Master Backend Development: Node, Docker, and MongoDB 2025
- Description
- Curriculum
- FAQ
- Reviews
What You’ll LearnMaster the art of building scalable and efficient backend servers with Node.jsConfigure a sophisticated Express server like a proContainerise and orchestrate your backend stack using Docker and Docker ComposeHandle MongoDB for database management with ease and confidenceLearn best practices for managing environment variables securelyEnhance your development process using nodemonDevelop REST APIs that are robust, secure, and scalableBuild production-ready backend systemsAnd much more to help you become the backend maestro you’re meant to be!RequirementsA computer with internet accessBasic understanding of JavaScript (ES6+) and REST APIsFamiliarity with Node.js package management (NPM)Willingness to learn and apply new concepts with confidenceNo need for prior Docker or MongoDB experience – we’ll cover it step by step!Need help? Ask questions anytime via the Q & A section or reach out directly for support.DescriptionAre you ready to step up your backend development skills and take them to the next level?In Master Backend Development, we will be exploring the popular trio of Node.js, Docker, and MongoDB, combining theory and practical application in an engaging, step-by-step format.We’ll start by setting up a robust Node.js Express server, dive deep into Docker for seamless containerization, and conquer MongoDB for database management. Together, we’ll tackle real-world challenges, so you’ll leave with hands-on experience and confidence to build scalable, efficient backend systems.By enrolling today, you’ll gain access to HD video lessons breaking down complex concepts into easy-to-follow steps. Plus, you’ll learn valuable skills that will benefit you for the rest of your career.What Exactly Will You Learn?Set up an advanced Node.js Express server from scratchDeep dive into how Express works under the hood.Use Docker to create a containerised application and enhance the development experienceImplement Docker Compose for orchestrating your Docker containersConfigure MongoDB inside a Docker containerConnecting your server to MongoDB using MongooseImplement validation using validate.jsHandle environment variables securely and efficientlyDebug backend issues with confidence using best practicesCreate and manage REST APIs like a professional developerOptimise your development workflow with tools like nodemonDevelop scalable, production-ready backend applicationsCourse StructureThis course is a hands-on, practical guide to building an Express server from the ground up. Each chapter builds on the previous one, and balances just the right amount of theory and knowledge, with majority of the lessons being practical coding. Build and configure an Express server from the ground upExplore Docker’s power to containerise and streamline your applicationsConfigure MongoDB and learn advanced database management techniquesUse Docker Compose to create multi-service applications effortlesslyDevelop REST APIs that are secure, efficient, and scalableYou’ll follow along with live demonstrations, and by the end, you’ll have a system you can replicate and expand upon for your own projects.Let’s embark on this journey together-step by step-to achieve backend mastery!Who This Course is ForDevelopers transitioning from beginner to intermediate levelsAnyone who knows JavaScript fundamentals and is eager to master backend developmentEmployees seeking to upskill for a career in backend programmingFreelancers and aspiring developers who want to build scalable, professional-grade systemsNo matter your background, this course will guide you through every step of becoming a confident backend developer.Get started now and transform your skills today!
-
7A Quick Explanation of DockerVideo lesson
-
8Installing Docker on Your Local MachineVideo lesson
-
9Creating a Dockerfile for Our AppVideo lesson
-
10Exploring Our Docker Images and ContainersVideo lesson
-
11A Brief Look at Overwriting in DockerVideo lesson
-
12Creating Convenient npm Scripts for Docker CommandsVideo lesson
-
13Module 3 ObjectivesVideo lesson
-
14Application CheckpointVideo lesson
-
15Understanding the Root Cause of the ProblemVideo lesson
-
16Setting up Docker VolumesVideo lesson
Docker run command:
docker run -v ./:user/src/app --name api my-app/express-api:latest-dev
-
17Module 3 ConclusionVideo lesson
-
32Module 7 ObjectivesVideo lesson
-
33An Overview of Our Router DesignVideo lesson
-
34Taking a Look at How Express Middleware WorksVideo lesson
-
35Exploring How the Express Router WorksVideo lesson
-
36Moving the Router Out of the Server.jsVideo lesson
-
37Setting up a Default RouterVideo lesson
-
38Wiring the Default Router to SetupRoutesVideo lesson
-
39A Quick Rundown of Our Middleware ChainVideo lesson
-
40Implementing More Default RoutesVideo lesson
-
41Refactoring with ConfigVideo lesson
-
42Module 8 objectivesVideo lesson
-
43An overview of the MongoDB driver sestupText lesson
-
44Adding a MongoDB initialiser to our APIVideo lesson
-
45Installing MongooseVideo lesson
Installing Mongoose for Your Project
To integrate Mongoose into your project, you will need to follow a series of steps carefully. Mongoose is a powerful tool for managing relationships between data, providing schema validation, and translating between objects in code and their representation in MongoDB.
Useful Resources:
Mongoose npm package
Official Mongoose Documentation
Installation Guide
Stop Docker Compose Containers: Before beginning the installation, ensure that all your Docker Compose containers are stopped to avoid any conflict. You can do this by running:
docker-compose down
Navigate to API Folder: Change your current directory to the API folder within your project where you wish to install Mongoose. Use the command:
cd api
Install Mongoose: While in the API folder, run the following npm command to install Mongoose:
npm install mongoose
Return to Project Root: After successfully installing Mongoose, navigate back to the root folder of your project:
cd ../
Rebuild Docker Compose API Image: To ensure that your Docker environment incorporates the newly installed Mongoose package, rebuild the API image using:
docker-compose build api
Rerun Docker Compose: Finally, to get your environment up and running again with the new installation, execute:
docker-compose up
By following these steps, you'll have Mongoose installed and ready for use in your project.
-
46Implementing config for MongoVideo lesson
-
47Implementing a Mongo DriverVideo lesson
-
48Updating the Docker Compose fileVideo lesson
-
49Refactoring the initialise appVideo lesson
-
50Setting up a User routerVideo lesson
-
51Wiring up a User GET requestVideo lesson
-
52Implementing a model layer for UserVideo lesson
I have created a txt file that contains the curl command that can be imported into Postman.
-
53Fixing the GET service functionVideo lesson
-
54Timestamps with mongoose middleware functionsVideo lesson
-
55Creating a PUT requestVideo lesson
-
56Creating a DELETE requestVideo lesson
-
57Module objectivesVideo lesson
-
58Understanding our validation issuesVideo lesson
-
59Basic Mongoose schema validationVideo lesson
-
60Preventing duplicate email addressesVideo lesson
-
61Creating our custom validation logicVideo lesson
-
62Installing and implementing validate.jsVideo lesson
-
63Wiring validate.js to our schemaVideo lesson
-
64Name attribute validationVideo lesson
Regex referenced in the lesson:
const regex = "[-'A-Za-z ]+";
-
65Refactoring the catch block errorsVideo lesson
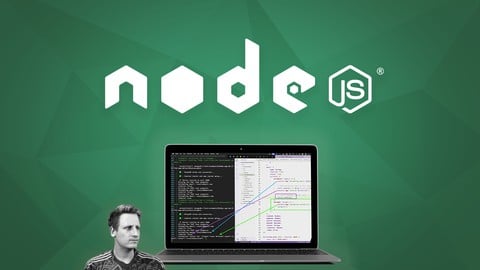
External Links May Contain Affiliate Links read more