Learn Python from a University Professor
- Description
- Curriculum
- FAQ
- Reviews
This course serves as an introduction to Python programming (no previous background needed). Whether you’re a high school student ahead of the game, a college student in search of additional support, a recent graduate preparing for a technical interview, an active professional seeking to expand your skill set, or just an amateur techie, this course is your ideal first exposure to the world of Python programming.
In this course, I’ve selected the most relevant topics to quickly get you started with Python. We’ll start with the basics: interaction with the user, arithmetic computations, conditional execution, loops, and functions. And we’ll also delve into more advanced topics: lists, tuples, dictionaries, file operations, and object-oriented programming. But most importantly: I’ll help you develop a programmer mindset by training your algorithmic thinking – an essential still that will help you quickly learn new programming languages in the future if you need to.
In most lessons of this course, I’ll be proposing interactive programming exercises, where I’ll ask you to pause the video and work on it, as I later guide through a step-by-step solution. You’ll work with a web-based code editor, where you’ll be able to write and test your programs, without installing any third-party software in your system; all you need is your web browser.
-
1The consoleVideo lesson
We’ll use this first lesson to introduce our live programming environment. First, I’ll show what it looks like to see a Python program executing, and how you, as a user of your own program, can interact with it. Then, I’ll introduce our interactive Python code editor, available here:
https://computersciencecamp.com/courses/python/tools
You'll be able to use the code editor to write and run your first program – one that simply prints a greeting message on the screen. After signing up on Computer Science Camp, you'll be able to create new Python projects and test your programs, as instructed on the lesson videos.
-
2The code editorVideo lesson
-
3A "hello world" programVideo lesson
-
4Python errorsVideo lesson
-
5VariablesVideo lesson
In this lesson we’ll introduce the concept of a “variable” as the a basic tool to save data in our program memory. We’ll then show how to leverage variables as a temporary storage for sequences of simple arithmetic computations. And we’ll conclude this lesson with a simple strategy to document our code through Python comments.
-
6ExpressionsVideo lesson
-
7Code commentsVideo lesson
-
8Reading keyboard inputVideo lesson
In this lesson, we cover basic user interaction in Python. Our hands-on work consists in creating a couple of programs that ask the user for an input through the keyboard. We then convert this input into different data types for further processing. The lesson concludes with some remarks about Python runtime errors, also known as exceptions.
-
9Data type conversionVideo lesson
-
10Python exceptionsVideo lesson
-
11Arithmetic operatorsVideo lesson
This lesson covers three types of built-in Python operators in detail: arithmetic, relational, and logical operators. We’ll see how to use these operators to increase the complexity and power of our computations, and you’ll practice their use by designing a simple interactive calculator.
-
12Relational operatorsVideo lesson
-
13Logical operatorsVideo lesson
-
14Importing modulesVideo lesson
In this lesson we study how Python extends its built-in functionality with external modules, and how your program can access these modules with special "import" statements. Our hands-on work includes two problems that focus on Python's “math” module.
-
15Problem: Distance between pointsVideo lesson
-
16Problem: Point coordinates in a circleVideo lesson
-
17The 'if' blockVideo lesson
So far, all of our Python programs have executed statements sequentially. In a program that has 3 lines of code, we expect line 1 to run first, then line 2, then line 3. While this execution model has already allowed us to introduce some level of interaction with the user, the real power of a programming language comes when you can alter the sequential control flow of your program by deciding what lines of code to execute, not to execute, or to execute multiple times based on certain conditions. In this lesson we introduce conditional execution as the most basic form of non-sequential control flow in Python.
-
18The 'if-else' blockVideo lesson
-
19The 'if-elif-else' blockVideo lesson
-
20Problem: Even-odd number detectorVideo lesson
In the previous lesson we’ve introduced three variations of the ‘if’ statement as a simple mechanism to control what code to execute based on conditions given as Boolean expressions. In this lesson, we’ll practice conditional execution with some interactive exercises. First, I’ll show you two examples for which you and I will be writing code together, and then I’ll propose a third problem that I’ll ask you to complete before I present my proposed solution.
-
21Problem: Triangle of sticksVideo lesson
-
22Problem: Conditional calculatorVideo lesson
-
23IndentationVideo lesson
In this lesson, I'll discuss two methods of indenting our code when using control flow, based on tabs and spaces. I'll present the advantages and disadvantages of each approach, and the built-in support for tab-based indentation in our code editor.
-
24The 'while' loopVideo lesson
In this lesson, I’ll introduce the ‘while’ loop as the most basic type of repetition control flow structure in Python. I’ll also use this lesson to define the concept of an “algorithm”, which will stay with us throughout the course, together with some preliminary algorithmic design guidelines using flowcharts.
-
25Algorithms and flowchartsVideo lesson
-
26Problem: Iterative square rootVideo lesson
-
27The 'break' statementVideo lesson
In this lesson I’ll introduce two Python keywords, called ‘break’ and ‘continue’, that allow us to alter the natural behavior of a loop, offering additional flexibility to control the repeated sequences of statements in our code. I’ll say a word or two about how to avoid getting stuck in infinite loops. And as always, we’ll conclude with a practice problem for you to internalize this new material.
-
28The 'continue' statementVideo lesson
-
29Infinite loopsVideo lesson
-
30Problem: Calculation of an averageVideo lesson
-
31ListsVideo lesson
In this lesson, I’ll provide a brief introduction to Python lists as a feature that allows us to group multiple values into one variable, and I’ll focus on a control flow structure called a ‘for’ loop, that allows us to conveniently traverse these sequences.
-
32The 'for' loopVideo lesson
-
33The 'range' functionVideo lesson
-
34Problem: Drawing a triangleVideo lesson
-
35Defining functionsVideo lesson
In the present chapter of this course will cover custom functions in detail: what is their main purpose, why are they necessary as the complexity of programs increases, how can we manage errors across functions, and more. In this lesson we’ll focus on the basics: how you can define your own functions and invoke them later from your main program.
-
36Function argumentsVideo lesson
-
37Problem: Printing a times tableVideo lesson
-
38Fruitful functionsVideo lesson
In this lesson we’ll increase our ability to communicate data between custom functions and a main program through the use of what we call “fruitful functions”. As a practice problem, we’ll work on an algorithm that translates numbers from decimal to binary (or base 2), as we leverage a fruitful function to pack this functionality. I’ll conclude with some remarks about the lifetime of variables within and across functions – what we call the “scope” of a variable.
-
39Problem: Base conversionVideo lesson
-
40Variable scopesVideo lesson
-
41Default argumentsVideo lesson
In this lesson I’ll introduce three new syntactic features related with function definitions and invocations that provide additional flexibility when dealing with argument passing. These features are called ‘default arguments’, ‘keyword arguments’, and ‘arbitrary arguments’.
-
42Keyword argumentsVideo lesson
-
43Arbitrary argumentsVideo lesson
-
44Problem: Printing arbitrary argumentsVideo lesson
-
45Recursion: Factorial calculationVideo lesson
Recursion is a method of solving a problem where its solution depends on solutions to smaller instances of the same problem. In Python we can write our own recursive functions, characterized by the fact that they invoke themselves within their own code. In this lesson, I’ll introduce this interesting idea bit by bit, by revisiting a familiar numeric algorithm —the calculation of the factorial of a number—, and I’ll show a detailed analysis of a recursive function that solves it.
-
46Recursion: Trace of factorial calculationVideo lesson
-
47Call treesVideo lesson
-
48Stack overflowVideo lesson
-
49Fibonacci numbersVideo lesson
In this lesson, I’ll continue discussing recursion through another numeric algorithm – the calculation of Fibonacci numbers. In the second part of the lesson, it’ll be time for you to get to work and design your own recursive function: one that identifies symmetric strings, also known as ‘palindromes’.
-
50Problem: Identifying palindromesVideo lesson
-
51ExceptionsVideo lesson
In this lesson we’ll talk about a specific kind of Python errors called ‘exceptions’. We’ll review some known situations in which such exceptions can occur. Then we’ll learn how to prevent exceptions from terminating a program, as we intercept them and handle them more gracefully. We’ll see how we can even raise our own custom exceptions. And finally, I’ll propose a problem where you’ll practice all these new concepts.
-
52Intercepting exceptionsVideo lesson
-
53Exception propagationVideo lesson
-
54Problem: Handling exceptionsVideo lesson
-
55Container data typesVideo lesson
In this lesson, I'll present some introductory concepts for this chapter related with data types, with a focus on container data types. I'll present a formal framework to assess the computational cost of operations on containers, and I'll show two syntactic features used to manage them, based on operators and functions.
-
56Computational costVideo lesson
-
57Operators, built-in functions, and methodsVideo lesson
-
58Basic list operationsVideo lesson
In this lesson, we’ll begin our deep dive into container data types by discussing Python lists. We’ll talk about the basic operations that allow us to declare, access, and manage lists, and I’ll propose an introductory problem for you to get your first hands-on experience with a list.
-
59Problem: Reading a list from the userVideo lesson
-
60List operatorsVideo lesson
In this lesson, we will expand our knowledge on Python lists by introducing a more advanced set of operators, built-in functions, and methods used to manage these structures. With these additional tools you will be able to design more advanced algorithms that rely on the use of lists.
-
61List functionsVideo lesson
-
62Representation of listsVideo lesson
In this lesson, we’ll delve into some details about how lists are internally represented, which will provide necessary insights on how to use lists as function arguments and return values. In the second part of this lesson, we’ll start with the first of a series of practice problems, where you’ll need to apply all concepts related with lists studied so far.
-
63Problem: Finding the minimum valueVideo lesson
-
64Problem: Sorted insertionVideo lesson
In this two-part lesson, you'll be working on two different problems related with lists, where you'll have the chance to practice the concepts studied in previous lessons.
-
65Problem: Deletion by contentVideo lesson
-
66Problem: Minimum frequencyVideo lesson
In this lesson you'll be working on two additional problems related with lists, with a level of completely that is slightly higher than the problems you've encountered so far.
-
67Problem: Second maximum elementVideo lesson
-
68Working with tuplesVideo lesson
In this lesson I’ll introduce a container data type, similar to a list, called a ‘tuple’. We’ll look at the syntax used to define and access tuples, the contexts in which you’ll want to use them, and the operators and functions related with them.
-
69Applications of tuplesVideo lesson
-
70Tuple operators and functionsVideo lesson
-
71Problem: Statistics of a data setVideo lesson
In this lesson, I'll propose a problem for you to practice the use of tuples as a way of returning multiple values in a function.
-
72Problem: Sorting a list of tuplesVideo lesson
In this lesson, I'll propose a problem where you'll need to process a data set organized as a list of tuples by sorting it in ascending order.
-
73Working with dictionariesVideo lesson
In this lesson, I’ll introduce Python dictionaries as powerful, widely used container data structures. We’ll learn how to create, modify, and query dictionaries, and I’ll discuss the uniquely efficient cost of these operations.
-
74Basic dictionary operationsVideo lesson
-
75Problem: Managing a dictionaryVideo lesson
In this lesson, I’ll propose an introductory problem to practice the creation of a dictionary followed by runtime insertions and updates.
-
76Dictionary operators and functionsVideo lesson
In this lesson I’ll expand our ability to manage dictionaries by presenting additional operators, built-in functions, and methods available for them.
-
77Direct-access tablesVideo lesson
As I was describing the operations supported by dictionaries, I've emphasized the ability of searches, insertions, and deletions to operate with *constant* cost. This means that, whether a dictionary has ten, one thousand, or one million key-value pairs in it, searching for one will take the same amount of time. In this lesson I'll describe the magic used by Python under the covers in order to accomplish this type of efficient behavior in dictionaries.
-
78Hash tablesVideo lesson
-
79CollisionsVideo lesson
-
80Strings as keysVideo lesson
-
81Problem: Merging dictionariesVideo lesson
-
82String declarationVideo lesson
Strings occur frequently in programs, and Python provides a variety of tools to process them. Processing a string may involve converting it to uppercase, finding occurrences of a sub-string in it, or formatting it before printing it into the terminal, just to name a few examples. This chapter will be dedicated to more advanced Python features to perform these tasks and more.
-
83Escaped charactersVideo lesson
-
84String slicesVideo lesson
-
85String compositionVideo lesson
-
86String formattingVideo lesson
Converting any data type into a string is useful when presenting that information to the user on the console through ‘print’ statements, and here we face the opportunity to format those strings in the most user-friendly way possible. In this lesson, we’ll study the tools provided by Python to conveniently format strings with the desired alignment, padding, and other layout preferences.
-
87AlignmentVideo lesson
-
88Floating-point formattingVideo lesson
-
89Problem: Printing a tableVideo lesson
-
90String operators and functionsVideo lesson
In this lesson I'll present a list of frequently used operators and functions related with strings, which will complement slicing, formatting, concatenation, and other features studied before.
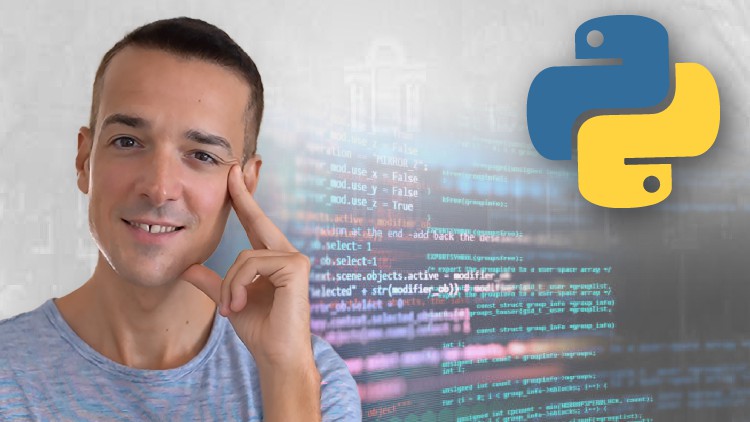
External Links May Contain Affiliate Links read more