Advanced Flutter: MVVM with Provider, Riverpod | BLoC
- Description
- Curriculum
- FAQ
- Reviews
Dive into the depths of Flutter’s MVVM architecture with different state managements in this comprehensive course designed for intermediate to advanced and expert developers. Learn how to build a dynamic movie app that interacts with a REST API, manages local favorites, and incorporates genre mapping for enhanced functionality with Dark and Light themes. This course will guide you through the journey of implementing MVVM with different state management strategies—setState, Provider, Riverpod, and BLoC—each covered in dedicated sections to provide you with a robust understanding of each approach.
Prerequisites:
-
Basic knowledge of programming
-
Basic familiarity with Flutter Widgets and Dart
-
Basic understanding of REST APIs is a plus, but we cover this in detail
-
Enthusiasm for learning state management in-depth
-
Macbook or Windows to develop
-
Any preferred IDE (such as Android Studio or IntelliJ IDEA), or a preferred text editor (like VS Code)—just not Microsoft Word!
Recording Equipment:
-
Video Editing Software: Camtasia 2023
-
Microphone: Blue Yeti X
This setup ensures that all course videos are clear and professionally produced.
What You Will Learn:
-
Implementing MVVM architecture in Flutter for scalable app development
-
Connecting to a REST API to fetch and display movie data
-
Local data management for user favorites
-
Advanced state management techniques with setState, Provider, Riverpod, and BLoC
-
Theme management in Flutter apps for dynamic light and dark modes
Course Structure:
-
Duration: Approximately 12 hours of content
-
MVVM Section, UI section, API section, and different sections for the state managements
-
Lecture length: each lecture is around 10 min
What to Expect After This Course:
-
Proficiency in using MVVM architecture for complex Flutter applications
-
Deep understanding of various state management techniques and when to use them
-
Skills to design and manage both themes and local databases in Flutter
-
Enhanced capability to handle real-world Flutter projects that require advanced state management and API integration
Limitations and Notes:
-
The course code works for all platforms supported by Flutter
-
The course project is designed for portrait mode responsiveness.
Resources:
-
Complete source code for the movie app for each state management explained in the course (setState, Provider, Riverpod, BLoC)
-
Step-by-step guides on implementing each feature
-
Access to slides and additional reading materials
-
1IntroductionVideo lesson
-
2Course for the best priceVideo lesson
-
3GitHub & Discord & Youtube & FBVideo lesson
-
4What will you learn in this course?Video lesson
-
5Get the most of this course and how to use the attached resourcesVideo lesson
-
6App Demo + More About this courseVideo lesson
-
7Course and Udemy important hintsVideo lesson
-
8Source code on Github + Attached lecturesVideo lesson
-
12Add the required packages throughout the courseVideo lesson
-
13Create The App ThemeVideo lesson
-
14Start Creating the movies screen and the cached network imageVideo lesson
-
15Start implementing the movies widget.mp4Video lesson
-
16Implement the favorite btn widgetVideo lesson
-
17Create the geners list dynamic widgetVideo lesson
-
18Implement the favorites screenVideo lesson
-
19Implement the Movie Details ScreenVideo lesson
-
20Implement the Error Widget and the Splash ScreenVideo lesson
-
25Create an account on Movies DB and request an API KeyVideo lesson
-
26API Documentation OverviewVideo lesson
-
27Create the Movie ModelVideo lesson
-
28Explain why we need to use the genre api and do the mappingVideo lesson
-
29Create the API Constants ClassVideo lesson
-
30Create a secured envirement for the API KEYsVideo lesson
-
31Start integrating the API in our code and send requestsVideo lesson
-
32Override the toString Function in the MoviesModelVideo lesson
-
33Implement the Movie Genres Model and the API CallsVideo lesson
-
34Create the Repository classVideo lesson
-
35Advanced Local State Management vs Global State ManagementVideo lesson
-
36Fetch the movies with pagination using the setState state managementVideo lesson
-
37Display the correct movie details on the screenVideo lesson
-
38Add animation for the imageVideo lesson
-
39Fetch the genres in the splash screenVideo lesson
-
40Display the correct genres on the screen and do the mappingVideo lesson
-
41Test the retry button in the Error handlingVideo lesson
-
42Implement the favorite btn with the setState management and display why it isVideo lesson
-
43Fix the average number formattingVideo lesson
-
45Explain the Provider State Management in theoryVideo lesson
-
46Start implementing the Theme State management with providerVideo lesson
-
47More about the Consumer Widget in ProviderVideo lesson
-
48Load the last saved themeVideo lesson
-
49Implement the Movies ProviderVideo lesson
-
50Implement the splash screen using the provider state managementVideo lesson
-
51Recap how our App code is connected together using MVVMVideo lesson
-
52Test the error handling in the Splash ScreenVideo lesson
-
53Implement the pagination using the provider state managementVideo lesson
-
54Display the movies information on the screen using a new way from the ProviderVideo lesson
-
55Display the correct genres on the screenVideo lesson
-
56Implement the Favorites ProviderVideo lesson
-
57Allow the user to add and remove from his favoritesVideo lesson
-
58Load the favorite moviesVideo lesson
-
59Display the favorites in the Favorites ScreenVideo lesson
-
60Quiz - Provider State ManagementQuiz
Quiz Related to the Provider State Management
-
61Get Started with the Riverpod state managementVideo lesson
-
62Create the theme providerVideo lesson
-
63Finalize the theme provider using RiverpodVideo lesson
-
64Recap on how we implemented the theme provider using the riverpod and how thiVideo lesson
-
65Introduce the Consumer widget in RiverpodVideo lesson
-
66Start Implementing the movies stateVideo lesson
-
67Create the copyWith Function to be able to update the movies stateVideo lesson
-
68Create the movies providerVideo lesson
-
69Implement the Splash Screen using Future BuilderVideo lesson
-
70Implement the Splash Screen using async notifierVideo lesson
-
71Test the error handling in the Splash Screen - RiverpodVideo lesson
-
72Fix the setState() or markNeedsBuild() called during build.Video lesson
-
73autoDispose & ref.keepAlive()Video lesson
-
74Fetch movies and implement the pagination in the Movies ScreenVideo lesson
-
75Display the correct movie data on the screen using the RiverpodVideo lesson
-
76Fix the duplicated movies bugVideo lesson
-
77Implement the favorites state and providerVideo lesson
-
78Allow the user to add and remove from his favoritesVideo lesson
-
79Load the favorites on the App startVideo lesson
-
80Display the correct favs on the screenVideo lesson
-
81Explain Stream ProviderVideo lesson
-
82Explain the logging observer using the RiverpodVideo lesson
-
83Explain the ConsumerStfulWidget - dont skipVideo lesson
-
84Display the correct movie genres on the screenVideo lesson
-
85Riverpod QuizQuiz
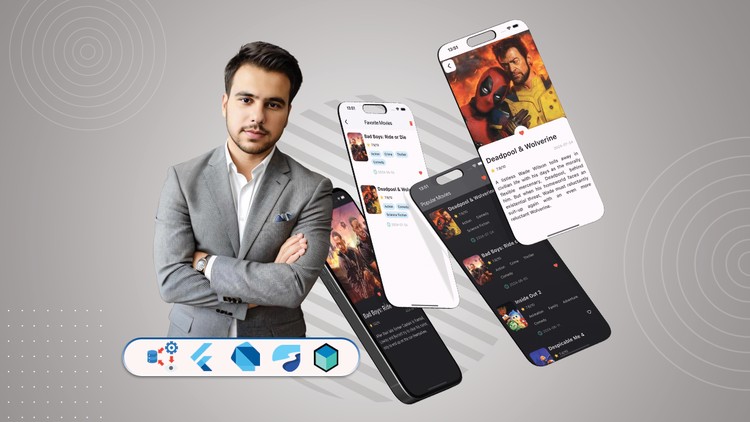
External Links May Contain Affiliate Links read more