JavaScript Mastery 2024: Zero to Expert with Interview Prep
- Description
- Curriculum
- FAQ
- Reviews
Welcome to the JavaScript Zero to Expert course of 2024 with Interview Preparation! This is a comprehensive, hands-on journey through JavaScript from its fundamentals to advanced concepts. Whether you’re new to programming or looking to deepen your understanding, this course is designed to cater to learners at all levels.
Why Take This Course?
In this course, we’ll cover everything from the basics of JavaScript syntax to advanced topics like object-oriented programming, asynchronous programming, and DOM manipulation. Plus, with our interview preparation series, you’ll be ready to ace JavaScript-related questions in job interviews.
With hands-on exercises, coding challenges, and real-world examples, you’ll get plenty of practice applying what you’ve learned. And with interview preparation videos, you’ll be ready to tackle JavaScript-related questions in job interviews.
Join this new course in 2024 and stay ahead of the curve. From beginners to experienced developers, this course is designed to accommodate all skill levels. With updated content and practical exercises, you’ll gain a comprehensive understanding of JavaScript that is relevant and applicable in today’s web development landscape. Get ready to elevate your coding skills and create dynamic, interactive web applications with JavaScript in 2024.
Here’s an overview of the topics covered in the course:
-
Introduction to JavaScript and setting up your development environment
-
Basics of programming in JavaScript, including variables, data types, and control structures
-
Working with arrays and objects, including manipulation and iteration
-
Functions, scope, and advanced function concepts like parameters and callbacks
-
Important array methods and other useful concepts like iterables, sets, and maps
-
Object-oriented JavaScript, including classes, inheritance, and prototypes
-
JavaScript execution, including execution context, hoisting, scope chain, and closures
-
DOM manipulation and event handling, including accessing and modifying elements, working with styles and attributes, and handling events
-
Asynchronous JavaScript, including working with timers, callbacks, and promises
-
Other important topics like error handling, working with APIs, and more
This course is suitable for beginners as well as experienced developers looking to deepen their understanding of JavaScript. Whether you’re looking to build websites, web applications, or backend services, JavaScript is an essential skill, and this course will provide you with the knowledge and skills you need to succeed.
-
4First Program in JavaScriptVideo lesson
-
5Introduction of var keywordVideo lesson
-
6Naming variables rules in JavaScriptVideo lesson
-
7Introduction of let and const keywordVideo lesson
-
8String Indexing in JavaScriptVideo lesson
-
9Useful String Methods in JavaScriptVideo lesson
-
10Concate and Template StringVideo lesson
-
11What is typeof Keyword?Video lesson
-
12BigInt, null and undefinedVideo lesson
-
13Boolean DatatypesVideo lesson
-
14What is Truthy and Falsy value?Video lesson
-
15If - else Conditional StatementVideo lesson
-
16Ternary OperatorVideo lesson
-
17What is nested-if ?Video lesson
-
18What is and(&&) or(||) operator ?Video lesson
-
19Understanding else if in JavaScriptVideo lesson
-
20Grade Determination using If, else if and else PracticeQuiz
-
21What is switch keyword?Video lesson
-
22Switch keywordQuiz
-
23What is For Loop ?Video lesson
-
24What is While Loop ?Video lesson
-
25What is Do-While Loop ?Video lesson
-
26What is continue and break keyword ?Video lesson
-
27Introduction of ArrayVideo lesson
-
28Array Methods: push, pop, shift, unshiftVideo lesson
-
29Array Methods: includes, indexOf, slice, join, concatVideo lesson
-
30Clone Array Methods: slice, spread, Array.from, concatVideo lesson
-
31Iterate Array: For and For of loopVideo lesson
-
32Iterate Array: For in and While loopVideo lesson
-
33What is Array Destructuring ?Video lesson
-
34Introduction of Object in JavaScriptVideo lesson
-
35How to add or remove values in objectVideo lesson
-
36Iterate Object: For of, For in, Object.keys()Video lesson
-
37What is spread operator in Javascript ? Arrays and ObjectsVideo lesson
-
38What is Object Destructuring and discuss different method of destructure ObjectVideo lesson
-
39Objects: Frequently asked interview questionsVideo lesson
-
40What is Functions in JavaScript ?Video lesson
-
41Functions: return keyword, arguments, parameters, default parametersVideo lesson
-
42Find Element in Array using a FunctionQuiz
-
43Exploring different ways to Define FunctionsVideo lesson
-
44Arrow Function PracticeVideo lesson
-
45What is Rest Parameters in Functions ?Video lesson
-
46What is Hoisting and Temporal Dead Zone?Video lesson
-
47How to define Functions inside Functions ?Video lesson
-
48What is Lexical Scope ?Video lesson
-
49What is Function scope and Block scope ?Video lesson
-
50What is Params Destructuring ?Video lesson
-
51What is Callback Functions ?Video lesson
-
52What is Functions return Functions ?Video lesson
-
53Array Operations: Map, Reduce, and FilterQuiz
-
54Introduction of .map() methodVideo lesson
-
55Introduction of .filter() methodVideo lesson
-
56Introduction of .reduce() methodVideo lesson
-
57Interview based Questions: When use map, filter and reduce methods ?Video lesson
-
58What is foreach method ?Video lesson
-
59What is .every() method ?Video lesson
-
60What is .some() method ?Video lesson
-
61Array Methods: .find(), .reverse(), .fill(), .splice()Video lesson
-
62Array Methods: .flat()Video lesson
-
63What is .sort() method in JavaScript ?Video lesson
-
69What is .this keyword ?Video lesson
-
70What is .call() and .apply() methods in JavaScript ?Video lesson
-
71What is .bind() method in JavaScript ?Video lesson
-
72.this : What is difference between Arrow function and Regular function ?Video lesson
-
73What is the difference between __proto__ and prototype in JavaScript ?Video lesson
-
74Introduction of Class in JavaScriptVideo lesson
-
75What is static method in JavaScript ?Video lesson
-
76What is Inheritance and Method Overriding in JavaScript ?Video lesson
-
77What is getter and setter methods in JavaScript ?Video lesson
-
78What is static properties in Class ?Video lesson
-
79How JavaScript works ?Video lesson
-
80What is Global Execution Context ?Video lesson
-
81What happen to function declaration ?Video lesson
-
82How Hoisting works ?Video lesson
-
83What happen with Function Expression in GEC ?Video lesson
-
84Are Let and Const Variables are Hoisted ?Video lesson
-
85What is Function Execution Context ?Video lesson
-
86What is Scope Chain in JavaScript ?Video lesson
-
87What is Clousers in JavaScript ?Video lesson
-
88Interview Based Questions : ClosuresVideo lesson
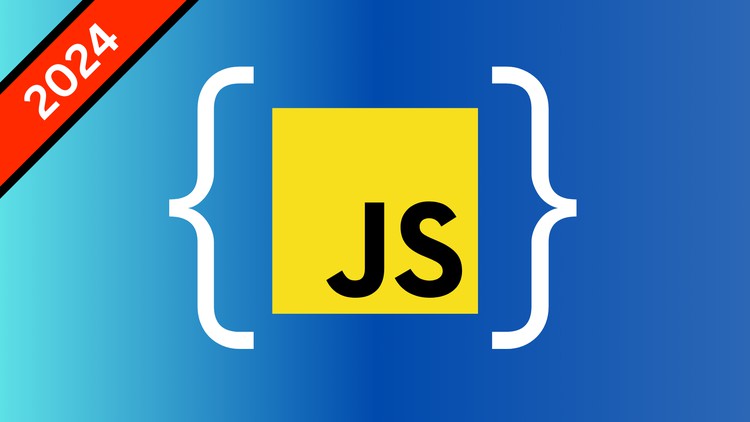
External Links May Contain Affiliate Links read more