Mastering REST APIs: Comprehensive Guide to REST APIs
- Description
- Curriculum
- FAQ
- Reviews
Introduction:
Dive into the world of REST APIs with this comprehensive course designed to equip you with the knowledge and skills necessary to build, manage, and integrate RESTful web services in Android applications. From basic concepts to advanced implementations using a variety of technologies, this course offers a thorough exploration of REST API development, ensuring you can create robust and efficient APIs for your projects.
Section 1: REST APIs
This section introduces the fundamentals of REST APIs, starting with an overview and progressing to practical applications using WAMP and PHP. You will learn to design user interfaces, establish HTTP connections using AsyncTask, and handle data responses effectively. The section also covers the use of Volley for network requests, including handling JSON data and image loaders, providing a strong foundation in Android REST API development.
Section 2: REST API with Flask and Python
Focusing on Flask and Python, this section covers the creation and execution of minimal APIs. You will explore key concepts such as endpoints, data formatting, request parsing, and inheritance. Through detailed examples and hands-on practice, you will gain the skills needed to build and manage REST APIs using Flask and Python, enhancing your ability to develop flexible and scalable web services.
Section 3: Project on Golang and MongoDB – Creating REST API
Engage in a project-based learning experience with Golang and MongoDB. This section guides you through setting up Docker containers, creating MongoDB clients, and interacting with databases using Postman. You will perform CRUD operations and handle person data, applying theoretical knowledge to real-world scenarios and building practical skills in REST API development.
Section 4: RESTful API Project – Employee Management System Tool
Develop a comprehensive Employee Management System using RESTful APIs in this section. You will create project dependencies, develop classes for departments and employees, and implement repositories and services. The section emphasizes building controllers and configuring project files, culminating in a fully functional management system that demonstrates your ability to create complex API-driven applications.
Section 5: REST Assured Java Case Study
This case study focuses on using REST Assured for API testing in Java. You will create JSON data, manage dependencies, and handle employee details through various operations. By performing CRUD operations and verifying outputs, you will gain valuable insights into automated testing and validation of REST APIs, ensuring your applications are robust and reliable.
Section 6: Project on REST API – Pet Clinic Application
The final section involves developing a Pet Clinic Application using REST APIs. You will set up a Maven project, create base entities, and develop model classes. The section covers creating repositories, handling REST exceptions, and developing serializers. By building REST controllers and services, you will complete a comprehensive application, solidifying your understanding and application of REST API development.
Conclusion:
By the end of this course, you will have mastered the principles and practices of REST API development for Android applications. Through a combination of theoretical knowledge and hands-on projects, you will be equipped to implement and manage REST APIs effectively, enhancing your skills and career prospects in Android development.
-
1Introduction to Web Service REST APIVideo lesson
This lecture introduces REST APIs (Representational State Transfer Application Programming Interfaces), explaining their role in web services. It covers the fundamental principles of REST, including statelessness, client-server architecture, and the use of standard HTTP methods (GET, POST, PUT, DELETE). The session provides a foundation for understanding how REST APIs enable communication between clients and servers.
-
2REST API PPTVideo lesson
This lecture presents a PowerPoint presentation on REST APIs, providing a structured overview of the concepts covered in the previous lecture. It includes visual aids and diagrams to illustrate REST principles, common use cases, and the structure of RESTful services. The presentation aims to reinforce understanding and provide a visual reference for REST API concepts.
-
3More on REST API PPTVideo lesson
Continuing from Lecture 2, this session delves deeper into the PowerPoint presentation on REST APIs. It covers additional details on RESTful architecture, advanced concepts, and real-world applications. The lecture expands on the initial presentation, offering more comprehensive insights into REST API design and implementation.
-
4Quiz on REST API IntroQuiz
-
5REST API Wamp with PHPVideo lesson
This lecture covers how to implement REST APIs using WAMP (Windows, Apache, MySQL, PHP) with PHP. It includes a step-by-step guide on setting up a local development environment, creating a basic REST API, and handling HTTP requests and responses using PHP. The session provides practical insights into building and testing RESTful services with WAMP.
-
6REST API Wamp with PHP ContinuesVideo lesson
Continuing from Lecture 4, this session further explores REST API development with WAMP and PHP. It includes advanced topics such as authentication, data validation, and error handling. The lecture provides additional examples and best practices for creating robust and secure RESTful APIs using PHP and the WAMP stack.
-
7Quiz on REST API Wamp with PHPQuiz
-
8REST API Android BasicVideo lesson
This lecture introduces the basics of integrating REST APIs with Android applications. It covers fundamental concepts such as making HTTP requests, handling responses, and parsing JSON data in an Android environment. The session provides a foundation for understanding how to consume REST APIs in mobile app development.
-
9Quiz on REST API Android BasicQuiz
-
10UI DesignVideo lesson
This lecture focuses on designing user interfaces (UI) for applications that consume REST APIs. It covers principles of UI design, including layout, usability, and responsiveness. The session provides guidance on creating intuitive and user-friendly interfaces that effectively present data retrieved from RESTful services.
-
11Connection with HTTP using Async Task ThreadVideo lesson
This lecture covers how to connect to REST APIs using HTTP in Android applications with asynchronous tasks. It explains the use of AsyncTask for handling network operations in the background, ensuring that the user interface remains responsive while data is being fetched from RESTful services.
-
12Quiz on AsyncQuiz
-
13Display Response DataVideo lesson
This lecture focuses on displaying data retrieved from REST APIs in an Android application. It covers techniques for presenting JSON data in user interfaces, including handling different data formats and updating UI elements with the fetched data. The session provides practical tips for ensuring that data is displayed clearly and effectively.
-
14Running DemoVideo lesson
This lecture provides a live demonstration of integrating and using REST APIs in an Android application. It includes a step-by-step walkthrough of a sample project, showcasing how to connect to an API, retrieve data, and display it in the app. The demo offers hands-on experience and reinforces concepts covered in previous lectures.
-
15REST API volley BasicVideo lesson
This lecture introduces Volley, a library for handling network operations in Android. It covers the basics of using Volley to make HTTP requests, manage responses, and handle errors. The session provides an overview of Volley’s features and benefits, making it easier to work with REST APIs in Android applications.
-
16UI Design and Single Class Design for VolleyVideo lesson
This lecture focuses on designing user interfaces and implementing a single class architecture for using Volley in Android applications. It includes best practices for organizing code, managing network requests, and updating the UI. The session aims to streamline the development process and ensure maintainable and scalable code.
-
17String Request Using VolleyVideo lesson
This lecture covers how to make string-based HTTP requests using the Volley library. It includes details on setting up string requests, handling responses, and processing data. The session provides practical examples of using Volley to retrieve and work with plain text data from REST APIs.
-
18String Request Using Volley ContinuesVideo lesson
Continuing from Lecture 13, this session delves deeper into string requests with Volley. It covers advanced topics such as request queuing, caching, and error handling. The lecture provides additional examples and best practices for managing string requests and optimizing network performance.
-
19Json Object Request Using VolleyVideo lesson
This lecture explores how to make JSON object requests using Volley. It includes details on parsing JSON responses, extracting data, and handling complex JSON structures. The session provides practical guidance on using Volley to work with JSON objects retrieved from REST APIs.
-
20Json Object Request Run DemoVideo lesson
This lecture features a live demonstration of making and handling JSON object requests with Volley. It includes a step-by-step walkthrough of a sample project, showcasing how to fetch and process JSON data in an Android application. The demo reinforces concepts and provides hands-on experience with Volley.
-
21JSON Array Request Using VolleyVideo lesson
This lecture covers how to handle JSON array requests using the Volley library. It includes techniques for parsing arrays, iterating through data, and displaying it in the user interface. The session provides practical examples of working with JSON arrays retrieved from REST APIs.
-
22Run Demo and Image LoaderVideo lesson
This lecture provides a live demonstration of using Volley to handle image loading in Android applications. It includes a walkthrough of integrating image requests with Volley, optimizing image loading, and displaying images efficiently in the app. The demo showcases practical use cases and performance considerations.
-
23Image Loader Using Volley and Run DemoVideo lesson
Continuing from Lecture 18, this session features an extended demonstration of using Volley for image loading. It includes advanced techniques for managing image requests, handling caching, and improving performance. The demo provides additional insights into optimizing image handling with Volley.
-
24Introduction and Installation of FlaskVideo lesson
-
25Writing Minimal APIVideo lesson
-
26Executing the APIVideo lesson
-
27EndPoints and Data FormattingVideo lesson
-
28Overview on All the ExamplesVideo lesson
-
29Overview on All the Examples ContinuesVideo lesson
-
30Understanding Data FormattingVideo lesson
-
31Arguments and ReqparsingVideo lesson
-
32Request Parsing InheritanceVideo lesson
-
33Intro to Gland Case StudyVideo lesson
-
34Running Docker ContainerVideo lesson
-
35Creating Mongo ClientVideo lesson
-
36Creating Mongo Client ContinueVideo lesson
-
37Database Using PostmanVideo lesson
-
38Database Using Postman ContinueVideo lesson
-
39Mongo dB Using PostmanVideo lesson
-
40Person Data Using IdVideo lesson
-
41Person Data Using Id ContinueVideo lesson
-
42Introduction to ProjectVideo lesson
-
43Creating a Project and Adding DependenciesVideo lesson
-
44Creating Department ClassVideo lesson
-
45Creating Employee ClassVideo lesson
-
46Department RepositoriesVideo lesson
-
47Department ServiceVideo lesson
-
48Employee ServiceVideo lesson
-
49Department ControllerVideo lesson
-
50Department Controller ContinueVideo lesson
-
51Employee ControllerVideo lesson
-
52Main FileVideo lesson
-
53Properties FileVideo lesson
-
54Introduction to ProjectVideo lesson
-
55Creating JSON DataVideo lesson
-
56Adding DependenciesVideo lesson
-
57Employee Details Part 1Video lesson
-
58Employee Details Part 2Video lesson
-
59Employee Details Part 3Video lesson
-
60Add Employee DetailsVideo lesson
-
61Update Employee DetailsVideo lesson
-
62Delete Employee DetailsVideo lesson
-
63Output of the ProjectVideo lesson
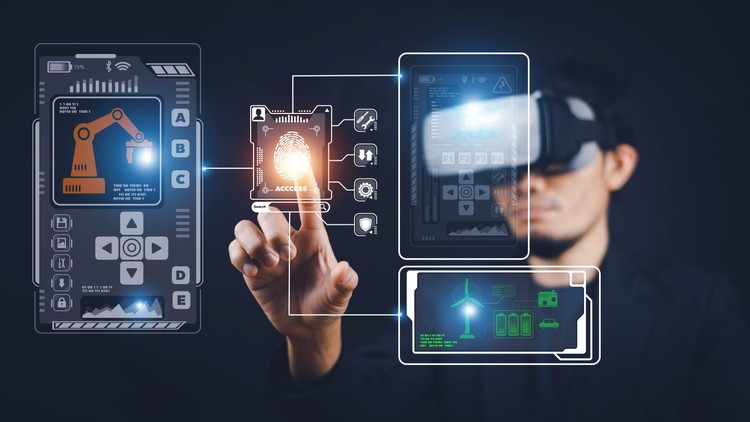
External Links May Contain Affiliate Links read more