Java Core Guide: Key Features, OOP, Collections & More
- Description
- Curriculum
- FAQ
- Reviews
Are you looking to learn Java Core from scratch? This course is designed for beginners who want to build a solid foundation in Java programming. Whether you are new to coding or want to strengthen your Java skills, this course provides clear explanations, hands-on coding examples, and useful interview preparation materials to help you succeed.Throughout the course, you will explore essential Java topics such as object-oriented programming (OOP), strings, Java collections, multithreading, and key Java features. Each topic is explained in a simple and structured way, making it easy to understand even if you have no prior programming experience.This course includes:Step-by-step explanations of Java Core concepts.Practical coding examples to reinforce your learning.Tests and quizzes to check your understanding.Interview preparation materials to help you land a Java-related job.Hands-on exercises to apply what you learn in real coding scenarios.By the end of this course, you will have a strong grasp of Java Core, enabling you to write Java programs confidently and prepare for technical interviews. All you need is a computer and basic computer skills-no prior coding experience required!Start your Java learning journey today and take the first step toward becoming a Java developer!
-
5VariablesVideo lesson
What are variables;
Key characteristics of variables;
Types of variables;
Variable Naming Rules
-
6Data Types (Primitives)Video lesson
Primitives
Suffixes for number types;
Explicit and implicit casting.
-
7Data types (Objects)Video lesson
-
8Operators in JavaVideo lesson
-
9If-else statementsVideo lesson
If-else statements
Ternary operator
-
10Switch statementsVideo lesson
Switch statements
-
11for loopsVideo lesson
for loops
-
12while loopsVideo lesson
while loops
do-while loops
-
13Autoboxing and UnboxingVideo lesson
Objects and primitives in Java
Autoboxing and Unboxing in Java
Primitives vs Wrapper Classes
-
14ArraysVideo lesson
-
15ExceptionsVideo lesson
Exceptions
Hierarchy of Exceptions in Java
Types of Exceptions
try-catch-finally block
-
16EnumVideo lesson
What is enum?
How to declare an enum
Using enums in switch statement
How to compare enums
Enum methods
Enums with methods and fields
Enums implementing interfaces
-
17SerializationVideo lesson
What is Serialization
Conditions for Serialization
serialVersionUID
Deserialization
Where Serialization can be used
Limitations of Serialization
-
18Java memory model, Garbage collectorVideo lesson
What is Java Memory Model
Primary memory areas
Java Memory Components
Garbage collector
-
19Java core final testQuiz
-
20Java core Interview questions and answersText lesson
-
21What is OOP?Video lesson
Agenda:
What is OOP?
Access modifiers in Java, examples, its role in OOP
Key components of OOP
Examples of OOP in real life
What is class?
Why do we need to use OOP?
Core principles of OOP
-
22InheritanceVideo lesson
Agenda:
What is Inheritance?
Why is Inheritance Needed?
Example of Inheritance
Drawbacks of Inheritance
-
23EncapsulationVideo lesson
Agenda:
Encapsulation definition
Key Aspects of Encapsulation
Take a look at an example
Benefits of Encapsulation
-
24PolymorphismVideo lesson
What is Polymorphism?
The goal of Polymorphism
Types of Polymprphism
Overloading vs Overriding
-
25Abstract classes and Interfaces, Abstraction principleVideo lesson
Definition of abstract class
Key Characteristics of an Abstract Class
What is an Interface?
Key Characteristics of an Interface
Peculiarities and differences of Abstract Classes and Interfaces
What is Abstraction in OOP?
Key idea of abstraction
Example of Abstraction
How is Abstraction Achieved in OOP
Anonymous class
Benefits of Abstraction
-
26Constructors, blocksVideo lesson
Constructors
Static blocks
Instance Initialization Blocks
Order of execution
Constructor overloading
super keyword
-
27GenericsVideo lesson
What is generics
Generic class
Generic method
Bounded type parameters
Wildcards
Erasure in Generics
-
28OOP final testQuiz
-
29OOP Interview questions and answersText lesson
-
34ArrayList vs LinkedListVideo lesson
ArrayList
LinkedList
-
35Equals and HashcodeVideo lesson
What is equals and hashcode methods
equals and hashcode contracts
Common mistakes to avoid
Best Practices for equals() and hashCode()
-
36HashMap and HashSetVideo lesson
HashMap
HashSet
-
37Comparator vs ComparableVideo lesson
Comparator
Comparable
When to use each
-
38TreeMap and TreeSetVideo lesson
TreeMap
TreeSet
-
39Explore TreeSetQuiz
-
40Iterator and IterableVideo lesson
Iterator key methods
ConcurrentModificationException
Why use an iterator
ListIterator
Iterable
-
41LinkedHashSetVideo lesson
What is LinkedHashSet
How does it work
Advantages of LinkedHashSet
-
42CopyOnWriteArrayListVideo lesson
CopyOnWriteArrayList
What is CopyOnWriteArrayList
Why is it Needed?
How Does It Work?
When to Use CopyOnWriteArrayList?
Drawbacks
-
43Java Collections final testQuiz
-
44Java Collections Interview QuestionsText lesson
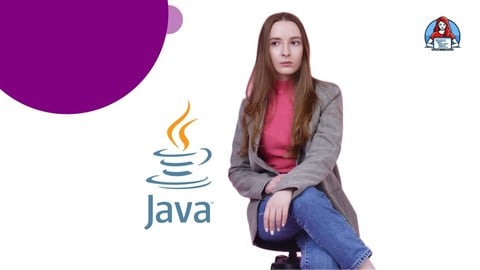
External Links May Contain Affiliate Links read more