Java Tutorial for Beginners : Complete Visual Learning
- Description
- Curriculum
- FAQ
- Reviews
Complete Java Tutorial for Beginners.
Java 2025: Learn Coding & How Code Runs with Visuals is not just another Java course—it’s a deep dive into programming from a RAM perspective, ensuring you gain 100% clarity on how Java executes your code in the backend.
Designed for absolute beginners, this course will help you build a solid foundation in Java programming, from the basics to advanced concepts. Whether you’re preparing for a Java certification, aiming for a software development career, or simply adding Java to your skill set, this course will guide you step by step.
Why Choose This Course?
Learn Java Like Never Before – Visualize how code executes in memory, ensuring 100% clarity.
No Prior Experience Needed – Start from scratch, no coding background required.
Hands-on Learning – Practical coding exercises with real-time memory tracking.
Certification Preparation – Get ready to ace your Java certification and impress potential employers.
Lifetime Access – Learn at your own pace and revisit lessons anytime.
What You’ll Learn:
Java Fundamentals – Master variables, data types, operators, and control flow with a clear understanding of how memory is allocated.
Object-Oriented Programming (OOP) – Learn about classes, objects, inheritance, polymorphism, and encapsulation, with real-time memory visualization.
Data Structures & Algorithms – Understand arrays, lists, maps, and sets while seeing how data is stored and manipulated in memory.
Exception Handling – Learn to write robust code by effectively managing errors and exceptions.
Multithreading – Explore concurrent programming and understand how multiple threads interact with memory.
Enroll Now and See How Java Works Behind the Scenes!
-
1Introduction to Java: History and Key FeaturesVideo lesson
In this tutorial will take you through the foundational aspects of Java, its history, and its key features. By the end of this session, you should have a clear understanding of what makes Java special and why it remains a popular choice for developers.
-
2Understanding JVM, JDK, and JREVideo lesson
In this session, we will explore the key components that make Java a robust and widely-used programming language: JVM (Java Virtual Machine), JDK (Java Development Kit), and JRE (Java Runtime Environment). These components are essential for developing and running Java applications efficiently.
-
3RAM and Numerical Data Types in JavaVideo lesson
In this session, we'll explore the concept of RAM (Random Access Memory) and its functionality in computer systems. RAM is a crucial component in computing, enabling quick access and processing of data by the CPU.
-
4Numerical Data TypesVideo lesson
In this lesson, we explore various numerical data types in Java, including byte, short, int, and long. We learn how to declare these data types, assign values to them, and understand their ranges and behaviors. Additionally, we discuss variable naming conventions and a concept called "looping" in data types.
-
5Primitive and Reference Data TypesVideo lesson
In this lesson, we will see:
Primitive Data Types: Basic data types in Java are categorized as numerical (integers and real numbers) and non-numerical (characters and boolean).
Reference Data Types: These include objects and arrays, which are not covered in this session.
-
6OperatorsVideo lesson
In this lesson, we will see:
Purpose of Operators: Operators are special symbols that perform operations on variables and values.
Types of Operators: Java provides various operators grouped into different categories based on their functionality.
-
7Conditional StatementsVideo lesson
In this lesson, we will see:
Conditional statements in Java allow us to execute different blocks of code based on specific conditions. These are fundamental for controlling the flow of a program and making decisions.
-
8If-Else StatementsVideo lesson
In this session, we will delve into practical examples to understand how to use if-else statements effectively in Java. We will cover the concepts of statement precedence and condition precedence through a series of examples, including how to determine if a given year is a leap year.
-
9Loops in JavaVideo lesson
In this lesson, we will see:
Loops and Their Usage
-
10Exploration of the for LoopVideo lesson
In this lesson, we will see: while loop, do-while loop and for loop.
-
11Quiz 1 - Java BasicsQuiz
Core Java Concepts
-
12Solving Number-Based ProblemsVideo lesson
In this session, we covered:
Understanding number-based problems.
Using loops to solve number-based problems.
Writing efficient code to handle these problems.
Analyzing the concept of perfect numbers.
Practicing the identification of prime numbers.
-
13Prime Number Algorithm and Range AnalysisVideo lesson
In this tutorial, we will delve into prime numbers, understand how to check if a number is prime, and extend this concept to identify all prime numbers within a given range. This guide will break down the concepts and code step-by-step, making it easy to follow and implement.
-
14Armstrong Numbers and Advanced Number CrunchingVideo lesson
This study guide will cover the following key concepts and coding techniques for understanding and implementing Armstrong numbers and number crunching in Java. We'll break down the process, code, and logic in a detailed and easy-to-understand manner.
-
15Introduction to Pattern ProgrammingVideo lesson
In this lesson, we will see: Patterns in Java involve printing symbols or characters in a specific order, creating visually recognizable structures. These exercises are commonly used in coding interviews to assess logical thinking and coding skills.
-
16Advanced Techniques in Pattern ProgrammingVideo lesson
Welcome to the next session on advanced pattern programming. Building on the basics we've covered, we'll now dive into more complex patterns, including number-based and string-based patterns. These patterns are crucial for enhancing problem-solving skills and are often featured in technical interviews.
-
17Handling Complex Pattern ProgramsVideo lesson
In this lesson, we will see: Patterns Programs
-
18Introduction to ArraysVideo lesson
In this lesson, we will see: Array
-
19Array Insertion TechniquesVideo lesson
In this lesson, we will see: Array Insertion.
-
20Array Deletion MethodsVideo lesson
In this lesson, we will see: Array Deletion.
-
21Array Searching and Sorting AlgorithmsVideo lesson
In this tutorial session, we'll delve into the fundamental concepts of searching and sorting arrays in Java. These operations are essential for organizing and retrieving data efficiently in programming.
-
22Quiz 2: Array FundamentalsQuiz
-
23Practical Programs in ArraysVideo lesson
In this session, we will learn how to find the smallest and second smallest elements in an array efficiently. This problem is crucial for coding interviews and exams, where time complexity constraints are common. We will discuss:
Basic concept and naive solution.
Efficient solution with time complexity considerations.
-
24Sliding Window Concept in ArraysVideo lesson
In this lesson, we will see: Sliding Window Concept
-
25Array Rotation ProblemVideo lesson
In this session, we delve into solving an array rotation problem frequently encountered in product-based company interviews. The goal is to rotate an array to the right by a given number of positions. We'll explore a detailed step-by-step approach to understand the problem, develop a solution, and optimize the code.
-
26Minimizing Swaps in ArraysVideo lesson
In this tutorial, we'll cover the problem of finding the minimum number of swaps required to rearrange an array such that all elements on the left of a given key element are less than the key and all elements on the right are greater than the key. This problem is useful for understanding array manipulation and optimizing operations within constraints.
-
27Multi-Dimensional ArraysVideo lesson
In this session, we will learn about multi-dimensional arrays. A multi-dimensional array can be visualized as arrays within arrays, where a one-dimensional array is a simple list, a two-dimensional array can be seen as a grid or a matrix, and higher-dimensional arrays extend this concept further.
-
28Common Interview Problems: ArraysVideo lesson
In coding interviews, one common type of problem involves multi-dimensional arrays, particularly the spiral matrix problem. Understanding how to navigate and manipulate these arrays is crucial. This guide will help you understand how to approach and solve spiral matrix problems, a skill that will serve you well in technical interviews.
-
29Array ManipulationVideo lesson
In this lesson, we will see: Introduction to Mutable and Immutable Strings
-
30Mutable and Immutable StringsVideo lesson
In this lesson, we will see: Strings
-
31String ManipulationVideo lesson
we will delve into string tokenizer, a feature that adds functionality not available in both strings and string buffers.
-
32String TokenizerVideo lesson
In this session, we will cover:
Understanding ASCII values
Character-based string manipulations
Writing code to alternate between upper and lower case characters in a string
Practical coding examples
-
33Character-Based String ManipulationsVideo lesson
In this session, we'll cover how to handle word-based string questions in Java. Previously, we dealt with character-based manipulations where we alternated between uppercase and lowercase characters. This time, we’ll focus on reversing words in a string and alternately converting them to uppercase and lowercase.
-
34Word-Based String ProgrammingVideo lesson
This session covers the concept of number-based strings, where characters and numbers are intertwined in a single string. The goal is to extract and manipulate these components separately.
-
35Quiz 3: String HandlingQuiz
-
36Introduction to Object-Oriented Programming (OOP)Video lesson
In this lesson, we will see: OOPs
-
37Creating and Using Classes and ObjectsVideo lesson
In this tutorial, we will explore how to create and use classes and objects in Java. We will define a class Dog with properties and methods, and understand the concepts of static methods and variables. This session is a hands-on guide to understanding basic Object-Oriented Programming (OOP) concepts in Java.
-
38Constructors and EncapsulationVideo lesson
In this session, we will explore two fundamental concepts in Java: Constructors and Encapsulation. These concepts are crucial for creating robust and maintainable code in Java.
-
39Understanding PolymorphismVideo lesson
In this session, we will explore the concept of polymorphism in Java, specifically focusing on method overloading. Polymorphism allows us to perform a single action in different ways, enhancing flexibility and reusability in code. We will understand method overloading by creating multiple methods with the same name but different parameters.
-
40Inheritance and Method OverridingVideo lesson
In this lesson, we will see: Inheritance and Method Overriding
-
41Abstract Classes and InterfacesVideo lesson
In this lesson, we will see: Abstract Classes and Interfaces
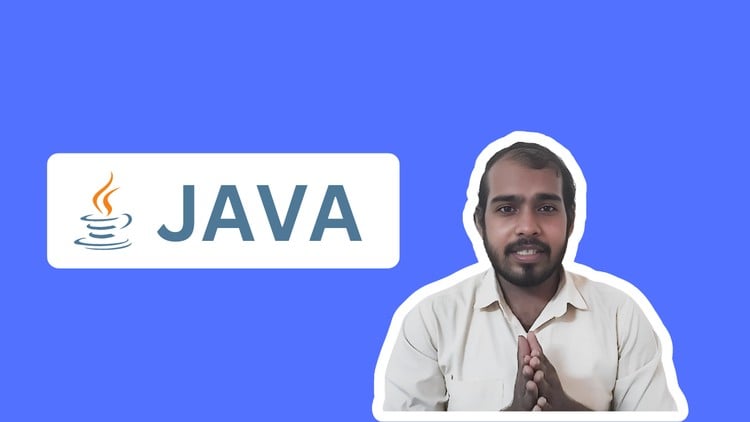
External Links May Contain Affiliate Links read more