same as the one provided by the programmer. If it isn't, the function updates the 'old value' variable with the current memory value and returns false. If it is the same, the function updates the state with the new value provided by the programmer and returns true.
Finally, the lecture covers the subject of an exclusive access mechanism, saying it doesn't have to be a real mutex but rather something implemented by the hardware being compiled. The efficiency of this mechanism cannot be improved beyond what the platform provides. Thus, developers must rely on the hardware.
Key Takeaways:
Understanding the causes of spurious reads in Compare-and-Swap operation.
The process of acquiring a notional lock for exclusive access to the operation.
The CAS operation's steps are based on the values in memory and those provided by the programmer.
The role of exclusive access mechanisms and hardware reliance in CAS operation.
-
37Pseudo code implementation of compare exchange strong - fasterVideo lesson
This lecture presents an optimized version of the Compare-and-Swap (CAS) operation in the strong case, leveraging that atomic reads are faster than writes. The optimization process primarily shifts from acquiring a lock as the first operation to reading the value first. If the value does not match the programmer's expectations, the function can return false immediately, reducing the need for unnecessary operations.
Furthermore, the lecture explains that this operation can occur without locking the value due to the atomic nature of the operations, ensuring no corrupt data is read. The lock moves to a more optimal position but introduces the possibility that the value might have changed after acquiring the lock, necessitating a double check under the lock.
The lecture acknowledges a slight overhead and a trade-off in this approach but emphasizes that a fast read upfront allows quick failure, resulting in overall efficiency. Finally, the lecturer references a dedicated course for concurrent data structure design for students interested in exploring these concepts further.
Key Takeaways:
The optimization of Compare-and-Swap operation to leverage faster atomic reads.
An understanding of how shifting the lock position can influence the operation.
A double-check under the lock is necessary due to potential value changes.
Acknowledgment of the trade-off and overhead in the optimized Compare-and-Swap operation.
Exploration of concurrent data structure design in a dedicated course.
-
38Compare and exchange weak - reason for spurios failuresVideo lesson
This lecture discusses the Compare-and-Exchange-Weak (CAS-Weak) operation, which aims to make the processing faster and allows shared mode operations if exclusive access is hard to acquire. Unlike Compare-and-Exchange-Strong (CAS-Strong), which seeks exclusivity, CAS-Weak permits other processes to proceed if they cannot.
The lecture elaborates on how CAS-Weak might be implemented, highlighting that the lock-free comparison remains the same, allowing the value to be read and returning false if it does not meet expectations. In this cooperative mode, time locking can be used. It suggests a try-once approach instead of waiting for exclusive access, speeding up the process.
However, it raises a tricky point: although the value can be changed after the first read part, it returns false if a lock can't be acquired. This 'spurious' change can lead to confusion and ambiguity. It's emphasized that the value may have changed between the initial read and lock acquisition, so a re-comparison is necessary. If the value is the expected value, the state can be changed, and a true value is returned.
Key Takeaways:
The functioning of Compare-and-Exchange-Weak (CAS-Weak) operation.
The benefits of a cooperative mode using time locking.
Understanding of 'spurious' change and resulting ambiguity.
The necessity of value re-comparison in CAS-Weak.
State changes and return values in CAS-Weak.
-
39Section QuizQuiz
-
40Memory ordering basics in concurrencyVideo lesson
This lecture delves into the vital role of synchronization orderings in the memory model of C++ atomics. It explains that the mere presence of atomic operations is insufficient; efficient and correct operation also requires a specific ordering. The lecturer discusses the benefits of sequentially consistent and weak models and how the latter can improve performance by reducing locking and assembly generation.
The lecture emphasizes that the primary use of atomics is to access memory exclusively or to perform an operation and reveal the final state to other threads, acting as a gatekeeper. It reminds us that memory is not atomic and can be accessed by multiple threads, highlighting that the programmer must request atomicity from the hardware.
The lecture underscores the importance of asking, "What guarantees that other threads see this memory in the desired state?" during programming. The lecturer also emphasizes the role of atomicity in acquiring and releasing exclusive access, noting that everything should be updated before access is provided, and all updates should be completed before data is published to the rest of the system for consumption.
Key Takeaways:
The role of synchronization orderings in the memory model of C++ atomics.
The importance of understanding the primary use of atomics.
The programmer must request the atomic nature of memory.
The importance of memory state visibility to other threads.
The necessity of complete updates before access and release in atomic operations.
-
41Memory ordering nuancesVideo lesson
This lecture focuses on the importance of memory access order for achieving synchronized data access in multi-core environments. It highlights that synchronization is impossible without a predictable order of data access, potentially leading to chaotic order. It emphasizes that atomic operations, which guarantee the validity of state change, and the order of memory access are equally important for synchronized data access.
The lecture introduces barriers built into the language that provides global control across all CPUs. The lecturer underscores the scalability of well-written concurrent code for a multi-core environment, ensuring performance irrespective of the number of cores. The role of hardware-implemented memory barriers in inserting certain barriers in code and potential ways to avoid them are discussed.
The lecturer explains that memory barriers can be either OEM-provided or constructed with a set of instructions, depending on the platform. The lecture concludes by viewing barriers as attributes on read and write operations, stressing that they provide the order in which these operations should happen.
Key Takeaways:
The importance of memory access order for synchronized data access.
The role of atomic operations in guaranteeing the validity of state change.
The function of language-embedded barriers in multi-core environments.
The significance of hardware-implemented memory barriers.
The understanding of barriers as attributes of read and write operations.
-
42Memory ordering and memory barriers in modern C++ languageVideo lesson
This lecture explores the implementation of the memory model according to the C++ standard, emphasizing the transition from C++03 to C++11. It discusses the absence of portable memory barriers in C++03, leading to platform-specific implementations, and contrasts it with C++11, which introduces standard memory barriers for portability across different platforms.
The instructor elaborates on the close relationship between memory barriers and memory order, underscoring that memory barriers facilitate memory order. They detail how C++ memory barriers function as modifiers on atomic operations, allowing for varying implementations depending on the compiler and platform but with consistent results due to standard compliance.
The lecture concludes with a practical example of using memory order to store a value in an atomic integer, showcasing the application of a release barrier to achieving the desired memory order.
Key Takeaways:
The transition from C++03's lack of portable memory barriers to C++11's standard memory barriers.
Importance of memory barriers in enabling memory order.
Use of C++ memory barriers as modifiers on atomic operations.
Compiler and platform-dependent implementations of memory barriers.
A practical example of using memory order in C++.
-
43Acquire Barrier in Modern C++Video lesson
The lecture dives deeper into using memory order in C++, highlighting different syntax usages and their effects on data management and control flow within a program. The session starts with explaining the memory_order_relaxed syntax, which indicates a lack of memory barriers and allows for operation reordering, a feature that can lead to unexpected results if not used judiciously.
The focus then shifts to the acquire barrier syntax, with the instructor explicitly describing its functionality within the code. They illustrate how an acquire barrier ensures that all operations scheduled post-barrier become visible only after the barrier, thereby maintaining the prescribed order of operations. Importantly, this restriction applies to all operations, not just reads or writes, and influences the control flow of the entire program, not merely the variable on which it is implemented.
The instructor stresses that these barriers are thread-specific, operating within the thread that issues the barrier and not affecting other threads working with the same memory.
Key Takeaways:
Interpretation and implications of memory_order_relaxed and acquire barrier syntax in C++.
The role of acquiring barriers in preserving the order of operations within a program.
The impact of memory barriers on both reads writes, and overall control flow.
Thread-specific application of memory barriers.
-
44Mid Section QuizQuiz
-
45Release Barrier in Modern C++Video lesson
The lecture dives into the specifics of the release barrier in C++ programming, explaining its syntax, usage, and impact on the execution flow within a thread. The instructor discusses the core principle of a release barrier: it guarantees that all memory operations are scheduled before the barrier becomes visible before the barrier itself. This ensures that all modifications made before this point are published before the program can proceed.
The lecturer then contrasts the functionality of the release barrier with that of the acquire barrier. While the acquire barrier allows operations before it to be reordered after it, the release barrier forbids this, instead permitting operations after it to be moved before it. These actions are confined to the issuing thread, demonstrating that memory barriers in C++ are thread-specific.
The discussion concludes by emphasizing the need to carefully implement barriers in multi-thread programming to synchronize and efficiently use memory barriers.
Key Takeaways:
Understanding the release barrier syntax and its effect on program execution.
The release barrier guarantees that operations are scheduled before the barrier becomes visible before the barrier itself.
The contrast between acquire and release barriers in terms of operation reordering.
The thread-specific application of memory barriers in C++.
The importance of synchronization in multi-thread programming.
-
46Using acquire and release barriers for synchronization in multithreadingVideo lesson
This lecture extends the previous discussion on acquire and release barriers, exploring how they interact and synchronize within a multi-threaded program in C++. The instructor illuminates the integral concept of memory order and how it's achieved using acquire and release barriers across different concurrent functions. The lecture emphasizes that this is not confined to a single thread but pertains to the holistic composition of programs, allowing synchronization of concurrent functions using these barriers.
A key highlight of the discussion involves an atomic variable 'X', written by Thread 1 with a release barrier and read by Thread 2 with an acquire barrier. The instructor explains how these barriers ensure consistent visibility of memory operations across threads, thus facilitating the synchronization of concurrent processes.
Furthermore, the lecture emphasizes the role of variable 'X' as more than just a placeholder for data but as a signaling mechanism indicating the completion of memory operations in a thread. It establishes how to acquire and release barriers to ensure consistent data visibility in multi-threaded programming.
Key Takeaways:
Understanding of memory order in concurrent programming.
Acquire and release barriers' joint role in synchronizing across different concurrent functions.
Using an atomic variable 'X' as a signaling mechanism informs of completed memory operations.
The principle of visibility and consistency is ensured by acquire and release barriers across different threads.
The concept of 'X' as a medium of communication to establish data consistency across threads.
-
47Using memory barriers as locks for efficient concurreny with modern C++Video lesson
This lecture presents a straightforward guide for transitioning from locks to atomics in C++ programming, emphasizing how to enhance performance through this change. The speaker starts by presenting the equivalency of a lock to an atomic variable, using an integer as a practical example, noting that other user-defined and plain old data types can also be utilized.
The main part of the discussion explains the transformation of the lock mechanism into the use of a store with memory order acquire in atomic operations. This ensures an acquire barrier is set, indicating the beginning of a critical section in the code.
In this critical section, operations like incrementing a variable are performed with the understanding that these operations could be scaled as needed. Completing the critical section is signaled by the memory order release, effectively "unlocking" the operations and informing other threads that operations can proceed as normal.
The value of the atomic variable acts as a gatekeeper, signifying when operations can and cannot proceed, ensuring effective and safe multi-threaded operations.
Key Takeaways:
Understanding of how to transition from locks to atomics in C++ programming.
Explanation of using store with memory order acquire and release in atomic operations.
The role of an atomic variable as a gatekeeper in multithreaded operations.
-
48Bidirectional barriers in Modern C++ memory modelVideo lesson
In this session, the focus shifts from unidirectional barriers, such as acquire and release, to bidirectional barriers, which provide stricter control over the execution flow. Two bidirectional barriers are discussed: memory order acquire-release and sequential consistency.
Memory order acquire-release, the first bidirectional barrier discussed, combines acquire and release barriers, creating a 'hard stop' in the code and forbidding operations from moving across this point. This barrier provides clarity and ensures precise control over optimizations, but it comes with a significant restriction: it must be used on the same atomic variable across all threads.
The other barrier, sequential consistency, doesn't require using a single variable, instead establishing a single modification order for all atomic variables. This barrier makes code execution stricter and more predictable, but it could come with a performance cost due to limited room for optimization. Thus, the choice of the barrier should be made carefully, depending on specific programming requirements.
Key Takeaways:
Understanding of bidirectional barriers in multithreaded programming.
Knowledge of the two types of bidirectional barriers: memory order acquire-release and sequential consistency.
Insight into the trade-offs between strict control and optimization opportunities when using different memory barriers.
-
49Why does compare and exchange in C++ have two parameters for memory ordering?Video lesson
In this lecture, the speaker discusses the default memory order when none is explicitly specified in a multi-threaded programming context. By default, the strongest memory order, sequential consistency, is applied. This order is chosen as the default to prevent the likelihood of bugs that could be introduced if a weaker order were applied.
To extract performance benefits, developers must explicitly specify a different memory order. This should be done only after establishing correct logic and test cases under the default sequential consistency. Only then should developers consider weaker barriers to improve performance. However, the speaker stresses that these modifications should be made carefully, and performance should be constantly measured.
The speaker also highlights that this default order is applied to overloaded operators and provides an example of specifying a weaker memory order using atomic operations. However, the speaker clarifies that it is not possible to change the memory order for overloaded operators, as they will always operate in a sequentially consistent manner.
The lecture ends with the promise to look at practical examples in the upcoming sessions, emphasizing the importance of carefully choosing the right atomic orders and barriers for the intended workflow.
Key Takeaways:
Sequential consistency is the default memory order when none is specified.
The choice of memory order plays a significant role in the performance and the likelihood of introducing bugs.
Careful consideration and constant measuring are crucial when modifying the memory to achieve better performance.
Overloaded operators always operate in a sequentially consistent manner, and their memory order can't be changed.
-
50Section QuizQuiz
-
51Purpose of memory order in modern C++ concurrency memory modelVideo lesson
This lecture discusses the topic of memory order manipulation in the context of multi-threaded programming. The speaker ponders on the question of why there's a need to change the memory order, despite the risks associated with it. He explains that the main motive is to extract maximum performance from the machine. Atomics and its associated barriers facilitate efficient communication with the machine, aiding in effectively utilizing its pipelines, parallelization, caching, and other optimization techniques.
In addition, the speaker emphasizes that explicitly specifying a memory order also communicates the programmer's intent about how the program should be executed. It acts as a form of instruction for the machine and a means of communication with other programmers. This explicit communication can be especially useful in lock-free programming paradigms where high-level communication like mutex often fails to deliver optimal performance.
Throughout the lecture, the speaker urges caution and clear communication when working with memory orders to achieve the best possible performance on a platform without introducing potential bugs or problems.
Key Takeaways:
Changing the memory order can extract maximum performance from the machine.
Atomics and barriers facilitate communication with the machine.
Explicit memory orders express a programmer's intent about program execution.
Memory orders play a crucial role in lock-free programming paradigms.
-
52Memory order as a tool to convey the C++ programmer's intentVideo lesson
This lecture explores the intricacies of changing memory order within multi-threaded programming. The speaker acknowledges the complexity and potential pitfalls of altering from a strong to a weak order, with potential run-time issues particularly problematic. However, they go on to explain that the change is worthwhile, as it enables programmers to maximize the performance of a machine.
The lecture emphasizes the role of atomics and barriers in facilitating dialogue with the machine. Such dialogue aims to optimize the machine's operations, such as pipeline usage, parallelization, caching, and other optimizations. The idea is to negotiate a middle ground for achieving the best possible performance on a given platform.
Additionally, the speaker highlights the secondary purpose of explicitly using a memory order: to communicate the programmer's intent regarding the program execution to fellow programmers. This becomes particularly important in lock-free programming paradigms, where high-level communication tools like mutex often prove insufficient for optimal performance and flexibility.
Key Takeaways:
Changing the memory order can boost machine performance.
Atomics and barriers aid in communicating with the machine.
Explicit memory orders communicate programmer intent.
Memory orders are crucial in lock-free programming paradigms.
-
53Memory order as programmer's intent : Example - 1Video lesson
This lecture focuses on understanding and applying memory orders in a concurrent programming context, utilizing a specific code example to delve into the subject. The speaker introduces an atomic variable named "count," incremented using the fetch add instruction with a memory order set to relaxed. The memory order setting signifies that no other memory access depends on this variable.
The lecture further emphasizes the concept of memory orders in concurrent programming, particularly the "relaxed" order, which specifies minimal synchronization. The given example indicates that the variable "count" isn't used for indexing or as a reference count anywhere else. This leads to the understanding that the 'relaxed' memory order enables the optimization of operations without jeopardizing the correct execution of the code.
However, the speaker acknowledges the role of platform-specific behaviors and their potential to override the specified memory order. An instance of this is the execution of the fetch add instruction in an x86 platform, which applies for an acquire-release order instead of the specified relaxed order, leading to potential miscommunications.
Key Takeaways:
Atomic variables and memory order manipulation in concurrent programming.
The "relaxed" memory order allows for certain optimizations.
Platform-specific behaviors can override specified memory orders.
The importance of understanding your compiler and platform at an assembly level.
-
54Memory order as programmer's intent : Example - 2Video lesson
This lecture focuses on understanding the practical implications of using the 'release' memory order in concurrent programming. Starting from the same atomic variable code example discussed earlier, the memory order is changed from 'relaxed' to 'release' to demonstrate the significant impact this single alteration can bring.
The speaker explains that in a context where a particular thread uses a 'count' index to prepare some memory and then release it to other threads, memory order can make a notable difference. When initializing the data, the 'relaxed' memory order can be used as the 'count' incrementation isn't visible to anyone.
The scenario changes once the initialization is complete and the data is ready to be shared with other threads. At this point, incrementing the 'count' variable using the 'release' memory order signals the system that the data array is ready to be accessed, effectively communicating and synchronizing data.
Key Takeaways:
The application and impact of 'release' memory order in concurrent programming.
Memory order changes the communication and synchronization mechanism in multithreaded systems.
The choice of memory order can be context-dependent: 'relaxed' for initialization and 'release' for signaling data readiness.
-
55Memory order as programmer's intent : Example - 3Video lesson
The speaker introduces another example of atomic variable usage in concurrent programming in this lecture. This time, a simple incrementation operation on an atomic variable named 'count' is presented. The presenter explains that while the given code could be seen as one of many possible atomic operations interacting with some memory and being managed by some mechanism, the actual intent behind the code is unclear from the provided context.
The lecturer humorously suggests a different interpretation: a developer might be using atomic operations because they appear to work and are 'cooler,' even though a traditional lock mechanism would function just as well. The lecturer warns against this approach, underlining that using atomic operations without a clear understanding of their purpose or implications can lead to a 'slippery slope.' The essence of the lecture is the caution against the indiscriminate use of atomic variables and the necessity of understanding the why and how of atomic operations.
Key Takeaways:
Atomic variables in concurrent programming and their interaction with memory.
The risk associated with using atomic operations without a clear understanding of their functionality.
The importance of understanding the rationale behind choosing atomic operations over other mechanisms like locks.
-
56Mid Section QuizQuiz
-
57Memory barriers and performance implicationsVideo lesson
This lecture explores the importance of memory ordering and the performance implications in concurrent programming. The speaker discusses how memory barriers, which ensure correct memory ordering, can sometimes be more resource-intensive than the atomic operations themselves.
The lecture further emphasizes the platform-specific nature of barrier implementation, recommending that performance measurements should only be conducted on the targeted platforms to avoid misleading results. It provides specific insights on the x86 architecture, stating that loads are generally "acquire loads," stores are "release stores," and read-modify-write operations are "acquire release." However, this can change over time or on different platforms.
The lecture concludes with a discussion on the cost of adding acquire on write and release barrier on read operations. The speaker advises avoiding these expensive operations where possible and adjusting code accordingly. The potential for weaker dependencies and barriers to translating into stricter requirements is also addressed, underscoring the need to manage expectations accordingly.
Key Takeaways:
Understanding of memory ordering and its performance implications in concurrent programming.
The platform-specific nature of barrier implementation.
Awareness of the costs associated with specific operations, like adding acquire on write and release barrier on read.
Knowledge about the potential for upcasting of dependencies and barriers in certain platforms.
-
58Sequential consistency and performance implicationsVideo lesson
This lecture explores the implications of using platform-specific memory barriers in concurrent programming, focusing on performance, portability, complexity, and error-proneness. It begins with an acknowledgment that while compilers may permit platform-specific barriers like "influence" on Linux or "exchange" on Windows, their use's cost and potential complications warrant careful consideration.
One significant concern the lecture brings up is the non-portability of platform-specific barriers, pointing out that such code would not only vary across operating systems but also be affected by different processor instruction sets. The complexity and high possibility of errors associated with these barriers' to correct and consistent writing are also discussed.
From a performance perspective, the lecture argues against using standalone barriers that are often too heavy and restrict optimization. Furthermore, the instructor advises against using barriers that limit reordering at specific levels, as reordering can occur at multiple levels in the code execution flow, leading to optimized performance.
Key Takeaways:
Understanding the implications of using platform-specific memory barriers.
Awareness of the non-portability and complexity associated with platform-specific barriers.
The potential of platform-specific barriers to lead to errors and performance restrictions.
Insight into the impact of memory reordering on performance optimization.
-
59Design and implementation guidelines for using std::atomicsVideo lesson
This lecture focuses on best practices for using atomic variables and operations in C++. The instructor emphasizes the importance of utilizing the default operations and operators provided by the language rather than trying to reinvent the wheel for a small potential boost in performance. This approach is advocated due to the unnecessary software complexity and potential for bugs that could arise from trying to bypass the built-in features.
In addition to this, the lecture also delves into the topic of memory barriers and their essential role in thread interactions through memory. Memory ordering and barriers, the lecture explains, can have a significant impact on performance. As such, when opting to use atomics, one also indirectly chooses to deal with memory ordering and barriers. The lecturer urges listeners to weigh this trade-off carefully.
Finally, the lecturer likens language facilities to running with scissors, warning that it should be done judiciously. Using these tools correctly falls on the programmer, not the language.
Key Takeaways:
Importance of using default atomic variables and operations in C++.
Awareness of the potential complications and bugs that could arise from bypassing built-in features.
Understanding the role of memory barriers in thread interactions and their impact on performance.
Emphasis on the responsible use of language facilities.
-
60When to use the atomics provided by the modern C++?Video lesson
This lecture explores the appropriate scenarios for implementing std::atomics in C++, specifically developing high-performance, concurrent, lock-free data structures. The instructor underlines the need to measure and prove the performance gains from using atomics, emphasizing the importance of judicious usage due to potential factors that could lower performance.
The lecture discusses how atomics can be particularly beneficial for data structures that are challenging to implement with locks. Examples include lists and trees, where memory ownership and updates need to be carefully managed.
Furthermore, using atomics becomes crucial when applications can't afford the drawbacks of locks, such as latency issues or deadlocks. These situations could include mission-critical software development where atomics can justify their complexity if implemented correctly. The lecturer also touches upon concurrent synchronization, achievable with the simplest atomic operations, load, and store.
Lastly, the discussion delves into the scenario where the system must not "invent" a write to a variable outside your sequentially consistent execution. The instructor warns that this could make it impossible for the programmer to know which locks to take, making it essential to control the order of execution using atomics for fine-grained control.
Key Takeaways:
Std::atomics can be beneficial for high-performance, concurrent, lock-free data structures.
It's necessary to measure and prove the performance gains when using atomics.
Atomics can help manage complex data structures like lists and trees.
The usage of atomics is crucial when lock drawbacks cannot be tolerated.
Fine-grained control of sequentially consistent execution can be achieved using atomics.
-
61Section QuizQuiz
-
62Concepts of Wait free programming in modern C++Video lesson
This lecture delves into the concept of lock freedom in concurrent programming, focusing on the most robust form known as wait-free freedom. The core principle of wait-free freedom is the elimination of waiting, ensuring that all threads in a system operate independently without delay. This approach promises an unprecedented level of parallelism and high system throughput. The lecture also discusses the practical constraints and idealistic assumptions of this approach, particularly in the context of B-tree algorithms. These algorithms are designed to complete a bounded number of steps regardless of other system activities, aiming for system-wide throughput and freedom from starvation. However, achieving wait-free status in programming requires careful planning and significant effort, balancing the ideal goals with practical limitations.
Key Takeaways:
Lock Freedom in Concurrent Programming
Wait-Free Freedom
Parallelism and Throughput in Programming
B-tree Algorithm Constraints
Planning and Effort in Achieving Wait Freedom
-
63Understanding Lock-Free Algorithms: Balancing Progress and ThroughputVideo lesson
This lecture delves into the concept of lock-free algorithms, a pivotal aspect of concurrent programming. It starts by defining what lock-free algorithms are and distinguishes them from the more liberal wait-free algorithms. The lecture emphasizes the primary objective of lock-free algorithms: ensuring that at least one thread makes progress in a multi-threaded environment. This approach seeks a balance, offering a middle ground between total freedom and strict control.
The discussion progresses to the notion of 'progress' in the context of lock-free algorithms. It is highlighted that this concept is relative, varying with the specific program under consideration. The lecture underscores the importance of continuous systemwide progress to avoid complete system lockdown, which is deemed a suboptimal design in lock-free contexts.
Moreover, the lecture acknowledges the inherent compromises of lock-free algorithms. While they guarantee systemwide throughput by ensuring constant progress, their throughput is generally lower compared to wait-free algorithms. This trade-off is crucial in understanding the practical applications and limitations of lock-free algorithms in various programming scenarios.
Key Takeaways:
Lock-Free Algorithms
Systemwide Throughput
Progress in Multi-Threaded Environments
Trade-offs in Algorithm Design
-
64Mastering Obstruction-Free Programming: A Guide to Lock-Free AlgorithmsVideo lesson
This lecture provides an in-depth exploration of obstruction-free programming, a nuanced approach within lock-free algorithms. We start by defining obstruction-free algorithms as the most conservative and weakest form of lock freedom, emphasizing their unique method of ensuring progress without interference. The central strategy involves executing code in a single, isolated thread while temporarily suspending all others that might cause obstruction, thus allowing a predetermined number of steps to be completed unimpeded.
The course then delves into the practical implications of this approach, such as how it prevents system failure due to the delay or failure of a single thread. However, it also acknowledges the possibility of 'live blocks', situations where threads give precedence to each other, leading to short-lived but potential delays in execution.
The lecture clarifies the distinction between lock-free and obstruction-free programming, underlining that while all lock-free algorithms are obstruction-free, the reverse is not true. This distinction is crucial for developers in accurately categorizing their code. The session ends by emphasizing the need for programmers to identify and resolve live locks, ensuring smooth and efficient execution.
Key Takeaways:
Obstruction-Free Programming
Lock-Free Algorithms
Thread Isolation and Suspension
Handling of Live Locks
-
65Unlocking ACID Principles in Lock-Free ProgrammingVideo lesson
This lecture is a comprehensive guide to understanding and implementing the ACID (Atomicity, Consistency, Isolation, Durability) principles in lock-free programming. It begins by establishing a parallel between transactional operations in databases and lock-free programming, highlighting the importance of treating code changes as transactions.
The first principle discussed is Atomicity, which ensures that operations are "all or nothing," eliminating the possibility of intermediate states. This segment delves into the concept of atomic writes, which involve reading, modifying, and writing data in an inseparable sequence, thus maintaining data integrity.
Next, we explore Consistency, emphasizing that transactions must transition data from one consistent state to another. This principle ensures that any observable state of the data is either its original or final state, with no in-betweens.
Isolation is then examined, underscoring the need for transactions to operate on data without simultaneous interference from other transactions. This ensures serialized processing where necessary, particularly when transactions interact with the same data set.
Durability, the final principle, ensures the permanence of a transaction's effects, even amidst concurrent operations. It addresses the 'lost update problem' by requiring subsequent transactions to recognize and respect the outcomes of preceding ones.
The course also addresses the complexities of concurrent updates and deletions in an ACID-compliant system, focusing on the unique challenges posed by lock-free environments.
Key Takeaways:
ACID Principles in Lock-Free Programming
Atomicity, Consistency, Isolation, Durability
Concurrent Updates and Deletions
Transactional Integrity and Data Consistency
-
66Essentials of Atomic Operations in C++11: A Practical OverviewVideo lesson
This lecture serves as an introduction to the semantics and operations of atomic data types in C++11, setting the stage for more advanced design discussions in upcoming sessions. It begins by clarifying the role of atomic types in ensuring that no external locking is necessary when working with these variables. This feature is a cornerstone of C++11's approach to concurrency and atomicity.
The course explains that while the C++ standard does not prevent compilers from using internal locking, it guarantees that atomic operations do not require external locking mechanisms. This aspect of atomicity is crucial in maintaining the ACID properties in multithreaded environments.
The discussion then moves to atomic operations' ability to prevent reordering of reads and writes, highlighting the importance of the 'atomic' keyword in imposing restrictions on the compiler during code generation. This ensures that the compilers respect the specified ordering.
Key operations like Compare and Swap (CAS), Compare Exchange Strong, and Compare Exchange Weak are explored. The lecture emphasizes that Compare Exchange Weak may require continuous checking in a loop due to the possibility of spurious failures, necessitating careful implementation.
The use of the 'atomic' keyword with user-defined types is also addressed, noting that the memory layout of these objects might differ when used as atomic. This can be particularly relevant in performance engineering scenarios.
Key Takeaways:
Atomic Data Types in C++11
No External Locking Required
Read and Write Order Preservation
Compare and Swap, Compare Exchange Operations
Atomic Operations with User-Defined Types
-
67Advanced Atomic Operations in C++: Mastering Concurrency and LockingVideo lesson
This lecture dives into the nuanced considerations of using the atomic keyword in C++ for concurrent programming. It begins by explaining how atomic operations for small or built-in types (like Plain Old Data types) are typically implemented without locks on most platforms, utilizing specific assembly instructions.
The discussion then shifts to larger, user-defined data types, where lock-based atomicity might be necessary due to alignment issues. When dealing with data types that don't align with the platform's atomic assembly instructions, software simulation using locks becomes essential. It's highlighted that programmers should always refer to documentation for specific details, as there's no universal rule in these scenarios.
Another key point covered is the need for explicit initialization of certain atomic variables. Due to the guarantees required by atomicity, some atomic variables don't initialize automatically, necessitating manual initialization.
The lecture also addresses the challenge of interleaved calls among threads. It explains that while an atomic operation ensures that a change by one thread is atomic, it doesn't prevent other threads from legally updating the atomic variable. This leads to potential issues like spurious updates, where a thread might incorrectly assume the value of an atomic variable hasn't changed.
Lastly, the distinction between atomicity and logical transactions is clarified. An example is used to illustrate how atomic operations on individual variables don't guarantee atomicity for a sequence of operations. This scenario often necessitates external locking to ensure the atomicity of the entire transaction, not just individual operations.
Key Takeaways:
Atomic Operations in C++
Lock-Based Atomicity for Large Data Types
Explicit Initialization of Atomic Variables
Challenges with Interleaved Calls and Spurious Updates
Difference Between Atomicity and Logical Transactions
-
68Quick CheckQuiz
Wait free, lock free and obstruction free programming paradigms
-
69Implementing Double-Check Locking in C++: From Single to Multi-threaded ProgramVideo lesson
This lecture provides a detailed exploration of the double-check locking pattern, particularly in the context of C++ programming. The session begins by introducing the concept in a single-threaded environment. The primary objective of double-check locking is to ensure the singleton pattern is maintained, guaranteeing a single instance of a resource in the system.
The course then delves into the implementation specifics using C++ semantics, particularly focusing on the enhancements offered by the concurrent and atomic features of C++11. Initially, the lecture illustrates the creation of a member pointer, initialized to null, and a method to manage this pointer. This method is responsible for either initializing the pointer if it doesn't exist or returning the existing instance.
While this method works well in a single-threaded application, the lecture points out its limitations in a concurrent environment. The session aims to bridge this gap by demonstrating how to adapt the double-check locking pattern for multi-threaded applications, addressing the challenges and nuances involved in this transition.
Key Takeaways:
Double-Check Locking Pattern
Singleton Pattern in C++
C++11 Concurrency and Atomic Features
Adaptation for Multi-threaded Environments
-
70Code walkthrough of concurrent implementation using mutex and atomics of C++11Video lesson
In this lecture, we delve into the advanced implementation of the double-check locking pattern in C++, focusing on thread safety. The session starts by introducing an atomic pointer as a central element in this pattern, used to manage a singleton instance in a multi-threaded environment.
Each thread attempting to access the singleton instance performs an atomic check to see if the instance is already initialized. This check ensures that even when multiple threads access the object simultaneously, their operations remain in separate contexts without overlap.
The critical aspect of this pattern is ensuring that no two threads initialize the singleton instance concurrently. To achieve this, a mutex is introduced, wrapped in a lock guard to leverage the RAII (Resource Acquisition Is Initialization) paradigm. This ensures the mutex is automatically released at the end of the scope or in case of an exception, allowing only one thread to proceed with initialization.
A key point emphasized in the lecture is the necessity of a second check after acquiring the mutex. This is to ensure that the instance hasn't been initialized by another thread in the interim. Only after confirming the instance is still null, the thread proceeds to create the new instance.
The lecture also highlights the robustness of this pattern in modern C++ implementations compared to older pthread-based implementations, which had issues with thread safety. The integration of atomic operations in C++ ensures that the double-check locking pattern is reliably thread-safe.
Key Takeaways:
Double-Check Locking Pattern
Thread Safety in Multi-Threaded C++ Applications
Atomic Pointers and Mutex Usage
RAII Paradigm in Resource Management
Importance of Second Check in Initialization
-
71Efficient Lazy Initialization in C++11: Leveraging Unique Pointers and Once FlagVideo lesson
This lecture presents an advanced and efficient method for lazy initialization in C++11, showcasing how it surpasses traditional mutex-based solutions. The approach hinges on two key elements: a unique pointer for instance management and a static std::once_flag. The unique pointer, initialized to null, ensures exclusive ownership and safe memory management of the instance. The std::once_flag acts as a gatekeeper, allowing the initialization code to be executed exactly once, thereby preventing multiple initializations in a multi-threaded environment.
The core of this technique involves using std::call_once along with a lambda function that returns the unique pointer. The std::once_flag is passed as an argument to std::call_once, ensuring that the initialization occurs only if the flag has not been set previously. This mechanism guarantees that only one thread can successfully set the flag and initialize the instance, while others find it already set, thus maintaining thread safety.
The advantages of this method are numerous. It avoids raw pointers by utilizing a unique pointer, ensuring automatic cleanup and scope-bound memory management. The minimal boilerplate makes the technique easily replicable and adaptable for various user-defined types. Furthermore, the transparency of this method aids in debugging and understanding the code, as it leaves no room for hidden assumptions.
Key Takeaways:
Lazy Initialization in C++11
Use of Unique Pointers and std::once_flag
Thread Safety with std::call_once
Automatic Memory Management
Minimal Boilerplate and Enhanced Code Clarity
-
72The surpirisngly cleanest concurrent initlaization solution!Video lesson
-
73Optimizing Concurrent Data Structures in C++: Beyond Syntax to Effective DesignVideo lesson
This lecture provides an insightful examination of the requirements for designing concurrent data structures in C++. The primary focus is on achieving synchronized access to shared resources, ensuring consistency throughout the program's lifetime. The session emphasizes that the goal is not to adhere to a specific syntax, such as using mutexes or atomics, but to attain effective and safe access to shared data.
The course discusses the limitations of traditional locking using mutexes, highlighting that while it is a functional approach, it presents challenges in program composition. The reliance on mutexes often leads to cumbersome, defensive programming, potentially resulting in over-engineering or reckless practices. This method’s syntactical limitations mean compilers cannot enforce stricter rules, increasing the risk of errors.
Atomics are presented as a more risk-oriented alternative for achieving performance, requiring significant discipline to avoid issues like deadlocks. However, the integration of atomics into the language itself poses challenges for code review and design, especially given their widespread availability to all developers.
The lecture also touches on emerging challenges in concurrency, particularly with the advent of microservices and other patterns where concurrency is no longer aligned with an object's lifetime. This decoupling requires a more nuanced, trade-off-oriented approach to design, highlighting the importance of a deep understanding of both locks and atomics for architecting robust concurrent systems.
Key Takeaways:
Synchronized Access in Concurrent Data Structures
Challenges with Traditional Mutex-Based Locking
Disciplined Use of Atomics for Performance
Decoupling of Concurrency from Object Lifetime
Importance of Effective Design Over Syntax
-
74Section QuizQuiz
Quiz about the double-check locking based implementations using modern C++
-
75Crafting Lock-Free Singly Linked Lists in C++: A Guide to Concurrent Data StructVideo lesson
In this lecture, we delve into the intricacies of implementing a lock-free singly linked list in C++. The focus is on understanding and creating a data structure that can be safely used in concurrent environments without the need for external locks. This approach is crucial for developers aiming to build efficient and robust multithreaded applications.
The session begins by outlining the basic operations of a singly linked list: construction, destruction, finding elements, and pushing elements to the front. The challenge addressed is crafting a lock-free version of this seemingly simple data structure. The lecture emphasizes the importance of achieving lock-free operations, which inherently implies no reliance on external locks.
The choice of the singly linked list as the subject for this discussion is strategic. It serves as an accessible yet potent example for teaching the principles of concurrent data structure design in C++. The lecture avoids providing ready-to-use code, encouraging learners to engage with the material by writing their own implementations. This hands-on approach is geared towards fostering a deeper understanding of concurrent programming and enhancing career growth.
Key Takeaways:
Lock-Free Implementation in C++
Singly Linked List Operations
Concurrent Data Structure Design
Importance of Self-Coding for Learning
-
76Code walkthrough of the implementation using Modern C++ Concurrency featuresVideo lesson
-
77Code Walkthrough of Constructor, Destructor and Find function implementationVideo lesson
This lecture provides an in-depth analysis of implementing key functions in a lock-free singly linked list in C++. We start with the constructor, which, in this case, can be left as default. This is because no specific allocations or initializations are needed, and from a concurrency perspective, there is no risk of concurrent access during construction. The logic here is straightforward: an object cannot be concurrently accessed before it’s fully constructed.
The focus then shifts to the destructor, which plays a crucial role in managing the list's lifecycle. Unlike the constructor, the destructor cannot be defaulted. It involves loading the atomic head pointer and walking over the list to delete each allocated item. The key insight here is that concurrency control, such as locking, is unnecessary in the destructor. The reasoning is based on the idea that once the destructor is invoked (due to scope ending or an exception), no other thread can access the object, thus negating the need for synchronization within the destructor.
Lastly, we discuss the implementation of the 'find' function. Marked as 'const', it signifies a read-only operation. The function traverses the list, starting from the atomic head, to locate and return a reference to the desired object. If the object isn’t found, it returns a null pointer. It’s emphasized that the 'find' function can safely run concurrently with other 'find' operations, as all are read operations. According to the C++ standard’s definition of race conditions, the absence of at least one write operation among concurrent operations on data means there can be no race condition. Therefore, multiple concurrent reads, as in the case of the 'find' function, do not pose a concurrency issue.
Key Takeaways:
Lock-Free Singly Linked List Functions
Default Constructor Implementation
Destructor for Managing List Lifecycle
Race Condition-Free 'Find' Function
Safe Concurrency in Read-Only Operations
-
78Analysis of the push_front function of the concurrent singly linked listVideo lesson
This lecture delves into the implementation of the push front operation in a lock-free singly linked list in C++. We start by explaining the basics of the operation, which involves inserting a new node at the front of the list. The process begins with the current state of the list, where the head may point to existing nodes or none at all. In executing push front, a new node is allocated and set to point to the current first element, effectively becoming the new head of the list.
The implementation phase is straightforward: create a new node, assign it the value to be inserted, and set its next pointer to the current head. Finally, the head is updated to point to this new node. Initially, the new memory allocated for the node is not visible to other threads, hence requiring no protection.
However, concurrency considerations are crucial. For readers, the atomic nature of the head pointer ensures consistent and safe access to the list, as it always points to a valid memory location. The challenge arises with concurrent writers. If multiple threads attempt to insert at the same time, there's a contention issue where two nodes compete to be the head, potentially resulting in a lost update and a memory leak.
To resolve this, the lecture suggests replacing the simple head assignment with a weak compare and exchange operation. This method involves a loop that continually attempts to update the head pointer until successful, ensuring that all insertions are accounted for without losing any nodes. The concept of compare and exchange is briefly mentioned, with resources for further exploration provided.
Key Takeaways:
Lock-Free Singly Linked List
Push Front Operation Implementation
Concurrency Considerations in Insertions
Atomic Head Pointer Management
Weak Compare and Exchange to Resolve Contention
-
79Analysis of the pop_front function design for a concurrent singly linked listVideo lesson
In this lecture, we build upon our initial design of a lock-free singly linked list in C++ by introducing a critical enhancement: the 'pop front' function. This addition, missing from the earlier version, becomes a new element in the public interface of the list. The fundamental structure remains consistent, featuring the template class list, constructor, destructor, 'find' function, and 'push front' function, along with the atomic head pointer and the deletion of copy and assignment operators.
The 'pop front' function's primary goal is to remove the first element of the list. We examine the operation's stages: the initial state where the head points to the first element, the intermediate state where the head starts pointing to the second node, and the final state where the original head node is released. The implementation involves loading the current head into a local pointer, setting the head to the second node, and then cleaning up the original node.
However, concurrency analysis reveals challenges. Concurrent operations of 'pop front' and 'find' can lead to problems like dangling pointers, as 'find' might access a node being deleted by 'pop front'. Similarly, concurrent erase and insert operations by different writers can cause race conditions due to an inconsistent head pointer state.
To address these challenges, we explore replacing simple assignments with the 'compare and exchange weak' operation. This ensures safe assignments while accommodating the possibility of spurious failures, necessitating a loop to repeatedly attempt the operation. Despite this, reader and writer issues persist due to the potential for spurious wakeups, leading to the ABA problem, which will be discussed in the next section of the course.
Key Takeaways:
Implementation of 'Pop Front' in Lock-Free Singly Linked Lists
Stages of Node Deletion in 'Pop Front'
Concurrency Challenges with 'Find' and 'Pop Front'
Use of 'Compare and Exchange Weak' for Safe Assignments
Persistence of Reader and Writer Issues Leading to ABA Problem
-
80Section QuizQuiz
Concurrent singly linked list using modern C++
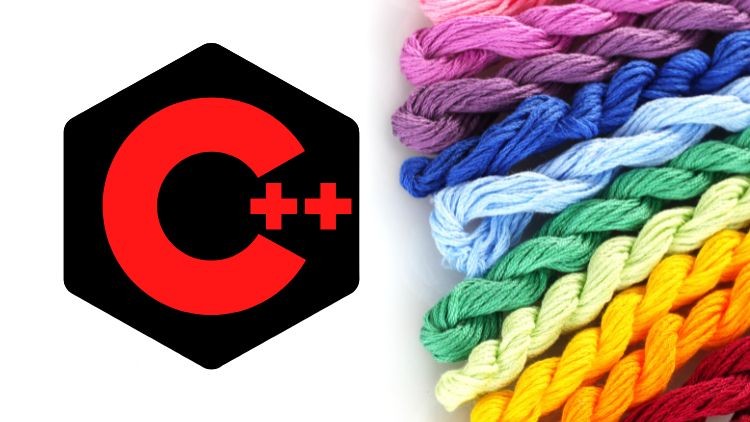
External Links May Contain Affiliate Links read more