Programming with C++ Language: The Complete Course
- Description
- Curriculum
- FAQ
- Reviews
Learn C++ Programming Language from basic to advance level easily by understanding every topic with practical session. Here I will explain every topics and subtopics in very easy language which helps the beginner to understand. This course uses the latest version C++ 17. You do not find any C++ tutorial anywhere like this course. This course include theory, concepts and practical of C++. Now a days C++ is very popular programming language and one of the top programming language also.
Introduction to C++
•First appeared in 1985
•C++ has expanded significantly time to time
•C++ is Object Oriented Programming language
•It is available in all platform
•Filename extension is .cpp
•Recent version is C++ 17 (December 2017)
•Recently C++ is mostly used in system programming and embedded system.
Features of C++
•Simple
•Portable
•Powerful
•Platform Dependent
•Efficient
•Object Oriented
•Rich Library
•Structured Programming language
•Case sensitive
•Security
Support dynamic memory allocation
What you can develop using C++?
•Operating System (Windows)
•Browsers (Mozilla Firefox, Chrome etc)
•IoT products
•Embedded System
•Compilers
•Databases (MySql)
•Gaming Development
•GUI Based Application (Adobe systems)
many more……..
-
8Structure of C++ ProgramVideo lesson
A basic structure of a C++ program includes the following elements:
Preprocessor Directives: These are the lines that start with a pound sign (#). They are used to include libraries and other files in the program. For example:
#include <iostream>
#include <cmath>
Namespace Declaration: This line declares the namespace to be used in the program. The standard namespace for C++ is std. For example:
using namespace std;
Main Function: The main function is the entry point of a C++ program. All C++ programs must have a main function. The code inside the main function will be executed first when the program runs. For example:
int main()
{
// program statements go here
return 0;
}
Variables Declaration: Variables can be declared inside the main function. A variable has a data type, a name, and an optional value. For example:
int a = 10;
double b = 20.5;
string name = "John Doe";
Statements and Expressions: Statements are instructions that perform actions. Expressions are combinations of variables and operators that evaluate to a value. Both statements and expressions can be included in the main function. For example:
cout << "Hello, World!" << endl;
int sum = a + b;
Function calls: Functions can be called from the main function or from other functions. Functions are used to encapsulate a block of code and can be reused multiple times. For example:
cout << "The sum is: " << sum << endl;
printResult(sum);
This is a basic structure of a C++ program. Depending on the requirements, a C++ program can include more elements such as classes, objects, arrays, loops, conditional statements, etc.
-
9Write and Run your First C++ ProgramVideo lesson
Here's an example of a basic "Hello, World!" program in C++:
#include <iostream>
using namespace std;
int main()
{
cout << "Hello, World!" << endl;
return 0;
}
Explanation:
The first line, #include <iostream>, is a preprocessor directive that includes the iostream library, which provides input and output capabilities to the program.
The line using namespace std; declares the std namespace, which contains the standard library functions.
The main function is the entry point of the program and it returns an int value. In this example, it returns 0, which indicates that the program ran successfully.
The line cout << "Hello, World!" << endl; uses the cout object from the iostream library to print the string "Hello, World!" to the standard output (usually the console). The endl is a special manipulator that adds a newline character after the output.
This program can be saved in a text file with a .cpp extension and compiled using a C++ compiler to produce an executable file. When run, the program will print "Hello, World!" to the console.
-
10Variables and ConstantsVideo lesson
In C++, variables are used to store values in memory, while constants are used to store values that cannot be changed during the execution of the program.
Variables are declared by specifying the data type followed by the name of the variable. For example:
int age;
double weight;
string name;
Variables can also be initialized at the time of declaration, as shown below:
int age = 30;
double weight = 75.5;
string name = "John Doe";
Constants, on the other hand, are declared using the keyword const. Constants must also be initialized at the time of declaration and their values cannot be changed later in the program. For example:
const int MAX_AGE = 120;
const double PI = 3.14159;
const string APP_NAME = "MyApp";
Note that the names of constants are usually written in uppercase letters to distinguish them from variables.
In C++, there are also several data types available for variables and constants, including int (for integers), double (for floating-point numbers), char (for single characters), bool (for Boolean values), and string (for strings of characters).
-
11cin, cout and endl (Objects of Input and Output Stream)Video lesson
-
12Primary DataTypesVideo lesson
C++ provides several built-in data types for storing different types of values, including:
int: An integer type that can store whole numbers within a certain range (usually -2147483648 to 2147483647).
float: A floating-point type that can store fractional numbers with single precision.
double: A floating-point type that can store fractional numbers with double precision.
char: A character type that can store a single character, such as a letter, symbol, or digit. It's stored as an integer value in the computer's memory and typically uses 1 byte of memory.
bool: A Boolean type that can store either true or false.
void: A special type that represents the absence of a type. It is used as the return type for functions that do not return a value.
In addition to these basic data types, C++ also provides several other data types, including arrays, structures, classes, and more. It's important to choose the appropriate data type for a given situation to ensure the program behaves correctly and uses memory efficiently.
-
13Example ProgramsVideo lesson
Here are five basic example programs in C++:
"Hello, World!" program:
#include <iostream>
using namespace std;
int main()
{
cout << "Hello, World!" << endl;
return 0;
}
Addition of two numbers program:
#include <iostream>
using namespace std;
int main()
{
int num1, num2, sum;
cout << "Enter two numbers: ";
cin >> num1 >> num2;
sum = num1 + num2;
cout << "The sum is: " << sum << endl;
return 0;
}
Conversion of Celsius to Fahrenheit program:
#include <iostream>
using namespace std;
int main()
{
double celsius, fahrenheit;
cout << "Enter temperature in Celsius: ";
cin >> celsius;
fahrenheit = (celsius * 9.0 / 5.0) + 32;
cout << "Temperature in Fahrenheit: " << fahrenheit << endl;
return 0;
}
-
14Quiz based on Section 3Quiz
Quiz based on Section 3
-
15Practice Test Based on Basics of C++ Programming LanguageQuiz
-
16Coding Exercise 1Quiz
-
17Operators and Type of OperatorsVideo lesson
-
18Demonstartion of Arithmetic OperatorsVideo lesson
-
19Demonstration of Relational OperatorVideo lesson
-
20Demonstration of Increment and Decrement OperatorVideo lesson
-
21Quiz Based on Section 4(Operators and Expression)Quiz
Quiz Based on Section 4(Operators and Expression)
-
22Practice Test Based on OperatorsQuiz
-
23Conditional/Decision making Satements in C++Video lesson
-
24if statementVideo lesson
-
25if....else statementVideo lesson
-
26if...else if ladderVideo lesson
-
27Nested if StatementVideo lesson
-
28switch...case statementVideo lesson
-
29Example ProgramsVideo lesson
-
30Quiz based on Section 5 (Decision making statements in C++)Quiz
Quiz based on Section 5 (Decision making statements in C++)
-
66What is Inheritance ?Type of InheritanceVideo lesson
-
67Private, Public and ProtectedVideo lesson
-
68Implementation of Single InheritanceVideo lesson
-
69Implementtation of Multiple InheritanceVideo lesson
-
70Implementtation of Multlevel InheritanceVideo lesson
-
71Quiz based on Section 14(Inheritance)Quiz
Quiz based on Section 14(Inheritance)
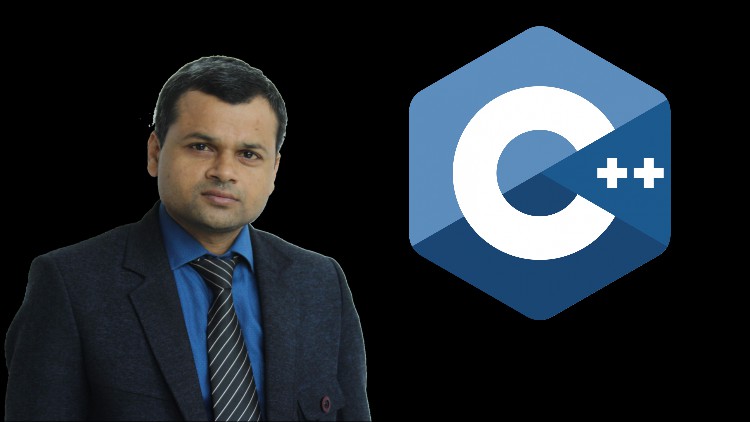
External Links May Contain Affiliate Links read more