Mastering Solidity, the Ethereum Programming Language
- Description
- Curriculum
- FAQ
- Reviews
This course aims to give an overview of what you need to know to program in Solidity. The course consists of two parts:In the first part, I explain best practices about developing Solidity code, without explaining the Solidity language much. The idea of the first part is to get you quickly started programming Solidity code.In the second part, I explain the Solidity language in depth.The first part of the course is ready and consists of the chapters 2 – 6. I have recorded the videos for the Sepolia testnet. Before the Sepolia testnet is end-of-life in Q4 2026, I will record the videos again for the following Ethereum testnet.In chapter 2, I explain some basic theory for people who do not know what a blockchain is.In chapter 3 – 5, I explain tools that are used to develop blockchain applications:These chapters explain the development tools Hardhat and Remix.You will see Solidity code for making your own cryptocurrency.You will see the JavaScript libraries ethers.js and web3.js that are used to interact with the Ethereum blockchain.You will see how you can connect a wallet with a blockchain application. I will show MetaMask, which is a popular wallet and also WalletConnect, which acts as a bridge to connect multiple kinds of wallets with an application. I will also show how you can transfer cryptocurrencies in MetaMask.You will see how to set up a local test blockchain on your computer and also how to interact with an Ethereum testnet on the Internet.You will see how you can register and configure an Ethereum Name.In chapter 6, I show how you can make a user interface that interacts with the Ethereum blockchain through the ethers.js library, it showshow to retrieve the address of a smart contract through an Ethereum Namehow to read data from the blockchain through a default providerhow to connect MetaMask or a wallet through WalletConnect with the user interfacehow to execute functions that write data to the blockchain through a connected wallethow to search for events emitted by a smart contractI am still working on the second part of the course that consists of all the chapters beginning from chapter 7. I will add more chapters over time. At the time of writing I have the following chapters online:Chapter 7 that explains what the following chapters of this part will be.Chapter 8 that explains the lexical elements of Solidity.Chapter 9 that explains an SPDX license and pragma directivesChapter 10 that explains the types in Solidity and how data is stored on the blockchainChapter 11 that explains functions in Solidity (except inheritance)Chapter 12 that explains inheritance in Solidity
-
4Chapter OverviewVideo lesson
Here, I give an overview of the other presentations in this chapter.
-
5Blockchain BasicsVideo lesson
In this presentation, I explain basic blockchain concepts. I explain:
what a blockchain is
how blocks in a blockchain refer to each other
how a blockchain can be made immutable
the role of validators
-
6Consensus MechanismsVideo lesson
In this presentation, I explain consensus mechanisms used by blockchains. I explain:
what a consensus mechanism is
Proof of Work (PoW)
Proof of Stake (PoS)
Proof of Authority (PoA)
I will compare PoW, PoS and PoA with each other
-
7Blockchain Theory TestQuiz
This test checks your knowledge about blockchains as explained in the "Blockchain Basics" and "Consensus Mechanisms" presentations.
-
8Blockchain in PracticeVideo lesson
In this presentation, I explain how blockchains are used in practice. I explain:
how public and private blockchains are used
Bitcoin (the biggest public blockchain)
Ethereum (the second biggest public blockchain)
how Ethereum will evolve over time
how costs are calculated when you write to the Ethereum blockchain
-
9Investing on the Ethereum BlockchainVideo lesson
In this presentation, I explain how you can invest on the Ethereum blockchain. I explain:
what fungible tokens are
what stablecoins are
how fungible tokens are traded in liquidity pools
what permanent or impermanent loss is in a liquidity pool
what non fungible tokens (NFTs) are
how you can invest in Ether and earn interest through a staking pool
-
10Blockchain DemoVideo lesson
In this presentation, I refer to a demo that illustrates how a blockchain works from a technical point of view.
The demo illustrates how Bitcoin works:
the demo illustrates a proof of work consensus mechanism
the demo illustrates how payment transactions are stored on the blockchain
Ethereum is a little bit different:
it uses a proof of stake consensus mechanism instead of proof of work
it stores programs instead of payment transactions
-
11Bitcoin White PaperText lesson
Here I refer to the Bitcoin White Paper.
-
12Ethereum PapersText lesson
Here I refer to the Ethereum White Paper, Yellow Paper and Beige Paper.
-
13Chapter OverviewVideo lesson
Here, I give an overview of the contents of this chapter.
-
14npmVideo lesson
Here I explain how to install npm, which is required for development
-
15Truffle and HardhatVideo lesson
Here I show the website of Truffle Suite and Hardhat, 2 popular development environments for Solidity. We will be using Hardhat in this course.
-
16Hardhat SetupVideo lesson
Here I show how to set up Hardhat.
-
17Hardhat Setup SummaryText lesson
Summary of the Hardhat Setup video
-
18Visual Studio CodeVideo lesson
Here I show where you could download the editor I will be using in this course: Visual Studio Code. This is for information only, you can use another editor!
-
19Hardhat Sample ProjectVideo lesson
Here we take a look at the sample project generated by Hardhat.
-
20Visual Studio Code ExtensionsVideo lesson
Here I compare the Visual Studio Code extension for Solidity made by Juan Blanco with the one made by the Nomic Foundation.
-
21ERC-20 StandardVideo lesson
Here I show some webpages with information about the ERC-20 standard and the GitHub repository of OpenZeppelin, which contains a standard implementation of the ERC-20 standard. We will extend this standard implementation to create our own cryptocurrency in this chapter.
-
22A First ContractVideo lesson
Here I show how we create our own cryptocurrency in Solidity.
-
23A First Contract SummaryText lesson
Summary of the A First Contract video
-
24Interacting With the Ethereum BlockchainVideo lesson
Here I show how we can interact with code deployed on the Ethereum blockchain.
-
25Testing Our First ContractVideo lesson
Here I show how we can test our first Solidity smart contract.
-
26Testing Our First Contract SummaryText lesson
Summary of Testing Our First Contract video
-
27Contract Size LimitVideo lesson
Here I explain that the maximum size of a compiled smart contract deployed to the Ethereum blockchain is 24 kB and I explain how you can get around this limit.
-
28Test Contract Size LimitVideo lesson
Here I explain how you can test the size of your compiled smart contracts.
-
29Test Contract Size Limit SummaryText lesson
Summary of Test Contract Size Limit video
-
30Hardhat ConfigurationVideo lesson
Here I gave an overview of what can be configured in Hardhat.
-
31Changing the Hardhat ConfigVideo lesson
Here I show:
how we can enable the optimizer in the Hardhat config and
how we can add blocks to the Hardhat test blockchain in intervals. By default, blocks are written instantly to the Hardhat test blockchain each time you write to the blockchain. This is not how Ethereum works. In Ethereum, blocks are not added instantly, but in intervals.
-
32Warning Against Sloppy CodeVideo lesson
Here I warn against some sloppy code I wrote in the previous video, which may work fine for tests that use Hardhat, but which is not guaranteed to work fine in production.
-
33Changing the Hardhat Config SummaryText lesson
-
34Hardhat LogVideo lesson
Here I show how you can log messages from your Solidity code to a console window.
-
35Hardhat Log SummaryText lesson
-
36Configuring Hardhat to Use web3.jsVideo lesson
Here I show how you can configure Hardhat to use web3.js.
-
37Configuring Hardhat to Use web3.js SummaryText lesson
-
38Change Contract Size Test to web3.js CodeVideo lesson
Here I show how the ethers.js code of the contract size test is changed to web3.js code.
-
39Change Contract Size Test to web3.js Code SummaryText lesson
-
40Change SeedToken Test to web3.js CodeVideo lesson
Here I show how the ethers.js code of the SeedToken unit tests is changed to web3.js code.
-
41Change SeedToken Test to web3.js Code SummaryText lesson
-
42A Closer Look at Hardhat, Truffle, ethers.js and web3.jsVideo lesson
Here I give an overview of the main development tools that are available to develop for the Ethereum blockchain: Hardhat Runner, Hardhat Network, Hardhat VSCode, Truffle, Ganache, Truffle for VSCode, ethers.js and web3.js
-
43Consensys Sunsets Truffle and GanacheText lesson
-
44Create Your Own Cryptocurrency and Add TestsText lesson
-
45Chapter OverviewVideo lesson
Here, I give an overview of the contents of this chapter.
-
46MetaMask WebsiteVideo lesson
Here I show the website of MetaMask. We will be using MetaMask as the wallet in which we store our ethers and cryptocurrencies and from which we make payments if we want to execute a function that writes to the Ethereum blockchain.
-
47MetaMask Set UpVideo lesson
Here I show how to install and set up MetaMask.
-
48Secret Recovery PhraseVideo lesson
Here I explain in more detail what a secret recovery phrase is.
-
49Set Up Hardhat Test BlockchainVideo lesson
Here I show how you can set up a Hardhat test blockchain and how you can connect MetaMask to it.
-
50Initialize Hardhat Test Blockchain: ScriptsVideo lesson
Here I show how you can initialize a Hardhat test blockchain with JavaScript code.
-
51Initialize Hardhat Test Blockchain SummaryText lesson
-
52Initialize Hardhat Test Blockchain: Run ScriptsVideo lesson
Here I execute the scripts to initialize a Hardhat test blockchain.
-
53Initialize Hardhat Test Blockchain: MetaMask CheckVideo lesson
Here we check with MetaMask that the Hardhat test blockchain is initialized with the script shown in the previous 2 videos.
-
54Transfer Cryptocurrencies With MetaMask: No Gas Estimate for Test BlockchainVideo lesson
Here I show an issue for sending cryptocurrencies on a Hardhat test blockchain with MetaMask: MetaMask does not have a valid gas estimate and therefore does not allow sending cryptocurrencies. I show how to fix this issue. This issue does not exist on the real Ethereum blockchain.
-
55Transfer Cryptocurrencies With MetaMask SummaryText lesson
-
56Transfer Cryptocurrencies With MetaMask: DemonstrationVideo lesson
Here I show how you can send cryptocurrencies from a MetaMask wallet on a Hardhat test blockchain. You can send cryptocurrencies in the same way on the real Ethereum blockchain.
-
57Transfer Cryptocurrencies With MetaMask: Clear ActivityVideo lesson
Here I show how you can clear the activity from a MetaMask account. If you reinitialize a test blockchain, you also have to reinitialize the MetaMask accounts used on that blockchain by clearing the activity of the accounts. It is not needed to clear the activity of an account on the real Ethereum blockchain, because the Ethereum blockchain is never reinitialized and keeps all history.
-
58Working With Blockchain NetworksVideo lesson
Here, I explain how you get ethers for the Ethereum mainnet and the Sepolia test blockchain. I also explain how to configure Ethereum blockchain networks in Hardhat and when to use them or when to use a local test blockchain.
-
59Interact With the Sepolia BlockchainVideo lesson
Here I show how to configure Hardhat to interact with the Sepolia test blockchain. You configure Hardhat the same way to interact with the Ethereum blockchain.
-
60Interact With the Sepolia Blockchain SummaryText lesson
-
61Private Key Format in Hardhat ConfigurationVideo lesson
Here I show how the Infura API key and the private keys of the accounts you add to a Hardhat configuration file look like and how you get these values. The private keys of the accounts have to be preceded by '0x' to denote that they are hexadecimal numbers.
-
62Recover MetaMask WalletVideo lesson
Here I show how you can recover your MetaMask wallet.
-
63MetaMask and Test BlockchainsText lesson
-
64Chapter OverviewVideo lesson
Here, I give an overview of the contents of this chapter.
-
65Remix IntroductionVideo lesson
Here I give an introduction to the Remix integrated development environment that can be used as an alternative to Visual Studio Code and Hardhat.
-
66Accessing Files With RemixVideo lesson
Here I show how you can access the file system with the Remix web application.
-
67Remix as a Desktop Application and WalletConnectVideo lesson
Here I show how you can run Remix as a desktop application, which solves the issue of accessing the file system, but introduces another issue: the Remix desktop application does not have browser extensions such as MetaMask. I show how you can circumvent the absence of MetaMask with WalletConnect.
-
68Remix and Solidity DependenciesVideo lesson
Here I explain that Visual Studio Code and Hardhat manage Solidity dependencies better than Remix.
-
69Factory Smart Contract and Deploy Through RemixVideo lesson
Here I show a factory smart contract that will create instances of our cryptocurrency smart contract and I also show how you can deploy this smart contract to the blockchain through Remix.
-
70Factory Smart Contract and Deploy Through Remix SummaryText lesson
-
71Ethereum Name ServiceVideo lesson
Here I show how you can register an Ethereum Name and how you can link it to an Ethereum address, such as the address of a smart contract.
-
72RemixText lesson
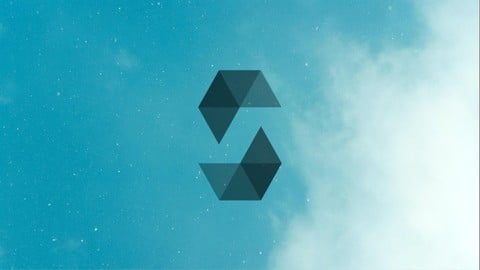
External Links May Contain Affiliate Links read more