Mastering SOLID Principles of Object Oriented Design (C#)
- Description
- Curriculum
- FAQ
- Reviews
Unlock the full potential of your C# programming skills with our comprehensive course on mastering SOLID principles. SOLID is an acronym for five foundational principles of object-oriented design that ensure your code is maintainable, scalable, and robust. These principles include Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion.
Throughout this course, you’ll dive deep into each of these principles with detailed explanations and practical examples tailored for C# developers. You’ll learn to identify and address common design issues, transforming your approach to software development. Through hands-on exercises, you’ll gain the skills to apply these principles effectively in real-world projects, enhancing your ability to write clean, efficient, and adaptable code.
This course is designed for developers at all levels, from beginners to seasoned professionals. If you’re new to object-oriented programming, you’ll gain a strong foundation in SOLID principles, setting you up for success in your programming career. Experienced developers will benefit from a deeper understanding and refinement of their coding practices, leading to more efficient and reliable software.
By the end of this course, you’ll have a solid grasp of how to implement SOLID principles in your C# projects, leading to better software development practices. Join us and elevate your coding proficiency, ensuring your applications are robust, flexible, and easy to maintain. Embrace the power of SOLID principles and revolutionize your software design with this essential course.
-
1Introduction to the CourseVideo lesson
Welcome to the SOLID Principles Course! In this brief introductory video, we'll give you a sneak peek into what this course is all about and why mastering SOLID principles is essential for any software developer. This video will provide an overview of the course structure, the key topics we'll cover, and the benefits you can expect from applying SOLID principles in your software design.
-
2Overview of SOLID PrinciplesVideo lesson
In this video, we dive into the fundamental concepts of software design. You'll learn why good design is crucial for creating effective, maintainable, and scalable software. We will also explore the challenges developers face in software design, such as complexity and flexibility, and discuss the serious consequences of poor design, including increased error rates and higher maintenance costs.
-
3What is Single Responsibility PrincipleVideo lesson
Understand the theory behind Single Responsibility Principle (SRP) and see what are the common signs in our code that could indicate SRP violations.
-
4Understanding and identifying SRP violation and fixing it - Code exampleVideo lesson
In this lecture we will look at a small piece of code catering to one functionality and try to understand and identify how this class is violating SRP. We will then try to fix the SRP violation by refactoring the code and splitting it into multiple classes.
-
5Benefits of conforming to Single Responsibility PrincipleVideo lesson
We will try to look at the changes we made and try to understand the benefits of SRP and how it leads to and is a first step in the direction of better design.
-
6What is Open-Closed PrincipleVideo lesson
Understand the theory behind Open-Closed Principle (OCP).
-
7Understanding and Identifying OCP violation and fixing it - Code exampleVideo lesson
Lets try to implement a simple user requirement and identify what are the common signs in our code that could indicate OCP violations. We will then try to fix the OCP violation by refactoring the code and introducing interfaced and splitting the single class into multiple classes.
-
8Benefits and importance of Open-Closed PrincipleVideo lesson
We will try to understand the benefits of OCP and how it another step in the direction of better design.
-
9What is Liskov Substitution Principle (LSP)Video lesson
Understand the theory behind Liskov Substitution Principle (LSP)
-
10Understanding and Identifying LSP violation and fixing it - Code exampleVideo lesson
Let's implement a simple user requirement and identify the common signs in our code that could indicate Liskov Substitution Principle (LSP) violations. We will then refactor the code to resolve these violations by ensuring that our derived classes can be used interchangeably with their base classes without altering the correctness of the program. This will help us adhere to the LSP, promoting more robust and maintainable code.
-
11Benefits and importance of Liskov Substitution Principle (LSP)Video lesson
We will explore the benefits of the Liskov Substitution Principle (LSP) and how it contributes to better software design. By adhering to LSP, we ensure that derived classes can replace their base classes without altering the program's behavior, leading to more reliable and flexible code.
-
12What is Interface Segregation Principle (ISP)Video lesson
In this video, you'll learn how ISP encourages creating smaller, more specific interfaces that are tailored to the needs of individual clients, rather than one large, general-purpose interface. This helps reduce the impact of changes and makes the system more maintainable and flexible.
-
13Understanding and Identifying ISP violation and fixing it - Code exampleVideo lesson
In this video, you'll explore how to identify and fix violations of the Interface Segregation Principle (ISP) in your code. Through a practical example, you'll learn how large, bloated interfaces can lead to issues when clients are forced to implement methods they don't need. The video will demonstrate how to refactor such interfaces into smaller, more focused ones, ensuring each client only depends on methods that are relevant to them, leading to cleaner and more maintainable code.
-
14Benefits and importance of Interface Segregation Principle (ISP)Video lesson
In this video, you'll discover the benefits and importance of the Interface Segregation Principle (ISP) in software design. ISP promotes the creation of small, focused interfaces, allowing for more flexible and modular code. You'll learn how adhering to ISP leads to easier maintenance, reduced complexity, and a more robust architecture by ensuring that clients only interact with the methods they actually need. This principle plays a crucial role in building scalable and adaptable systems.
-
15What is Dependency Inversion Principle (DIP)Video lesson
The Dependency Inversion Principle (DIP) promotes a design where high-level modules do not depend on low-level modules but instead rely on abstractions. It encourages decoupling dependencies to make code more flexible, maintainable, and easier to test.
-
16Understanding and Identifying DIP violation and fixing it - Code exampleVideo lesson
Explores how to recognize violations of the Dependency Inversion Principle (DIP) in code and demonstrates how to fix them. It likely walks through a code example where high-level modules are directly dependent on low-level implementations, causing tight coupling and reducing flexibility. The video then shows how to refactor the code by introducing interfaces or abstractions, making the high-level modules depend on these abstractions instead of concrete implementations. This process helps improve maintainability, testability, and scalability of the system.
-
17More Topics closely related to DIPVideo lesson
The video provides an in-depth explanation of key software architecture principles, focusing on Dependency Inversion Principle (DIP), Inversion of Control (IoC), IoC Containers, and Dependency Injection (DI).
-
18Benefits and importance of Dependency Inversion Principle (DIP)Video lesson
Highlights the advantages of adopting DIP in software development.
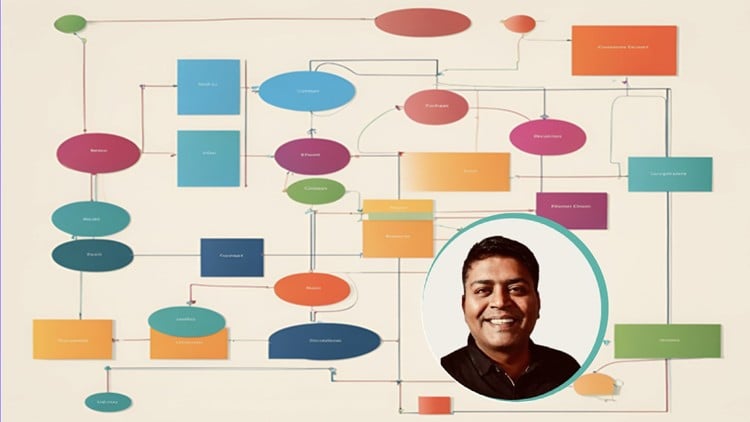
External Links May Contain Affiliate Links read more