Master Angular 17 + 18: Stay Ahead with the Latest Features
- Description
- Curriculum
- FAQ
- Reviews
Master Angular 17+18: Stay Ahead with the Latest Features
Stay ahead in modern frontend development with this Angular 17 and 18 course, tailored for developers looking to master the latest features, migrate from Angular 13, and build scalable, high-performance applications. Whether you’re focusing on Angular 17 or upgrading to Angular 18, this course equips you with essential skills to stay competitive.
Discover Angular’s powerful Signals feature for efficient state management and improved application reactivity. Dive into the latest advancements in Angular 17 and 18 to ensure your applications are optimized and future-proof.
Follow a clear, step-by-step migration path from Angular 13, addressing challenges in both versions. Learn how to use Defer for lazy loading, deferring non-critical tasks to enhance performance. Look at the future zoneless architecture, which eliminates the need for Zone.js, simplifying debugging and boosting performance.
Master practical features like Router Redirects as Functions for flexible routing logic and NG Content Placeholder for dynamic content projections, making your components reusable and efficient. Throughout the course, you’ll apply best practices while building a real-world application from scratch.
Please note: Server-Side Rendering (SSR) is not covered in this course. However, this program is perfect for developers eager to stay ahead with Angular’s latest advancements.
-
3Overview over the changes of Angular 17Video lesson
-
4Build-in Control FlowVideo lesson
-
5Check your knowledge about Build-in Control FlowQuiz
-
6Standalone ComponentsVideo lesson
Standalone Component
The new standard way to create a Component
- Simplified Module System: Standalone components in Angular allow you to create components without the need to declare them in an Angular module (`NgModule`). This simplifies the development process by reducing boilerplate and making it easier to manage component dependencies.
- Direct Dependency Injection: Standalone components can directly import dependencies like services, directives, and other components, eliminating the need to include these in an `NgModule`. This leads to more modular and reusable code.
- Improved Tree Shaking: By using standalone components, Angular can better optimize your application during the build process, resulting in smaller bundle sizes and improved load times. Unused code is more effectively removed, leading to better performance.
- Easier Lazy Loading: Standalone components make it easier to implement lazy loading since they are self-contained and can be loaded on demand without needing to load an entire module. This enhances application performance by reducing initial load times.
- Flexible Component Composition: Standalone components promote a more flexible approach to composing components in Angular. You can directly use standalone components in other parts of your application without the overhead of managing module imports.
- Enhanced Testing: Testing standalone components becomes more straightforward because you don't need to set up a testing module (`TestBed`) with all the necessary imports and declarations. This reduces setup time and complexity in unit tests.
-
7Check your knowledge about Standalone ComponentsQuiz
-
8DeferVideo lesson
Defer
A new way to Lazy Load Component
- Lazy Loading Optimization: The `@defer` annotation in Angular 17 allows parts of the application to be loaded only when needed, such as when they come into view, when the user interacts with them, or after a specified delay.
- Customizable Triggers: Developers can specify various triggers for deferred loading, such as `on viewport`, `on interaction`, `on hover`, `on timer`, or even custom conditions, offering fine-grained control over when components are loaded.
- Improved User Experience: By deferring the loading of non-essential components, the `@defer` annotation helps reduce initial load times and makes applications feel faster and more responsive to users.
- Placeholder and Loading States: The `@defer` annotation allows developers to specify placeholder content that is displayed while the deferred content is being loaded, as well as a loading state to handle the appearance of the component while it's being fetched.
- Error Handling: In addition to placeholders and loading states, the `@defer` annotation supports an `@error` block, which can be used to display error messages if the deferred content fails to load.
- Prefetching Capabilities: The `@defer` annotation also supports prefetching of content, allowing developers to load resources in the background before they are needed, reducing potential delays when the content is eventually displayed.
-
9Check your knowledge on the @defer AnnotationQuiz
-
10SignalsVideo lesson
Signals
A new way to handle Data
- Reactive State Management: Signals provide a new way to manage and react to state changes, similar to how state management works in reactive frameworks like React.
- Automatic Change Detection: Signals optimize change detection by only updating components that depend on the signal, rather than re-running the entire change detection cycle.
- Declarative Syntax: Signals use a more declarative approach, making it clearer and easier to understand which parts of the application depend on specific pieces of state.
- Improved Performance: By reducing unnecessary updates and focusing change detection only where it's needed, Signals help improve the performance of Angular applications.
- Simplified Codebase: Signals can simplify complex state management code, reducing the need for manual subscriptions or complex reactive programming techniques.
-
11Check your knowledge about Angular SignalsQuiz
-
12Signals - Effect and SourcesVideo lesson
Effects
Managing Side Effects Reactively
- Purpose of Effects
Effects are designed to handle side effects like HTTP requests, logging, or interactions with services in a structured, reactive way. They allow you to decouple side effects from component logic.- Automatic Triggering
Effects automatically react to changes in their dependencies, ensuring that side effects are executed only when necessary, reducing boilerplate code.- Improved Testability
By isolating side effects in dedicated units, Effects make it easier to test components and application logic without needing to mock or simulate side effects directly.- Integration with Signals
Effects work seamlessly with Signals, enabling a unified reactive approach to handling both state and side effects in Angular applications.Signal Component Inputs
Streamlining Component Communication
- Reactive Data Binding
Signal Component Inputs automatically react to changes in state, enabling components to stay synchronized without manual subscription or additional logic.- Simplified Input Management
They simplify how data flows into components, reducing the need for lifecycle hooks like ngOnChanges to detect input changes.- Improved Readability
Using Signal Component Inputs makes component templates more intuitive and less reliant on imperatively managing input values.- Backward Compatibility
Signal Component Inputs can coexist with traditional @Input bindings, allowing a gradual migration to this new approach.toSignal
Bridging Observables and Signals
- Purpose of toSignal
Converts Observables into Signals, allowing developers to use reactive streams in a simpler and more declarative manner.- Easier State Management
With toSignal, Observables can feed into the Signal-based state system, providing synchronous, template-ready values.- Reactive Integration
Enables seamless interaction between Angular's reactivity model and existing RxJS-based solutions, making it easier to integrate legacy codebases.- Error and Completion Handling
Automatically handles Observable errors and completion, ensuring Signals remain stable without additional boilerplate.toObservable
Converting Signals to Observables
- Purpose of toObservable
Converts Signals into Observables, enabling the use of RxJS operators and advanced reactive programming techniques.- Seamless Integration
Facilitates the use of Signals in existing Angular applications that rely heavily on Observables for event streams or async operations.- Flexible Reactive API
Allows Signals to participate in complex reactive workflows, combining them with other Observables for richer interactions.- Gradual Migration
Supports incremental adoption of Signals while retaining existing Observable-based architecture, making the transition smooth and non-disruptive. -
13Check your knowledge about Effects, Signal Input, toSignal, toObservableQuiz
-
14Bootstrap your applicationVideo lesson
Boostrap your Application
Bootstrapping with `app.config.ts`
- Centralized Setup: The `app.config.ts` file centralizes configuration, streamlining the bootstrapping process by managing services, routes, and initial setup logic in one place.
- Modular Flexibility: It supports modular bootstrapping, allowing configurations for different aspects like HTTP interceptors and state management, improving code organization.
- Environment-Specific Configurations: Developers can easily customize bootstrapping for different environments, offering flexibility in setup.
- Standalone Components Support: Seamless integration with Angular’s standalone components simplifies their configuration and management, enhancing the developer experience.
-
15Test your knowledge on Angular Bootstrapping with app.config.tsQuiz
-
16Builder & LiveserverVideo lesson
New Builder and Vite in Angular 17
New Builder (`esbuild`)
- Performance Enhancement: Angular 17 introduces `esbuild` as the new default build system, replacing Webpack. This upgrade significantly improves build times, often by a factor of 2 to 4, resulting in faster production builds and a more efficient development process.
- Simplified Configuration: The new builder streamlines configuration and automatically optimizes bundle sizes. Developers can transition to `esbuild` by simply updating the `angular.json` file in existing projects, ensuring a smooth migration.
- Optimized Output: With `esbuild`, the generated code is more efficient and smaller, enhancing both performance and loading times for applications.
Vite as the Live Server
- Instant Feedback: Angular 17 integrates Vite as its new Live Server, providing a faster and more responsive development experience. Vite reloads the application instantly when changes are made, allowing developers to see updates in real-time.
- Enhanced Development Workflow: Vite is fully integrated with the Angular CLI, making the development process more seamless. It compiles and reloads applications automatically, reducing the overhead on developers and speeding up the feedback loop.
- Support for Standalone Components: Vite works seamlessly with Angular's standalone components, ensuring that changes are immediately reflected without requiring additional setup or configuration.
-
17Angular DevtoolsVideo lesson
Angular DevTools Overview
- Powerful Debugging: Angular DevTools is an official Chrome extension that provides developers with powerful tools for debugging and profiling Angular applications. It allows you to inspect component hierarchies, view detailed performance metrics, and track change detection cycles in real-time.
- Component Tree Visualization: With Angular DevTools, you can explore the component tree of your application, understanding how different components are nested and how they interact with each other. This makes it easier to diagnose issues related to component structure and state management.
- Performance Profiling: The extension includes a profiler that helps you identify performance bottlenecks in your application. By visualizing the time taken by change detection and rendering processes, you can optimize your application's performance effectively.
- Change Detection Tracking: Angular DevTools provides insights into the change detection mechanism of Angular. It shows which components are being checked and how often, helping you to fine-tune your application’s responsiveness and efficiency.
- Standalone Component Support: The latest versions of Angular DevTools fully support standalone components, allowing you to debug and profile these components just as easily as traditional Angular components.
Angular DevTools is an essential tool for Angular developers, enhancing the ability to build, debug, and optimize Angular applications efficiently.
-
18Angular 18 ChangesVideo lesson
-
19Angular 17 Comprehensive QuizQuiz
-
24Quick ExplainationText lesson
-
25Create app with base componentsVideo lesson
Code snippets
main-page.component.scss
:host {
min-height: 100vh;
display: flex;
flex-direction: column;
.weather-card-bg {
background: linear-gradient(0deg, #f27bb8, #585df3);
}
}
-
26Adding search and card base stylingVideo lesson
-
27Extend card to contain weather forecastVideo lesson
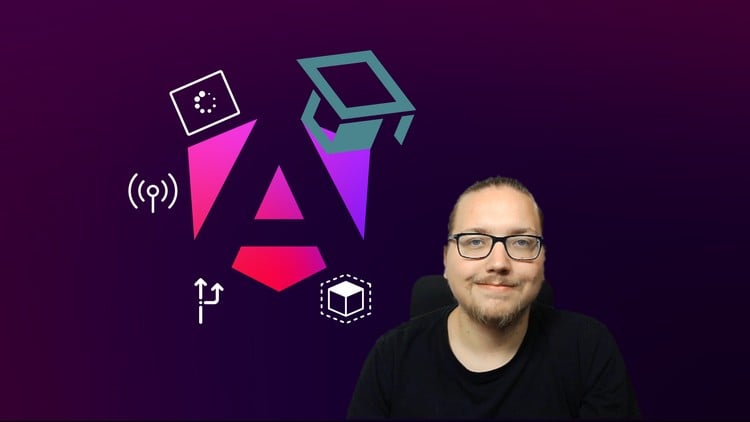
External Links May Contain Affiliate Links read more