Mastering JavaScript Arrays
- Description
- Curriculum
- FAQ
- Reviews
Effectively working with arrays is critical to any JavaScript programmer. Arrays are an important data structure, and this course will provide you with the competency you need to master them.
In this course you are going to learn all there is to know about JavaScript arrays. I start from the beginning, so if you are comfortable with JavaScript, you may want to use the first section as a review or skip it. You will learn the basics of different ways to create an array and work with the elements. You will learn the basics of iterating an array and some unique ways to create arrays and access elements. You will learn all the methods to modify or mutate an array, as well as those methods that are preferred in the functional programming world because they preserve the original array. You will learn how to combine arrays and extract values as well as multiple ways to search arrays for values. We will end with a look at some array-like collections available in JavaScript and how you can use those.
By taking this course:
-
You will feel more comfortable working with arrays in any coding problem you encounter.
-
You will become more familiar with functional methods for working with arrays (reduce, map, filter).
-
You will become familiar with and use all the different methods for manipulating an array in JavaScript.
-
You will be able to work with arrays using different techniques.
If you learn by doing, this course gives you plenty of chances to work on an exercise and then sit back and watch as I go through that exercise. Jump in today and begin mastering JavaScript Arrays!
-
3The Nature of JavaScript ArraysVideo lesson
-
4Creating ArraysVideo lesson
-
5Using Array.of to Create an ArrayVideo lesson
-
6Using Array.fill to Create an ArrayVideo lesson
-
7Reading and Writing Array ElementsVideo lesson
-
8Addressing Elements with the at MethodVideo lesson
-
9Sparse ArraysVideo lesson
-
10Working with the length PropertyVideo lesson
-
11Deleting Array ElementsVideo lesson
-
12Emptying an ArrayVideo lesson
-
13Using Arrays as Stacks (push and pop)Video lesson
-
14Using unshift and shiftVideo lesson
-
15Exercise 1 StartVideo lesson
-
16Exercise 1 FinishVideo lesson
-
17Iterating an Array with the for LoopVideo lesson
-
18Exercise 2 StartVideo lesson
-
19Exercise 2 FinishVideo lesson
-
20Review Topic: Understanding the PrototypeText lesson
-
21Review Topic: Understanding the Ternary OperatorText lesson
-
22Should You Use the for in Loop on Arrays?Video lesson
-
23Using the for of LoopVideo lesson
-
24Exercise 3 StartVideo lesson
-
25Exercise 3 FinishVideo lesson
-
26Understanding Array-like CollectionsVideo lesson
-
27Converting Array-like Collections to ArraysVideo lesson
-
28Converting a String to an ArrayVideo lesson
-
29Accessing Characters in a StringVideo lesson
-
30Using split to Create an Array from a StringVideo lesson
-
31Gathering Arguments into an ArrayVideo lesson
-
32Accessing Array Values with a StatementVideo lesson
-
33Converting Object Properties to an ArrayVideo lesson
-
34Exercise 4 StartVideo lesson
-
35Exercise 4 FinishVideo lesson
-
36Checking for Array-like CollectionsVideo lesson
-
37Should you Mutate an Array?Video lesson
-
38Reversing the Elements in an ArrayVideo lesson
-
39Sorting the Elements in an ArrayVideo lesson
-
40Sorting an Array of ObjectsVideo lesson
-
41Using the Splice MethodVideo lesson
-
42Copying Elements Within an ArrayVideo lesson
-
43Understanding the Array PrototypeVideo lesson
-
44Cloning an ArrayVideo lesson
-
45Method ChainingVideo lesson
-
46Exercise 5 StartVideo lesson
-
47Exercise 5 FinishVideo lesson
-
48Introducing Iterating over an Array Using Array MethodsVideo lesson
-
49Using the forEach MethodVideo lesson
-
50Using the map MethodVideo lesson
-
51Using the filter MethodVideo lesson
-
52Using the reduce and reduceRight MethodsVideo lesson
-
53Using the every MethodVideo lesson
-
54Using the some MethodVideo lesson
-
55Exercise 6 StartVideo lesson
-
56Exercise 6 FinishVideo lesson
-
57Review Topic: Arrow FunctionsText lesson
-
58Exercise 7 StartVideo lesson
-
59Exercise 7 FinishVideo lesson
-
60Exercise 8 StartVideo lesson
-
61Exercise 8 FinishVideo lesson
-
62Passing a Function to Array.fromVideo lesson
-
65IntroductionVideo lesson
-
66Using the join MethodVideo lesson
-
67Combining Arrays TogetherVideo lesson
-
68Extracting a Subarray from an ArrayVideo lesson
-
69Flatten an ArrayVideo lesson
-
70Understanding toStringVideo lesson
-
71Exercise 9 StartVideo lesson
-
72Exercise 9 FinishVideo lesson
-
73Exercise 10 StartVideo lesson
-
74Exercise 10 FinishVideo lesson
-
75Exercise 10 Follow UpVideo lesson
-
76Introduction to SearchingVideo lesson
-
77Using indexOf and lastIndexOfVideo lesson
-
78Checking an Array Using includesVideo lesson
-
79Review Topic: CoercionText lesson
-
80Using More Flexible Comparisons with findVideo lesson
-
81Using More Flexible Comparisons with findIndexVideo lesson
-
82Exercise 11 StartVideo lesson
-
83Exercise 11 FinishVideo lesson
-
84Exercise 12 StartVideo lesson
-
85Exercise 12 FinishVideo lesson
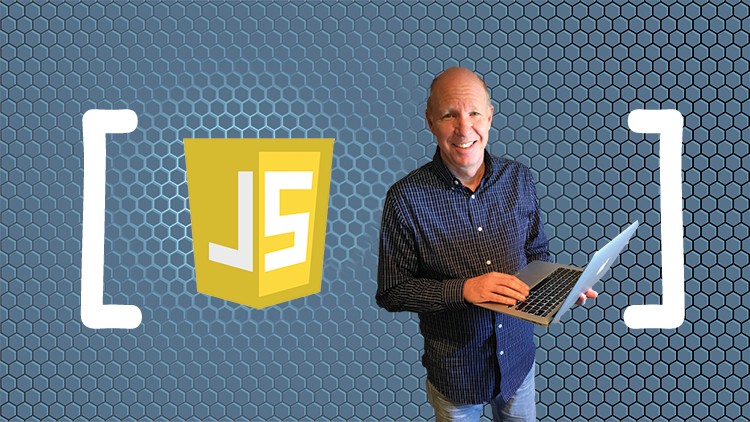
External Links May Contain Affiliate Links read more