JavaScript Fundamentals (2025): From Basics to Brilliance
- Description
- Curriculum
- FAQ
- Reviews
Welcome to “JavaScript Fundamentals (2024): From Basics to Brilliance” – your definitive guide to mastering the world’s most popular programming language.
This comprehensive course is designed for learners of all levels seeking to gain proficiency in JavaScript and web development. Whether you’re an absolute beginner or a developer looking to brush up on your skills, this course offers a deep dive into the fundamentals of JavaScript along with the latest ES6 features.
The course begins with an introduction to JavaScript, setting up the environment, and understanding what JavaScript is all about. We then delve into JavaScript basics, covering variables, data types, operators, and control structures. This foundational knowledge will equip you with the understanding needed to start writing your own scripts.
Next, we move into a detailed exploration of functions in JavaScript, where you’ll grasp the concepts of function expressions and declarations. This knowledge is pivotal in writing cleaner and more efficient code.
We then transition into modern JavaScript with a thorough examination of ES6 features. You’ll learn about ‘Let’ and ‘Const’, arrow functions, template literals, destructuring assignment, spread and rest operators, default parameters, and modules and imports. These ES6 features will enable you to write more concise and powerful code.
The course continues with a deep dive into Objects in JavaScript. You’ll understand what objects are, how to create and access them, as well as exploring arrays and array methods. This knowledge is crucial for data handling in JavaScript.
Finally, you’ll apply your newfound knowledge in a mini project where you’ll build a basic calculator. This hands-on experience will solidify your learning and give you the confidence to tackle more complex projects.
With “JavaScript Fundamentals (2024): From Basics to Brilliance”, you’ll not only learn JavaScript, but you’ll understand how it works. This course is your stepping stone towards becoming a proficient JavaScript developer capable of building dynamic and interactive websites.
No matter your previous coding experience, this course is designed to make JavaScript accessible and easy to understand. By the end of the course, you will have a strong understanding of JavaScript, paving the way for learning advanced front-end frameworks like React, Vue, Angular, or Svelte.
Start your JavaScript journey today and join the community of developers who have chosen “JavaScript Fundamentals (2024): From Basics to Brilliance” as their path
-
1What is JavaScript?Video lesson
This comprehensive session is designed to familiarize learners with the core concepts of JavaScript programming. Starting with variables and data types, students will understand how to store and manipulate data. The use of operators for performing arithmetic, logical, and comparison operations will be explained, setting the stage for implementing control structures such as loops and conditional statements. This foundational knowledge is crucial for building the logic behind web interactivity, enabling learners to write effective and efficient JavaScript code.
-
2JavaScript BasicsQuiz
-
3Setting up the environmentVideo lesson
The session progresses to guide learners through setting up their development environment, including choosing an IDE (Integrated Development Environment) and executing their first script. This foundational step is critical for all aspiring web developers, providing the necessary tools and context for writing and testing JavaScript code.
-
4JavaScript in the Browser vs. Node.jsVideo lesson
Discover the distinct environments where JavaScript thrives: the browser and Node.js. In the browser, JavaScript powers dynamic web pages by manipulating the DOM, handling user events, and interacting with web APIs like fetch for network requests. It enhances user experiences with real-time updates and interactivity.
On the other hand, Node.js brings JavaScript to the server side, allowing for scalable network applications and server-side scripting. Node.js excels in handling asynchronous operations with its event-driven architecture, perfect for building APIs, handling file systems, and managing databases.
Understand the differences in APIs, execution contexts, and use cases between client-side and server-side JavaScript, and learn how to leverage the strengths of both environments to create robust, full-stack applications. This topic equips you with the knowledge to choose the right tools and approaches for your web development projects.
-
5JavaScript History and EvolutionVideo lesson
Trace the fascinating journey of JavaScript from its inception to its current status as a cornerstone of modern web development. Learn how JavaScript was created by Brendan Eich in 1995 at Netscape, initially designed to add interactivity to web pages. Follow its rapid growth from a simple scripting language to a powerful, full-fledged programming language.
Understand the key milestones, such as the standardization by ECMAScript, the introduction of AJAX which revolutionized web applications, and the significant updates with ES6 (ECMAScript 2015) that brought arrow functions, classes, modules, and more. Explore subsequent iterations and enhancements in ES7, ES8, and beyond, introducing features like async/await, new data structures, and improvements in performance and security.
This topic provides a comprehensive overview of JavaScript's evolution, highlighting how it has adapted to changing technological landscapes and developer needs, becoming an essential tool for creating dynamic, interactive web applications.
-
6Variables and data typesVideo lesson
Students will start by learning about variables and the various data types available in JavaScript, such as strings, numbers, and booleans. Understanding these concepts is essential for data manipulation and storage in web applications.
-
7Navigating Variables and Data Types in JavaScriptQuiz
-
8- OperatorsVideo lesson
The course then explores operators for performing arithmetic, comparison, and logical operations, forming the basis of decision-making in code.
-
9Cracking the Code of Operators in JavaScriptQuiz
-
10- Control structuresVideo lesson
Control structures, including loops and conditional statements, are introduced as methods to control the flow of execution in programs, enabling developers to write more efficient, dynamic, and interactive web features.
-
11Mastering Control Structures in JavaScriptQuiz
-
12Data Type ConversionVideo lesson
Master the essential concept of data type conversion in JavaScript, a crucial skill for ensuring data integrity and avoiding runtime errors. Learn how to convert data between different types, such as strings, numbers, booleans, and objects, to facilitate seamless operations in your code.
Understand implicit and explicit conversions. Explore implicit conversion, also known as type coercion, where JavaScript automatically converts data types during operations, like concatenating a number and a string. Delve into explicit conversion methods, allowing precise control over data types, such as using Number(), String(), Boolean(), parseInt(), and parseFloat() functions.
Recognize common pitfalls and unexpected behaviors in type conversions, and learn best practices to handle them effectively. This topic ensures you can confidently manage and manipulate different data types, enhancing your ability to write robust and error-free JavaScript code.
-
13Type CoercionVideo lesson
Explore type coercion in JavaScript, an automatic conversion process where the language converts data types to enable operations between mismatched types. Understand how JavaScript implicitly changes types in expressions and comparisons, often leading to unexpected results.Learn the difference between explicit and implicit coercion. Explicit coercion occurs when you manually convert data types using functions like Number(), String(), and Boolean(). Implicit coercion, however, is handled by JavaScript, such as when using the + operator to concatenate a string and a number.Delve into common scenarios where type coercion occurs, such as in comparisons (== vs ===), arithmetic operations, and logical expressions. Recognize potential pitfalls, like the conversion of null to 0 or undefined to NaN, and learn best practices to manage and predict type coercion behavior in your code. This topic equips you with the knowledge to write more predictable and bug-free JavaScript applications.
-
14- Understanding functionsVideo lesson
Functions are at the heart of JavaScript programming, offering a way to encapsulate code for reuse and modularity. This module explains the concept of functions in JavaScript, detailing how they are defined and invoked. Learners will distinguish between function expressions and function declarations, understanding the nuances and use cases of each.
-
15Unlocking the Power of Functions in JavaScriptQuiz
-
16- Function expressions and declarationsVideo lesson
Emphasis is placed on the importance of functions for organizing code, improving readability, and the principles of scope and closure. By mastering functions, students will advance their JavaScript proficiency, paving the way for more complex programming constructs and patterns.
-
17Decoding Function Expressions and DeclarationsQuiz
-
18Anonymous FunctionsVideo lesson
Discover the power and flexibility of anonymous functions in JavaScript. Unlike named functions, anonymous functions are defined without a name and are often used as arguments to other functions or assigned to variables. Learn how these functions enhance code readability and maintainability by reducing boilerplate and making your code more concise.Understand typical use cases for anonymous functions, such as callbacks in event handlers, timers, and array methods like map(), filter(), and reduce(). Explore the syntax and differences between anonymous function expressions and named function expressions.Anonymous functions are crucial in functional programming patterns and are widely used in modern JavaScript frameworks and libraries. By mastering this topic, you'll be able to write more efficient and cleaner code, leveraging the versatility of anonymous functions to streamline your JavaScript development.
-
19IIFE (Immediately Invoked Function Expressions)Video lesson
Dive into the concept of Immediately Invoked Function Expressions (IIFE) in JavaScript, a powerful pattern that allows functions to be executed as soon as they are defined. IIFEs are anonymous functions wrapped in parentheses and followed by another set of parentheses for immediate invocation.Understand the primary use cases and benefits of IIFEs, such as creating a private scope to avoid polluting the global namespace, encapsulating code to protect it from external interference, and executing initialization code without leaving a footprint in the global scope.Explore the syntax and variations of IIFEs, including their use with arrow functions in modern JavaScript. Learn how to use IIFEs to implement module patterns, enabling better organization and modularity in your code. By mastering IIFEs, you'll gain the ability to write cleaner, more efficient, and more maintainable JavaScript code.
-
20Higher-order FunctionsVideo lesson
Unlock the power of higher-order functions in JavaScript, a fundamental concept where functions operate on other functions by taking them as arguments or returning them. This approach is central to functional programming, allowing for more abstract and flexible code.
Learn how higher-order functions enable advanced operations like filtering, mapping, and reducing arrays through methods like `filter()`, `map()`, and `reduce()`. Explore real-world applications such as event handling, asynchronous programming with promises, and implementing callbacks.
Understand the benefits of higher-order functions, including code reuse, improved readability, and the ability to write more declarative code. Dive into practical examples and best practices, enhancing your ability to write elegant and efficient JavaScript code by leveraging the full potential of higher-order functions.
-
21ClosuresVideo lesson
Discover the powerful concept of closures in JavaScript, an essential feature that allows functions to retain access to their lexical scope even when executed outside of it. Understand how closures enable functions to "remember" the environment in which they were created, providing access to outer function variables and parameters even after the outer function has returned.Learn practical use cases for closures, such as creating private variables, implementing function factories, and maintaining state in asynchronous code. Explore how closures are commonly used in event handlers, callback functions, and module patterns to encapsulate and protect data.Closures are a foundational concept in JavaScript, crucial for writing robust and modular code. By mastering closures, you'll enhance your ability to create flexible and maintainable functions, effectively managing scope and variable lifetimes in your JavaScript applications.
-
22CallbacksVideo lesson
Master the concept of callbacks in JavaScript, a key technique for handling asynchronous operations. Callbacks are functions passed as arguments to other functions and executed after the completion of a task, enabling non-blocking code execution.Understand how callbacks work in various scenarios, such as event handling, timers, and network requests. Learn to implement callbacks to perform sequential operations, handle responses from asynchronous functions, and manage dependencies between tasks.Explore the common challenges and pitfalls of using callbacks, including callback hell and pyramid of doom, and discover strategies to mitigate these issues, such as modularizing code and using named callback functions.By mastering callbacks, you'll be able to write efficient, responsive, and maintainable JavaScript code, handling asynchronous operations with ease and improving the overall user experience in your applications.
-
23- Let and ConstVideo lesson
In JavaScript, let and const introduce block scope variables, enhancing code readability and maintainability. The let keyword allows you to declare variables that are limited in scope to the block, statement, or expression where they are used, distinct from the global scope of the var keyword. This prevents variables from being accessed outside their intended scope. On the other hand, const is used to declare variables meant to remain constant through the execution of a program, offering protection against reassignment. Understanding let and const is crucial for managing variable lifecycles and ensuring code integrity
-
24Exploring Let and Const in JavaScriptQuiz
-
25- Arrow functionsVideo lesson
Arrow functions provide a concise syntax for writing function expressions in JavaScript, using the => arrow notation. They offer several advantages over traditional function expressions, such as lexical this binding, which means they do not create their own this context, making them ideal for use in callbacks and methods within objects. This feature simplifies the behavior of asynchronous code and methods in classes. Learning to utilize arrow functions is key to writing cleaner, more readable JavaScript code, especially when dealing with higher-order functions or event handlers
-
26Navigating the World of Arrow FunctionsQuiz
-
27- Template literalsVideo lesson
Template literals in JavaScript are a significant enhancement for working with strings. Enclosed by the backtick ` characters, they allow for embedded expressions, called placeholders, within string literals. This feature simplifies the process of concatenating strings with variables or expressions, improving code readability and maintainability. Template literals support multiline strings and basic string formatting capabilities without the need for complex concatenation or function calls, making them invaluable for generating dynamic content.
-
28Crafting Dynamic Strings with Template LiteralsQuiz
-
29- Destructuring assignmentVideo lesson
Destructuring assignment in JavaScript provides a succinct syntax for extracting values from arrays or properties from objects into distinct variables. It simplifies working with data structures by reducing the need for explicit assignments and temporary variables, leading to cleaner and more readable code. Destructuring is particularly useful in scenarios involving function parameters, return values, or manipulating data stored in objects and arrays, enhancing code efficiency and readability.
-
30Unraveling Destructuring Assignment in JavaScriptQuiz
-
31- Spread and Rest OperatorsVideo lesson
The spread and rest operators (...) serve dual purposes in JavaScript. The spread operator allows an iterable such as an array or string to be expanded in places where zero or more arguments (for function calls) or elements (for array literals) are expected. It is widely used for array or object cloning and merging. The rest operator, conversely, collects multiple elements and condenses them into a single array. This is incredibly useful for function definitions, allowing you to handle an indefinite number of arguments as an array. Understanding these operators is pivotal for writing flexible and concise JavaScript functions.
-
32Harnessing the Power of Spread and Rest OperatorsQuiz
-
33- Default parametersVideo lesson
Default parameters in JavaScript functions enable the initialization of named parameters with default values if no value or undefined is passed. This feature enhances function flexibility and readability, allowing for more predictable outcomes and fewer errors in function calls. Default parameters support not just simple values but also complex expressions and function calls, making the functions in JavaScript more powerful and versatile.
-
34Enhancing Functions with Default ParametersQuiz
-
35- Modules and ImportsVideo lesson
JavaScript modules are a method to split the code of a script into manageable pieces or files. With import and export statements, modules allow for the separation of concerns, clarify namespace management, and improve code reusability and maintainability. Understanding how to structure a project with modules and how to import or export components, libraries, or utilities is crucial for developing scalable JavaScript applications
-
36Navigating the World of Modules and Imports in JavaScriptQuiz
-
37ClassesVideo lesson
Explore the power of classes in JavaScript, introduced in ES6, to bring a more structured and object-oriented approach to your code. Classes provide a clean syntax for creating objects and managing inheritance, making your code more readable and maintainable.
Learn how to define classes using the `class` keyword, create instances with the `constructor` method, and use methods to add functionality to your objects. Understand the benefits of using classes for creating reusable and scalable code structures.
Delve into advanced features such as inheritance, allowing you to create subclasses with the `extends` keyword, and the `super` keyword to call parent class methods. Discover how to use getters and setters for encapsulating and managing access to object properties.
By mastering classes, you'll be equipped to write more organized and modular JavaScript code, leveraging the full potential of object-oriented programming principles in your projects.
-
38Promises and Async/AwaitVideo lesson
Master asynchronous programming in JavaScript with Promises and Async/Await, essential tools for managing operations that take time to complete, such as network requests and file I/O.
Start with Promises, objects that represent the eventual completion or failure of an asynchronous operation. Learn how to create and handle Promises using `resolve` and `reject`, and manage their outcomes with `.then()`, `.catch()`, and `.finally()` methods. Understand chaining Promises for sequential operations and how to handle multiple Promises simultaneously with `Promise.all()` and `Promise.race()`.
Advance to Async/Await, a modern syntax built on Promises that allows writing asynchronous code that looks synchronous, enhancing readability and maintainability. Explore how to define asynchronous functions using the `async` keyword and pause their execution with `await`, ensuring clean, linear flow control without deep nesting.
By mastering Promises and Async/Await, you'll be able to write efficient, non-blocking code, improving performance and user experience in your JavaScript applications.
-
39Symbol TypeVideo lesson
Explore the Symbol type in JavaScript, introduced in ES6, a unique and immutable primitive value used to create anonymous object properties. Symbols are essential for avoiding property name collisions, especially in scenarios involving multiple scripts or libraries.
Understand how to create symbols using the `Symbol()` function, and how each symbol is guaranteed to be unique, even if they have the same description. Learn how to use symbols as object property keys, providing a way to define non-enumerable properties that are hidden from typical property access methods like `for...in` loops or `Object.keys()`.
Delve into the concept of global symbols with `Symbol.for()` and `Symbol.keyFor()`, which allow symbol sharing across different parts of your codebase. Explore well-known symbols such as `Symbol.iterator`, `Symbol.toStringTag`, and `Symbol.hasInstance`, which extend and customize object behavior.
By mastering the Symbol type, you'll be equipped to write more robust and collision-free JavaScript code, leveraging the full capabilities of symbols for advanced programming techniques and ensuring better namespace management in your applications.
-
40Iterators and GeneratorsVideo lesson
Delve into Iterators and Generators in JavaScript, powerful features introduced in ES6 that facilitate custom iteration behavior and efficient data processing.Start with iterators, which provide a standard way to traverse through elements of a collection. Learn how to create an iterator using the `next()` method, which returns objects with `value` and `done` properties. Understand the importance of the `Symbol.iterator` method in making objects iterable, allowing them to be used in `for...of` loops, spread syntax, and other iteration contexts.Advance to generators, special functions that simplify the creation of iterators. Define generators using the `function*` syntax and the `yield` keyword to pause and resume function execution, producing a sequence of values over time. Explore practical use cases of generators, such as handling asynchronous flows, producing infinite sequences, and managing complex data streams.By mastering iterators and generators, you'll be able to write more flexible and efficient JavaScript code, capable of handling custom iteration logic and optimizing performance in data-intensive applications.
-
41- What are objects?Video lesson
Objects in JavaScript are collections of related data and functionality. They are used to structure and represent information about the real world in a manner that can be managed and manipulated within code. JavaScript objects can be created through literal notation or constructors, and they can hold properties and methods. Knowing how to effectively create, manipulate, and access objects is fundamental to applying JavaScript in web development, as objects form the backbone of most JavaScript applications.
-
42Understanding the Essence of Objects in JavaScriptQuiz
-
43- Creating and accessing objectsVideo lesson
Creating and accessing objects in JavaScript are foundational skills for web developers. Objects can be created using braces {}, with properties and methods defined within. Accessing these properties and methods is done using dot notation or bracket notation, allowing for dynamic interaction with the parts of an object. Mastering object creation and access patterns is crucial for structuring applications and managing data in JavaScript.
-
44Crafting and Accessing Objects in JavaScriptQuiz
-
45- ArraysVideo lesson
Arrays in JavaScript are used to store multiple values in a single variable. They are objects that represent a list of items, providing methods and properties to perform operations like searching, sorting, and manipulating data. Arrays are zero-indexed, with the first element at index 0. Understanding arrays and their methods is crucial for handling collections of data, making them one of the most frequently used structures in JavaScript programming
-
46Crafting and Accessing Arrays in JavaScriptQuiz
-
47- Array methodsVideo lesson
JavaScript arrays come with a variety of built-in methods that allow for easy manipulation and traversal of the array elements. Methods such as map(), filter(), reduce(), forEach(), and many others provide powerful tools for performing operations on arrays, from transforming each item in an array to filtering out items based on a condition. Familiarity with these methods is essential for efficient data handling and functional programming in JavaScript.
-
48Exploring the Array Realm in JavaScriptQuiz
-
49Prototypes and InheritanceVideo lesson
Unlock the power of prototypes and inheritance in JavaScript, foundational concepts for creating reusable and efficient object-oriented code.Start with prototypes, the mechanism by which JavaScript objects inherit properties and methods. Learn how every JavaScript object has a prototype, accessible via the `__proto__` property, and how prototype chaining allows objects to inherit from other objects, enabling method and property sharing.Explore how to create and link prototypes using constructor functions and the `new` keyword. Understand the role of the `prototype` property in defining methods and properties that are shared across all instances of a constructor function.Dive into inheritance, a key principle for building hierarchical class structures. Learn how to implement inheritance using `Object.create()` and the `class` syntax introduced in ES6, which provides a more intuitive and readable way to work with prototypes. Discover how the `extends` keyword allows subclasses to inherit from parent classes, and how to use the `super` keyword to call and extend parent class methods.By mastering prototypes and inheritance, you'll be able to write more modular, maintainable, and scalable JavaScript code, leveraging the full potential of object-oriented programming to create sophisticated applications.
-
50Object.freeze() and Object.seal()Video lesson
Understand the importance of `Object.freeze()` and `Object.seal()` in JavaScript, two methods that help control object mutability and enhance data integrity.
Object.freeze()
`Object.freeze()` makes an object immutable. Once an object is frozen:
- No new properties can be added.
- Existing properties cannot be removed or modified.
- The prototype cannot be changed.
- Attempts to change the object will silently fail in non-strict mode or throw an error in strict mode.
Use `Object.freeze()` to create read-only objects, ensuring that their state remains constant throughout the lifecycle of your application.
Object.seal()
`Object.seal()` allows modification of existing properties but prevents the addition or removal of properties:
- You can still change the values of existing properties.
- Properties cannot be deleted or added.
- The prototype cannot be changed.
`Object.seal()` is useful when you need to ensure the object structure remains consistent but still require the ability to update existing property values.By mastering `Object.freeze()` and `Object.seal()`, you'll be equipped to manage object immutability and integrity in your JavaScript applications, leading to more predictable and robust code.
-
51Object DestructuringVideo lesson
Unlock the power of object destructuring in JavaScript, an ES6 feature that allows you to extract properties from objects and assign them to variables with a clean and concise syntax. This powerful technique simplifies the process of accessing and working with object properties.
Learn the basic syntax of object destructuring, which involves extracting properties from an object and assigning them to variables using curly braces on the left side of the assignment.
Explore advanced destructuring techniques, including assigning default values to variables if the corresponding property is undefined, renaming variables to avoid conflicts or improve readability, extracting properties from nested objects in a single statement, and using rest syntax to collect remaining properties into a new object.
By mastering object destructuring, you can write more readable and maintainable JavaScript code, reducing boilerplate and making it easier to work with complex objects.
-
53ArraysVideo lesson
Arrays in JavaScript are used to store multiple values in a single variable. They are objects that represent a list of items, providing methods and properties to perform operations like searching, sorting, and manipulating data. Arrays are zero-indexed, with the first element at index 0. Understanding arrays and their methods is crucial for handling collections of data, making them one of the most frequently used structures in JavaScript programming.
-
54Optimizing Arrays with Array MethodsQuiz
-
55LoopsVideo lesson
Loops are a fundamental aspect of JavaScript, allowing for the execution of a block of code multiple times based on a specified condition. JavaScript supports several types of loops, including for, while, and for...of, each with its use-case. Loops are essential for iterating over data structures like arrays or objects, performing repetitive tasks efficiently. Knowing how to effectively use loops can significantly enhance a program’s ability to process data and perform complex operations
-
56Navigating Loops in JavaScriptQuiz
-
57Array methodsVideo lesson
JavaScript arrays come with a variety of built-in methods that allow for easy manipulation and traversal of the array elements. Methods such as map(), filter(), reduce(), forEach(), and many others provide powerful tools for performing operations on arrays, from transforming each item in an array to filtering out items based on a condition. Familiarity with these methods is essential for efficient data handling and functional programming in JavaScript.
-
58Mastering the Art of Array ManipulationQuiz
-
59Multi-dimensional ArraysVideo lesson
Discover the concept of multi-dimensional arrays in JavaScript, an essential feature for handling complex data structures. Multi-dimensional arrays are arrays that contain other arrays as their elements, creating a grid-like structure ideal for representing matrices, tables, and other multi-level data.
Understand the basics of creating and accessing multi-dimensional arrays. Each element within a multi-dimensional array can be accessed using multiple indices, representing its position in each dimension.
Explore practical applications, such as storing and manipulating data in two-dimensional arrays (tables or matrices) and extending this concept to three or more dimensions for more complex data models. Multi-dimensional arrays are particularly useful in scenarios like game development, data analysis, and scientific computing.
By mastering multi-dimensional arrays, you'll be equipped to handle complex data structures efficiently, making your JavaScript code more powerful and versatile for a wide range of applications.
-
60for...in LoopVideo lesson
Learn about the for...in loop in JavaScript, a fundamental construct used for iterating over the enumerable properties of an object. This loop allows you to access and manipulate each property in an object efficiently.Understand how the for...in loop works: it iterates through the keys (property names) of an object, providing a simple way to inspect or modify the values associated with each key. This loop is particularly useful when working with objects whose properties need to be accessed dynamically.
Explore practical applications of the for...in loop, such as iterating over object properties to perform operations like logging values, copying objects, or implementing algorithms that require inspecting object contents. By mastering the for...in loop, you'll be able to write more efficient and dynamic JavaScript code, making it easier to work with objects and their properties in a variety of programming scenarios.
-
61for...of LoopVideo lesson
Explore the for...of loop in JavaScript, a powerful construct introduced in ES6 for iterating over iterable objects like arrays, strings, maps, sets, and more. Unlike the for...in loop, which iterates over property names, the for...of loop iterates over the values of an iterable.
Understand how the for...of loop provides a cleaner and more readable syntax for accessing each element in a collection. This loop simplifies working with arrays and other iterable objects by directly yielding their values.
Discover practical applications of the for...of loop, such as iterating through array elements, processing characters in a string, traversing nodes in a NodeList, and handling entries in collections like maps and sets.
By mastering the for...of loop, you'll enhance your ability to write concise and efficient JavaScript code, making it easier to work with various iterable data structures in your applications.
-
62What is DOM?Video lesson
The Document Object Model (DOM) is a critical concept in web development, serving as the bridge between JavaScript and the structure of HTML documents. Understanding the DOM is essential for JavaScript developers, as it allows them to dynamically interact with the webpage, modifying the document structure, style, and content. The DOM represents the page so that programs can change the document structure, style, and content. JavaScript uses the DOM to create interactive web experiences, enabling web pages to react to user inputs without the need to reload. This topic covers the fundamentals of DOM, how it is structured, and how JavaScript can be used to read and manipulate web page content, thereby enhancing user engagement and experience.
-
63Delving into the World of the Document Object Model (DOM)Quiz
-
64Selecting and changing elementsVideo lesson
This topic delves into the core methods JavaScript offers for selecting and modifying webpage elements, highlighting the power of JavaScript to create dynamic and interactive web applications. Learners will explore various selectors provided by the DOM, such as getElementById, getElementsByClassName, querySelector, and querySelectorAll, to efficiently select elements within a web page. Furthermore, we will cover how to use JavaScript to change the content, properties, and attributes of selected elements, demonstrating the dynamic capabilities of web applications. By mastering element selection and manipulation, developers can create more responsive, interactive, and tailored web experiences.
-
65Manipulating Elements with JavaScriptQuiz
-
66Event listenersVideo lesson
Event listeners are a cornerstone of interactive web development, allowing JavaScript developers to execute code in response to user actions such as clicks, keyboard input, and page loads. This topic teaches how to use JavaScript to register and handle events, enabling dynamic page behavior without refreshing the page. We cover different types of events, how to attach event listeners to DOM elements, and the use of anonymous functions or named event handlers to respond to user interactions. Through practical examples and best practices, learners will understand how to create seamless and interactive user experiences.
-
67Harnessing the Power of Event ListenersQuiz
-
68Manipulating CSS classes and inline stylesVideo lesson
Manipulating CSS classes and inline styles with JavaScript is essential for dynamically changing the appearance and layout of web pages. This topic explores the mechanisms JavaScript provides to add, remove, or toggle CSS classes, and how to directly modify the inline styles of HTML elements. Learners will understand the importance of separating structure from presentation and how JavaScript can control webpage styling to reflect state changes or user interactions, significantly improving the user interface and experience.
-
69Styling Elements Dynamically with CSS in JavaScriptQuiz
-
70Event DelegationVideo lesson
Learn about event delegation in JavaScript, a technique that allows you to manage events more efficiently by taking advantage of event bubbling. Instead of attaching event listeners to individual elements, you attach a single event listener to a parent element. This parent element can handle events triggered by its child elements, making your code more efficient and easier to manage.
Understand the benefits of event delegation:
Performance: Reduces the number of event listeners, which can enhance performance, especially in applications with many interactive elements.
Simplicity: Simplifies event handling by consolidating multiple event listeners into a single listener on a common ancestor.
Dynamic Content Handling: Easily manages events for dynamically added or removed elements, as the event listener on the parent element can handle events for new child elements without additional setup.
Explore practical applications, such as handling click events for a list of items, form validation, and managing complex UI interactions. By mastering event delegation, you'll be able to write more scalable and maintainable JavaScript code, efficiently handling events in your web applications.
-
71Working with FormsVideo lesson
Explore how to effectively work with forms in JavaScript, essential for handling user input and processing data in web applications.
Understand the basics of form manipulation:
Accessing Form Elements: Use methods like getElementById, querySelector, or accessing form elements directly via their name attribute.
Form Submission: Handle form submission events using the submit event and prevent default behavior to manage form data programmatically.
Form Validation: Implement client-side validation using HTML5 form validation attributes (required, minlength, etc.) or custom JavaScript validation logic.
Modifying Form Elements: Dynamically update form element values, attributes, or styles based on user interaction or application logic.
Asynchronous Form Submission: Use fetch or XMLHttpRequest to submit form data asynchronously, handle responses, and update the UI accordingly.
Explore practical use cases such as login forms, registration forms, data entry, and complex form interactions in single-page applications (SPAs).
By mastering form handling techniques in JavaScript, you'll enhance user experience, improve data integrity, and create responsive web applications that effectively interact with users.
-
72Introduction to CanvasVideo lesson
Discover the Canvas API in JavaScript, a powerful tool for drawing graphics, creating animations, and rendering complex visual content directly in the browser. The <canvas> element, introduced in HTML5, provides a versatile and efficient way to build dynamic, interactive visualizations.
Understand the basics of using the Canvas API:
Setting Up the Canvas: Learn how to add a <canvas> element to your HTML and set its width and height.
Rendering Context: Get the 2D rendering context using getContext('2d'), which provides methods for drawing shapes, text, images, and more.
Drawing Shapes and Lines: Explore methods to draw basic shapes (rectangles, circles) and lines, and understand how to style them with colors, gradients, and patterns.
Working with Text: Learn how to render text with various fonts, sizes, and alignments.
Manipulating Images: Discover how to draw images onto the canvas, manipulate pixel data, and create effects like cropping and scaling.
Creating Animations: Utilize the requestAnimationFrame method to build smooth, efficient animations.
By mastering the Canvas API, you'll be able to create rich, interactive graphics and animations, enhancing the visual experience of your web applications and engaging users with compelling content.
-
73Try - Catch - FinallyVideo lesson
Error handling is a crucial part of developing robust JavaScript applications. The try-catch-finally construct provides a structured approach to detect errors in code execution and handle them gracefully without stopping the entire script. This topic focuses on the syntax and practical use of try, catch, and finally blocks in JavaScript, including how to catch different types of errors and clean up resources, ensuring applications remain stable and reliable even when unexpected situations occur.
-
74Mastering Error Handling with Try-Catch-Finally in JavaScriptQuiz
-
75Throw and Custom ErrorsVideo lesson
Beyond built-in error handling, JavaScript allows developers to throw their own errors using the throw statement, enabling more refined control over error management within applications. This topic covers how to create and use custom error objects to convey more specific information about issues that arise during execution. Learners will discover techniques for throwing, catching, and handling custom errors, facilitating more nuanced error handling strategies that enhance debugging and application resilience.
-
76Unleashing the Power of Custom Errors in JavaScriptQuiz
-
77Error ObjectsVideo lesson
Dive into error objects in JavaScript, a crucial component for handling and managing errors effectively. Error objects provide detailed information about runtime errors, helping you diagnose and debug issues in your code.
Understand the basics of error objects:
Creating Error Objects: Learn how to create an error object using the Error constructor or specialized constructors like TypeError, SyntaxError, ReferenceError, and RangeError.
Error Properties: Explore key properties of error objects, such as name (type of error), message (description of the error), and stack (stack trace), which provide valuable information about the nature and origin of the error.
Throwing Errors: Discover how to use the throw statement to generate custom errors in your code, allowing you to signal and handle exceptional conditions explicitly.
Catching Errors: Understand how to catch and handle errors using try...catch blocks, ensuring that your code can gracefully recover from unexpected issues and maintain stability.
Custom Error Types: Learn how to define custom error types by extending the Error class, enabling you to create more specific and meaningful error objects tailored to your application's needs.
By mastering error objects, you'll enhance your ability to debug, handle exceptions, and maintain robust, reliable JavaScript applications.
-
78Async Error HandlingVideo lesson
Master async error handling in JavaScript, an essential skill for managing errors in asynchronous operations such as network requests, file I/O, and timers.
Understand the challenges of asynchronous code:
Callbacks: Learn how to handle errors in callback-based code by following the convention of passing an error object as the first argument to the callback function.
Promises: Explore error handling in Promises using .catch() to handle rejections, ensuring that any error occurring in the promise chain is properly managed.
Chaining Promises: Understand the importance of returning promises in chains and using multiple .catch() methods to handle errors at different stages.
Async/Await: Discover how to use try...catch blocks in conjunction with async functions and the await keyword to handle errors in a more synchronous, readable manner.
-
79Logging and Monitoring ErrorsVideo lesson
Enhance your JavaScript applications' reliability by implementing effective error logging and monitoring strategies. Proper logging and monitoring help you detect, diagnose, and resolve issues quickly, ensuring a seamless user experience.
Logging Errors
Console Logging: Utilize console.error(), console.warn(), and console.log() to log errors, warnings, and informational messages during development.
Custom Logging Functions: Create custom logging functions to standardize log messages and direct them to different outputs (console, files, remote servers).
Error Object Properties: Log detailed error information, including the error message, name, stack trace, and custom properties, to provide comprehensive insights into the issue.
Monitoring Errors
Error Tracking Services: Integrate with error tracking services like Sentry, LogRocket, or Bugsnag to automatically capture, store, and analyze errors in real-time. These services offer dashboards, alerting, and detailed reports.
Performance Monitoring Tools: Use performance monitoring tools like New Relic, Datadog, or Google Analytics to track application performance and detect anomalies that might indicate underlying issues.
Remote Logging: Implement remote logging to send error logs to a centralized server for aggregation and analysis, ensuring that client-side errors are captured and reviewed.
-
80Coding styleVideo lesson
A consistent coding style is vital for maintaining readability and manageability of JavaScript codebases. This topic emphasizes the importance of adhering to a coding style guide, discussing common conventions and best practices in JavaScript coding, including naming conventions, formatting, and structuring code. Learners will understand how a unified coding style improves code quality, collaboration among developers, and reduces the likelihood of errors.
-
81Crafting Clean Code: The JavaScript Coding StyleQuiz
-
82CommentsVideo lesson
A consistent coding style is vital for maintaining readability and manageability of JavaScript codebases. This topic emphasizes the importance of adhering to a coding style guide, discussing common conventions and best practices in JavaScript coding, including naming conventions, formatting, and structuring code. Learners will understand how a unified coding style improves code quality, collaboration among developers, and reduces the likelihood of errors.
-
83The Art of Commenting in JavaScriptQuiz
-
84Testing and debuggingVideo lesson
Testing and debugging are key to delivering error-free JavaScript applications. This topic introduces the fundamentals of writing test cases and using debugging tools to identify, diagnose, and resolve issues in JavaScript code. It covers unit testing, integration testing, and the use of developer tools in browsers for debugging. By learning how to effectively test and debug, developers can enhance the reliability, performance, and user experience of their web applications.
-
85Testing and Debugging: Ensuring Smooth JavaScript ExecutionQuiz
-
86Code OptimizationVideo lesson
Enhance the performance and efficiency of your JavaScript applications through effective code optimization techniques. Code optimization involves refining your code to reduce execution time, memory usage, and improve overall responsiveness. Focus on minimizing loops, reducing function calls, and leveraging built-in methods for common tasks. Ensure your code is DRY (Don't Repeat Yourself) and avoid unnecessary computations by storing results that are reused multiple times.
Utilize tools like minifiers to compress your code and remove extraneous characters, thereby reducing file size and improving load times. Optimize your use of asynchronous operations to prevent blocking the main thread, and employ techniques like debouncing and throttling to manage high-frequency events efficiently. Regularly profile your code using browser developer tools to identify bottlenecks and areas for improvement, ensuring your application runs smoothly and efficiently.
-
87Using Linting ToolsVideo lesson
Improve the quality and consistency of your JavaScript code by using linting tools. Linters automatically analyze your code to detect potential errors, enforce coding standards, and ensure best practices. Tools like ESLint and JSHint are popular choices that provide comprehensive rule sets and can be customized to fit your project's needs.
Integrate linting into your development workflow to catch issues early and maintain a clean codebase. You can configure linting rules to align with your team's coding style, and set up pre-commit hooks to run lint checks before code is committed to version control. By consistently using linting tools, you'll reduce bugs, improve readability, and foster a more maintainable and collaborative code environment.
-
88Version Control with GitVideo lesson
Master version control with Git, an essential tool for managing code changes, collaborating with team members, and maintaining a history of your project's development. Git allows you to track changes, revert to previous states, and create branches for feature development or bug fixes without affecting the main codebase. This facilitates parallel development and helps maintain code stability.
Understand the basics of using Git: initializing repositories, staging changes, committing updates, and pushing commits to remote repositories like GitHub or GitLab. Learn how to handle merge conflicts, use branching strategies like Git Flow, and leverage pull requests for code reviews. By integrating Git into your workflow, you ensure a structured, organized approach to development, making it easier to collaborate and maintain high-quality code over time.
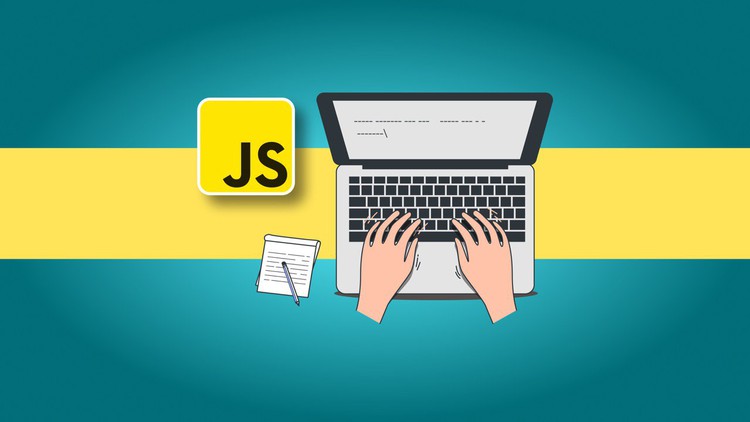
External Links May Contain Affiliate Links read more