JavaScript: The Critical Parts Masterclass
- Description
- Curriculum
- FAQ
- Reviews
This course focusses on and explains thoroughly the critical concepts and topics found in JavaScript. It dives into important fundaments that often get glossed over. And then takes on the more advanced techniques in JavaScript and explores them deeply. The course not only covers the HOW, it also focusses on the WHY in order to increase your understanding. The HOW and WHY are both critical to becoming a top JavaScript developer.
At 25 hours of instruction, you will master JavaScript in a way only top JavaScript developers do. Here is why:
-
The course is taught by the lead trainer at All Things JavaScript, whose mission is to facilitate your journey from novice to expert.
-
The course is constantly updated with new content and new topics.
-
The course focusses on JavaScript, so you learn JavaScript fully without worrying about ancillary technologies.
-
The course explores how things work under the hood so your understanding is deep and relevant.
-
The curriculum touches multiple aspects of JavaScript.
-
The curriculum was developed over a period of several years.
If you want to improve your JavaScript skills, this course is for you!
The topics you will learn in this course are timeless and will continue to serve you for years in a promising career as a JavaScript developer. You will not find a course as detailed and as in-depth as this course and the concepts and topics taught will put you in the top of all JavaScript developers.
-
6IntroductionVideo lesson
-
7The JavaScript Runtime EnvironmentVideo lesson
-
8Understanding the JavaScript EngineVideo lesson
-
9The Memory Heap and Call StackVideo lesson
-
10Understanding Garbage CollectionVideo lesson
-
11Removing Event ListenersVideo lesson
-
12Understanding the Event LoopVideo lesson
-
13The Node Runtime EnvironmentVideo lesson
-
14Exercise Start: Exploring Call Stack and Event LoopVideo lesson
-
15Exercise End: Exploring Call Stack and Event LoopVideo lesson
-
16How JavaScript EvolvesVideo lesson
-
17Section IntroductionVideo lesson
-
18The Execution ContextVideo lesson
-
19A Consistent Global ObjectVideo lesson
-
20HoistingVideo lesson
-
21Function Declarations Versus Function ExpressionsVideo lesson
-
22Exercise Start: Function Execution ContextVideo lesson
-
23Exercise End: Function Execution ContextVideo lesson
-
24Lexical EnvironmentVideo lesson
-
25Scope and the Scope ChainVideo lesson
-
26Block ScopeVideo lesson
-
27Exercise: Using let in a for LoopVideo lesson
-
28Creating ConstantsVideo lesson
-
29Should You Continue Using var?Video lesson
-
30Global Variables and the Danger of the Scope ChainVideo lesson
-
31Exercise Start: ScopeVideo lesson
-
32Exercise End: ScopeVideo lesson
-
33JavaScript and Data TypesVideo lesson
-
34Objects are EverywhereVideo lesson
-
35Type CoercionVideo lesson
-
36Making Use of Truthy and FalsyVideo lesson
-
37Loose Equality Versus Strict EqualityVideo lesson
-
38Exercise Start: CoercionVideo lesson
-
39Exercise End: CoercionVideo lesson
-
40Using the BigInt TypeVideo lesson
-
41Passing Primitives and Objects: Value or Reference?Video lesson
-
42Cloning JavaScript ObjectsVideo lesson
-
43Exercise: Pass by ReferenceText lesson
-
44Exercise Start: Pass by Reference and CloningVideo lesson
-
45Exercise End: Pass by Reference and CloningVideo lesson
-
46Using Strict ModeVideo lesson
-
47Tricky Fundamentals IntroductionVideo lesson
-
48CallbacksVideo lesson
-
49Understanding the Keyword thisVideo lesson
-
50Using the Keyword this with Object MethodsVideo lesson
-
51Exercise Start: The Keyword thisVideo lesson
-
52Exercise End: The Keyword thisVideo lesson
-
53Common Issues with the Keyword thisVideo lesson
-
54Using call() and apply()Video lesson
-
55Using bind()Video lesson
-
56Taking Control of the Keyword thisVideo lesson
-
57Exercise Start: Using call(), apply() or bind()Video lesson
-
58Exercise End: Using call(), apply() or bind()Video lesson
-
59Arrow FunctionsVideo lesson
-
60Solving this Binding with Arrow FunctionsVideo lesson
-
61Arrow Functions are NOT for Every OccasionVideo lesson
-
62IntroductionVideo lesson
-
63Functions are First Class CitizensVideo lesson
-
64Higher Order FunctionsVideo lesson
-
65Creating Your Own Higher Order FunctionsVideo lesson
-
66Exercise Start: Create Higher Order FunctionVideo lesson
-
67Exercise End: Create Higher Order FunctionVideo lesson
-
68ClosuresVideo lesson
-
69Using Closure with Returned FunctionsVideo lesson
-
70Important Features of ClosuresVideo lesson
-
71Looking Ahead: The Traditional Module PatternVideo lesson
-
72Exercise Start: Closures 1Video lesson
-
73Exercise End: Closures 1Video lesson
-
74Exercise Start: Closures 2Video lesson
-
75Exercise End: Closures 2Video lesson
-
76Immediately Invoked Function Expressions (IIFEs)Video lesson
-
77Applying IIFEsVideo lesson
-
78Exercise Start: IFFEsVideo lesson
-
79Exercise End: IFFEsVideo lesson
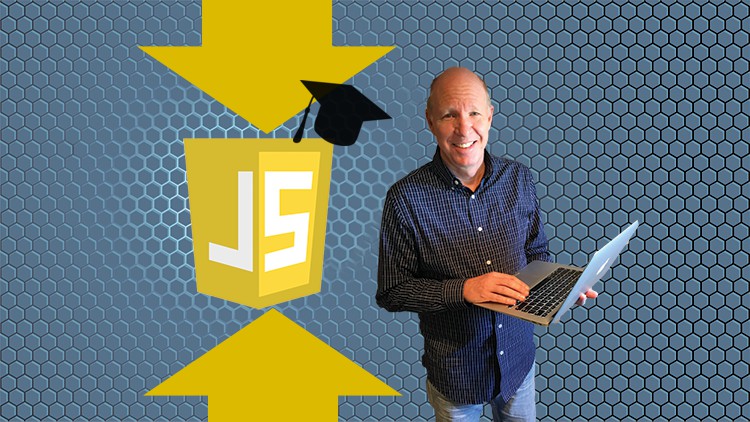
External Links May Contain Affiliate Links read more