Master the Fundamentals of Python
- Description
- Curriculum
- FAQ
- Reviews
Master the Fundamentals of Python is an extremely comprehensive course targeted for beginners who want to build their skills slowly and thoroughly without knowledge gaps. This course is packed full of material to ensure your understanding, regardless of your learning style, and includes the following:
Interactive Video Lessons
More than 25 hours of hands-on, interactive video lessons are provided. We will be programming together in the excellent Jupyter Notebook as we complete each module. Eventually, we will graduate to using Visual Studio Code, a more professional coding environment.
A Digital Book
You’ll get a 300+ page downloadable PDF of the book Master the Fundamentals of Python. This allows you to access all of the course contents in a single document, even when offline.
Exercises and Solutions
More than 200 exercises with detailed solutions are available for you to practice what you’ve learned.
Projects
There are several projects available where you’ll build larger programs that combine together multiple different topics. Some of the projects include Choose Your Own Adventure, Tic-Tac-Toe, and Texas Hold’em Poker with artificial intelligence.
Certification Exam
After covering all of the material in the course, you will be given a challenging certification exam to prove your mastery of the material. Passing this exam awards you a certificate of completion.
About the Instructor
This course is taught by expert instructor Teddy Petrou who is author of multiple books, including:
- Pandas Cookbook
- Master Data Analysis with Python
- Master the Fundamentals of Python
- Build an Interactive Data Analytics Dashboard with Python
Teddy has taught hundreds of students Python and data science during in-person classroom settings. He sees first hand exactly where students struggle and continually upgrades his material to minimize these struggles by providing a simple and direct path forward.
Teddy has demonstrated his deep fluency in Python by developing open source Python libraries and is the creator of dexplo, a suite of data science packages that include bar_chart_race, dexplot, jupyter_to_medium, and dataframe_image. He holds a Master’s degree in Statistics from Rice University.
Course Curriculum
- Operators
- Syntax
- Objects and types
- Strings
- Lists
- Ranges and constructors
- Conditional statements
- Writing entire programs
- Looping
- List comprehensions
- Built-in functions
- User-defined functions
- Tic-Tac-Toe
- Tuples, sets, dictionaries
- Modules
- User-defined modules
- Errors and exceptions
- Files
- Classes
- Texas hold’em poker
-
10Introduction to Jupyter NotebooksVideo lesson
-
11Jupyter Notebook BasicsVideo lesson
-
12Edit vs Command ModeVideo lesson
-
13Command Mode Keyboard ShortcutsVideo lesson
-
14Other Notebook TipsVideo lesson
-
15Markdown BasicsVideo lesson
-
16Exiting the Browser TabVideo lesson
-
17Completing ExercisesVideo lesson
-
18Creating New NotebooksVideo lesson
-
19Jupyter Notebook ExtensionsVideo lesson
Windows users - watch the next video if there are only a few extensions listed.
-
20Windows Issue with Notebook ExtensionsVideo lesson
Windows users - run the following command in your terminal: jupyter contrib nbextension install --user
-
21Jupyter Notebook SummaryVideo lesson
-
22Getting Started with the ModulesVideo lesson
-
23Arithmetic OperatorsVideo lesson
-
24More Arithmetic OperatorsVideo lesson
-
25Multiple Arithmetic OperatorsVideo lesson
-
26Change Operator Precedence with ParenthesesVideo lesson
-
27Comparison OperatorsVideo lesson
-
28Comparison and Arithmetic Operators TogetherVideo lesson
-
29Chained Comparison OperatorsVideo lesson
-
30Unary Plus and Minus OperatorsVideo lesson
-
31Boolean OperatorsVideo lesson
-
32The or OperatorVideo lesson
-
33The not OperatorVideo lesson
-
34Combining Boolean and Other OperatorsVideo lesson
-
35Assigning Values to Variable NamesVideo lesson
-
36Multiple VariablesVideo lesson
-
37Python CommentsVideo lesson
-
38Augmented Assignment StatementsVideo lesson
-
39Other OperatorsVideo lesson
-
40Open Project NotebookVideo lesson
-
41Project SolutionsVideo lesson
-
42What is Python?Video lesson
-
43What is a Computer Programming Language?Video lesson
-
44Programming Language ImplementationsVideo lesson
-
45Specific Example of Different ImplementationsVideo lesson
-
46Language SpecificationVideo lesson
-
47Python ImplementationsVideo lesson
-
48Python SyntaxVideo lesson
-
49Components of the Python Programming LanguageVideo lesson
-
50Whitespace and IndentationVideo lesson
-
51Long lines of codeVideo lesson
-
52Python is an Interactive LanguageVideo lesson
-
53Running Entire Python ProgramsVideo lesson
-
54Why use Python?Video lesson
-
55Module 2 ProjectVideo lesson
-
56Objects in the Real WorldVideo lesson
-
57An Introduction to Types in PythonVideo lesson
-
58Writing IntegersVideo lesson
-
59The Boolean TypeVideo lesson
-
60The Float TypeVideo lesson
-
61The Complex TypeVideo lesson
-
62The None ObjectVideo lesson
-
63Passing Variables to the type FunctionVideo lesson
-
64Object IdentityVideo lesson
-
65Dynamic TypingVideo lesson
-
66Built-in TypesVideo lesson
-
67Object Attributes and MethodsVideo lesson
-
68Accessing Attributes and Methods with Dot NotationVideo lesson
-
69What isn't an ObjectVideo lesson
-
70Module 3 SummaryVideo lesson
-
71Module 3 ProjectVideo lesson
-
72Introduction to StringsVideo lesson
-
73Strings Containing QuotesVideo lesson
-
74Strings with Escape CharactersVideo lesson
-
75Empty StringsVideo lesson
-
76UnicodeVideo lesson
-
77Operators with StringsVideo lesson
-
78MethodsVideo lesson
-
79Method ChainingVideo lesson
-
80Find the Length of a StringVideo lesson
-
81String InterpolationVideo lesson
-
82Selecting SubstringsVideo lesson
-
83Selecting Substrings with Slice NotationVideo lesson
-
84Changing the Characters of a StringVideo lesson
-
85Testing for a SubstringVideo lesson
-
86Module 4 SummaryVideo lesson
-
87Module 4 ProjectVideo lesson
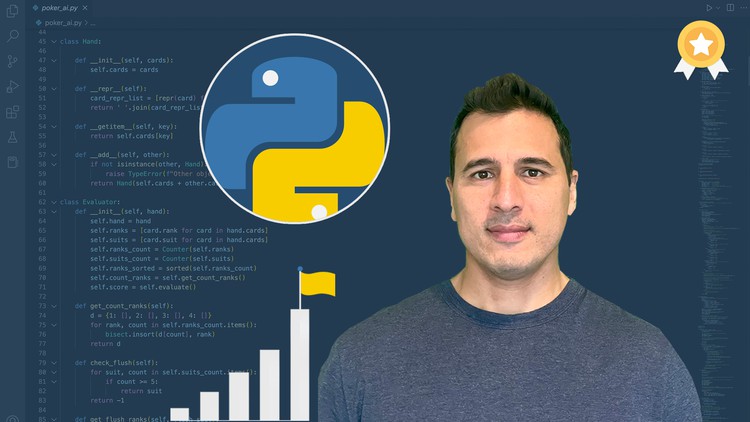
External Links May Contain Affiliate Links read more