iOS with Swift & Firebase
- Description
- Curriculum
- FAQ
- Reviews
Throughout this course, we will develop a complete iOS application using XCode and Swift programming language. In this course, we will develop a shopping list application with both local and remote databases. During the development, we will discuss topics such as interface design, databases and data structures as well as user authentication through Firebase MBaaS.
This course is useful for a variety of users. It can help if you wish to strengthen your development understanding with XCode or if you want to learn more about data structures, reading and writing local and remote databases.
-
1Introduction to This CourseVideo lesson
In this lesson, we will look at the course ahead of us. We will explore the different aspects of the course and what we are about to develop.
-
2Starting a New Project and Testing SimulatorVideo lesson
In this lesson, we will start a new app in XCode and test our simulator.
-
3Designing Our Lists StoryboardVideo lesson
In this lesson, we design our outlets and also name them as well the name for our table view cell
-
4Connecting Our Storyboard Outlets to the CodeVideo lesson
In this lesson, we will add a new View Controller through storyboard called ListsViewController. We'll also connect the data source and delegate for our table view in storyboard and add the necessary protocols. We should make a button outlet for the profile button.
-
5Table View Data SourceVideo lessonIn this lesson, we will add the only two absolutely necessary methods of our data source, we will also test our data source.
-
6Understanding Our Data StructureVideo lessonIn this non-programming lesson, we will look at how our data is structured.
-
7Adding Item ClassVideo lessonIn this lesson, we will add our Item Class.
-
8Adding User ClassVideo lesson
In this lesson, we will add our User Class.
-
9Adding Grocery List ClassVideo lessonIn this lesson, we will add our List Class.
-
10Adding App Data ClassVideo lesson
In this lesson, we will add our App Data class's starting elements. We would need to introduce and initialize our Current Lists as well as a dummy current user. We will also add an override init for future use
-
11Preparing First ListVideo lesson
In this lesson, we will add our first list, we will not however write it to disk as we still don't have that function.
-
12Testing Out the Initial ListVideo lesson
In this lesson, we want to access the newly created initial list.
-
13Adding a New ListVideo lessonBy now, we have a list view interface where we can view our basic starter lists. Now let's try and add a new list. In here, once everything work, we will face a new issue and that is our app will not maintain its data between two sessions of the application. We will address that in our next section.
-
14Understanding DataVideo lesson
In this lesson, we will have a quick look at why we have to record our data on the disk and what component we should add to do so.
-
15Conforming User Class to NS CoderVideo lesson
We need to conform our custom class to NSCoder to be able to save it to disk using plist file.
-
16Convert MethodsVideo lesson
In this lesson we will add a convert class to convert between bools and dates and strings.
-
17Conforming Item and Grocery Class to NS CoderVideo lessonWe need to conform our custom class to NSCoder to be able to save it to disk using plist file.
-
18Read Write Data MethodsVideo lesson
In this lesson, we will add a new method to our app data where all of our lists get written to the disk using a json serialization. To write data, we will also introduce a new OfflineLst.
-
19Reading Data Method Part 1Video lessonIn this lesson, we will adjust our list view to properly read and write data to the disk. We will also make sure if we make a new list, it gets written to the disk.
-
20Deleting a List by DraggingVideo lesson
In this lesson, we will learn how to delete a list by dragging it.
-
21Items InterfaceVideo lesson
In this lesson, we head back to our storyboard and design our items interface.
-
22Items View ControllerVideo lessonIn this lesson, we add a new view controller and connect it to our storyboard and connect the different outlets to their functions and outlets.
-
23Segue to the Item View ControllerVideo lesson
In this lesson, we will code the navigation to and back from Items View Controller.
-
24Cur ListVideo lessonIn this lesson, we will introduce our curList which is the list that we have clicked on in the Lists Activity. We will pass an argument through our PrepareForSgue.
-
25Items Table Data SourceVideo lessonIn this lesson, we will add the data source and delegate connections through this storyboard and also add the necessary protocols and the two required methods of number of rows and get cell.
-
26Clicking on an ItemVideo lessonIn this lesson, we change the status of an item by clicking on it, we also write our data to disk after doing that.
-
27Dragging to Delete an ItemVideo lesson
In the previous lesson, once we set everything correctly to delete and item, we realize that the Lists View Controller doesn't update its table view properly. That we will take care of in this lesson.
-
28Adding a New ItemVideo lessonIn this lesson, we will learn how to add an item by pressing the done button on the text field.
-
29Understanding What HappenedVideo lesson
In this lesson, we will look back at everything we have done so far and we try to make sense of everything we have done so far and how we could build on those.
-
30Introduction to BackendVideo lesson
In this lesson, we will look at our backend data and why we would need them.
-
31Firebase ConsoleVideo lesson
Once we install the pods based on Firebase, we will re-open the project through the workspace and add the google plist
-
32Setting Firebase RulesVideo lessonOnce we install the pods based on Firebase, we will re-open the project through the work-space.
-
33Initializing Firebase in AppDataVideo lesson
In this lesson, we write the code for our Firebase connection as well as our new OnlineLST
-
34Alert Show MethodVideo lessonIn this lesson, we will add a new method to show messages to user.
-
35Testing Out FirebaseVideo lessonWe will do a quick test on Firebase in this lesson to make sure it works properly.
-
36How to Use the AssetsVideo lesson
In this lesson we will have a quick look at our assets and how we could use them.
-
37Profile ActionVideo lessonIn this lesson, we will learn how to add the various profile actions to our login button.
-
38Register Alert ViewVideo lesson
In this lesson, we will add an alert dialog for when the register button is pressed.
-
39Register MethodVideo lesson
In this lesson, we will learn how to actually register our user on Firebase back-end.
-
40Set Local UserVideo lesson
In this lesson and after registration, we will discuss how to change the local user.
-
41Save User Main Values on CloudVideo lesson
Once user is created, we should write its main data to our backend.
-
42Save All User Lists OnlineVideo lessonIn this lesson, we will learn how to save an entire list to our online back-end.
-
43Login Alert ViewVideo lessonIn this lesson, we will write the alert view for our login menu. This code is little too long and also trivial, so we will use a little cheat in here,
-
44Login MethodVideo lesson
In this lesson we will use our Authentication to login our user to Firebase back-end.
-
45Reading Data Method Part 2Video lesson
In this lesson and after logging in, we continue with reading data.
-
46Reading Online DataVideo lessonIn this lesson we introduce the OnlineLST array and we also write the code to read the online lists in our App Data.
-
47Compare Lists MethodVideo lesson
In this lesson, we will look at the course ahead of us. We will explore the different aspects of the course and what we are about to develop.
-
48Reading Data Method Part 3Video lesson
In this lesson, we will start a new app in XCode and test our simulator.
-
49Setting Profile ButtonVideo lessonThrough setting our profile button, we can give our user a little feedback on whether they are online or offline.
-
50New List on CloudVideo lesson
In this lesson, we will learn how to add a new list on cloud.
-
51Delete List on CloudVideo lesson
In this lesson, we will learn how to delete a list from cloud.
-
52Save a New Item on CloudVideo lesson
In this lesson, we will learn how to add an item on cloud.
-
53Change Item Status on CloudVideo lesson
In this lesson, we will re-write our items once they are tapped on.
-
54Delete Item on CloudVideo lessonIn this lesson, we will learn how to delete an item.
-
55Logging Out From CloudVideo lessonIn this lesson, we will code our log out method.
-
56Recap of Online DataVideo lessonThis is also a good time to test all of our login, register methods once again. In here, we realize that we need to call our readData once again at the end of the register method. We will also test our application by manually adding an item to the cloud.
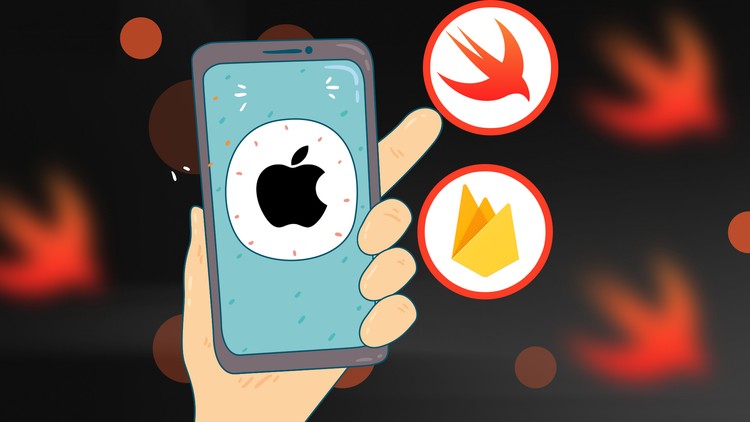
External Links May Contain Affiliate Links read more