Create a Complete Grid-Based Puzzle Game in Godot 4 with C#
- Description
- Curriculum
- FAQ
- Reviews
This course will cover everything you need to know about creating a small 2D grid-based puzzle game from start to finish in the Godot Engine 4.3+ using C# scripting. This course covers many aspects of creating a grid-based puzzle game including:
-
Building placement on a grid with variable tile sizes
-
Querying grid state using LINQ with C# data structures like HashSets and Dictionaries
-
Creating levels using the new Godot 4.3 TileMapLayer, including multi-level maps with y-sorting, animated tiles, and custom tile data
-
Saving and loading level completion progress
-
Implementing audio including building placement and destruction effects and music
This list is not exhaustive – please see the course outline for a glimpse into the topics that are covered.
The goal of this course is to show you how to take an empty project and turn it into a small, complete game. In doing so, this course will give you a robust exposure to many aspects of the Godot engine from C# scripting, to input handling, to tile maps, to saving and loading data, to audio, and more. By the time you complete this course, you will feel comfortable working on your own projects in Godot 4.3+ and C#. You will walk away from this course with a solid foundational understanding of making games that are not only functional but also fun.
This course will help you greatly if:
-
You are ready to dive into a crash course for Godot 4.3+ with C#
-
You are comfortable in Unity and want to transfer your C# scripting skills to Godot
-
You have some game development knowledge and want to learn Godot
-
You have some programming knowledge and want to make games
-
You struggle to complete games and want to start and finish a project
Please note that this course is focused purely on the start-to-finish process of making a grid-based puzzle game. With that goal in mind, there is no time spent explaining fundamental programming concepts of statically typed languages. Familiarity with programming and statically typed languages is strongly recommended before taking this course.
Several lessons are available to for you to preview for free. Please take a look at those videos before enrolling to determine if the pace of this course is right for you!
-
8Basic Grid Operations and TileMapLayer IntroductionVideo lesson
-
9Using a HashSet to Mark Occupied TilesVideo lesson
-
10Minor Code CleanupVideo lesson
-
11Adding the Asset Pack and Tower ArtVideo lesson
-
12Introduction to AutotilingVideo lesson
-
13Refactoring Code Into a GridManagerVideo lesson
-
14Defining and Using TileSet Custom DataVideo lesson
-
15Adding a Base BuildingVideo lesson
-
16Using Autoloads and Custom Signals for Grid State UpdatingVideo lesson
-
17Using Grid State for More Sophisticated Building RulesVideo lesson
-
18Highlighting Expandable Building AreaVideo lesson
-
19Adding Animated Tree TilesVideo lesson
-
20Accounting for Trees in GridManagerVideo lesson
-
21Applying Y-Sorting for Proper Draw OrderVideo lesson
-
22Adding Village BuildingsVideo lesson
-
23Introduction to Custom ResourcesVideo lesson
-
24Highlighting Resource TilesVideo lesson
-
25Tracking Collected Resource TilesVideo lesson
-
26Introduction to Control NodesVideo lesson
-
27Streamlining Building PlacementVideo lesson
-
28Creating a Building ManagerVideo lesson
-
29Creating a Building Placement GhostVideo lesson
-
30Indicating Whether Building Ghost is ValidVideo lesson
-
31Cancelling Building PlacementVideo lesson
-
32Refactoring Building Manager to Support StatesVideo lesson
-
33Refunding Resources and Updating Grid on Building DestructionVideo lesson
-
34Adding a Win ConditionVideo lesson
-
35Transforming the Main Scene Into a Base Level SceneVideo lesson
-
36Adding Terrains to the TilesetVideo lesson
-
37Adding Water to the TilesetVideo lesson
-
38Adding Shadows to the TilesetVideo lesson
-
39Designing Your First LevelVideo lesson
-
40Adding Camera PanningVideo lesson
-
41Fixing Tileset, Centering the Camera, and Adding Configurable Starting ResourcesVideo lesson
-
42Fixing Shadow Tiles Invalidating Buildable TilesVideo lesson
-
43Making UI Buttons Follow CameraVideo lesson
-
44Making Buildings Occupy Multiple TilesVideo lesson
-
45Adding Base Building Art and Preventing DeletionVideo lesson
-
46Allowing Building Deletion by Clicking Into Occupied AreaVideo lesson
-
47Preventing Building Placement on Differing Elevation LayersVideo lesson
-
48Introduction to Extension MethodsVideo lesson
-
49Making Building Radius CircularVideo lesson
-
50Introduction to UI ThemingVideo lesson
-
51Theme Files and Theme VariationsVideo lesson
-
52Styling Building Selection UIVideo lesson
-
53Fonts and Button StylingVideo lesson
-
54Improving the Design of Building SectionsVideo lesson
-
55Displaying Resource Count in Game UIVideo lesson
-
56Building a Main Menu Skeleton UIVideo lesson
-
57Building a Framework for Changing LevelsVideo lesson
-
58Creating a Level Complete ScreenVideo lesson
-
59Moving to the Next Level from the Level Complete ScreenVideo lesson
-
60Designing More LevelsVideo lesson
-
61Addressing Some Errors and IssuesVideo lesson
-
62Creating a Level Select ScreenVideo lesson
-
63Moving Level Definitions to Custom ResourcesVideo lesson
-
64Showing Levels on the Level Select ScreenVideo lesson
-
65Integrating the Level Select Screen With the Main MenuVideo lesson
-
66Improving the Look of Building PlacementVideo lesson
-
67Introduction to Animation PlayerVideo lesson
-
68Using Tweens to Animate Building PositionVideo lesson
-
69Centering Building Placement on Mouse PositionVideo lesson
-
70Animating Building PlacementVideo lesson
-
71Call Building Placement Animation on DemandVideo lesson
-
72Building Destruction AnimationVideo lesson
-
73Adding a Mask to Building DestructionVideo lesson
-
74Fixing Destruction-Related BugsVideo lesson
-
75Building Placement ParticlesVideo lesson
-
76Building Destruction ParticlesVideo lesson
-
77Creating a Goblin CampVideo lesson
-
78Making the Goblin Camp FunctionalVideo lesson
-
79Creating a Barracks BuildingVideo lesson
-
80Allowing Barracks to Be Placed in Goblin CampsVideo lesson
-
81Showing Barracks Attack AreaVideo lesson
-
82Making the Barracks Destroy Goblin CampsVideo lesson
-
83Handling Goblin Camp Restoration on Barracks DestroyVideo lesson
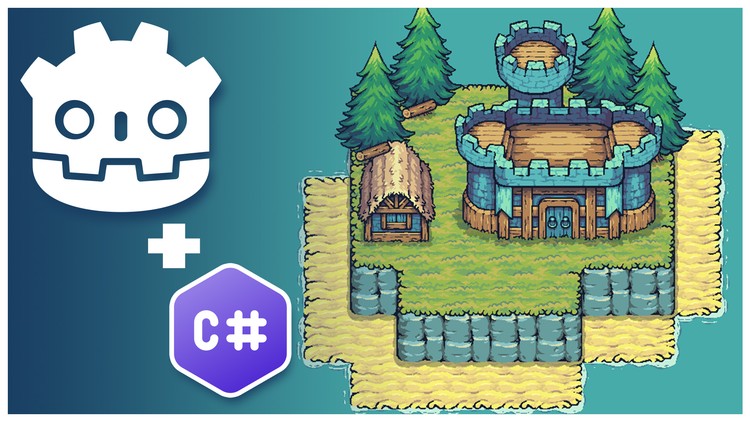
External Links May Contain Affiliate Links read more