Technical Java Interview Prep for IT professionals
- Description
- Curriculum
- FAQ
- Reviews
Hello. We have been waiting for you. Welcome. Now that you are here, let’s begin. Thank you for being here.
Java has been in the IT field for years. By coding hands on as you watch video lessons, you will gain the skills to Solve given problems in java that may show up in the interviews. Some lessons may be from recent interviews collected from the local community. We aim to create a discord community to crowdsource technical java interview information. Time to time, add in resources to the course and use a discord server to discuss current and past frequently asked interview questions. Host live events to help beginners learn more about java interview questions. Provide insights regarding solving the technical coding problems.
Create a code resource depot using submitted input interview problems (in near future after forming the discord 7 to 77+ server). Anyone in the IT field can join. Anyone else who wants to pursue a career as an IT professional can join and be exposed to the coding field. Join surveys sent to the discord community and submit your interview questions. Optionally ask the community to help solve the problems. Meet new people and see how they solve a coding challenge versus others.
Enjoy watching 11+ hours of lessons, and know that I have spent 77+ hours plus a decent number of weekends to produce these valuable lesson contents. This course will wisely grow bigger and better with the discord community. Thank you for your participation. You rock!
-
1Introduction. Getting to know the instructor and the content.Video lesson
Welcome message from Benji
-
2What platform are we going to use to write our codes?Video lesson
-
3ResourceText lesson
Check out the resource and review as needed. Take it and own the knowledge. You can always refer to your resources.
Please take a moment to take this survey: https://docs.google.com/forms/d/e/1FAIpQLSfk7bEgb11c3men0VRSpV4Euwx70dNqbL4DsL4qLDE1BKQfrw/viewform?usp=sf_link
Also here is the link to join discord group:
https://discord.gg/B3kawGkqFQ
or try this https://discord.gg/2FZE72sT3P
-
4Find Common Characters inside given two stringsVideo lesson
Given two strings, return common letters
logic,
find short and long strings
shorter string characters can then be compared with longer string characters
if there is a match, add it inside a new string
repeat the steps, finally print new string.
-
5Greatest ascending differenceVideo lesson
/*
Greatest ascending difference
Given integer array, find the greatest difference between two elements:
x & y, where y>x and y comes after x
return -1 if there is no difference.
[4,2,5,8,1] returns 6 because ascending difference is 2 - 8
[6,5,4,3,2,1] returns -1 because there is no ascending difference
*/
-
6Find first occurrence of given value: Find the index value via recursive callVideo lesson
-
7Is the array sorted ? Return true or falseVideo lesson
in this solution, we are solving if given array is sorted. Return true otherwise false.
Note that if we change the index, we can see if the part of the array is sorted or not.
-
81 Read input data from stdin, via Scanner classVideo lesson
We will use stdin box in the onecompiler website to mimic what interviewers use to provide input for analysis. Such idea is key to getting started with coding task, and most importantly learn ways to filter input data such as using java 8 lambda operation. Lambda should not scare you because in this lesson, it will help you write much less code when there is already a lot of lines of code to partly minify the lines.
We will look into how to utilize Scanner and BufferReader classes to read from standard input known as stdin. This logic is an oldest and yet it is still in use in today's world.
I highly recommend to code hands on while you watch the video lesson. You will also have access to the code written in the lesson. See Resources tab that will include the external link to original code at onecompiler website.
Thank you for your hard work. Now you are one step closer being prepared in the interview.
-
92 Read Input from stdin via BufferedReaderVideo lesson
Read values of Stdin using Buffered Reader class.
-
103 Parse and store values inside a list from stdin via Scanner,Video lesson
Note that we take the lesson 1 function and make changes in a new file.
convert the method into return type.
using ArrayList container.
pay attention to method signature, void is removed and Arralist<String> is added. Thus, we must return the list at the end of the method.
Now the method is stores stdin values in a list.
-
114 Get the stdin data as buffered stream and store values inside a list.Video lesson
compare what we did with lesson 3 and see if you notice any differences.
made many mistakes and most of them were to pinpoint what parts you should remember the most. Some other mistakes were mainly because I was occupied with two or more tasks at the time of recording lead to some simple mistakes. At the end of the video, though, I was able to fix all mistakes for your reference.
Have you not joined the discord server? We are waiting for you to arrive! What are you waiting for? Download the discord app on your smart device or use web to join discord channel. See you there.
https://discord.gg/2FZE72sT3P
-
125 Analysis 1: Filter Numbers from parsed data via lambda or regex statementVideo lesson
Analysis of how we can extract numbers from given list of items through stdin box. We will try lambda and regular expression solutions to see which one works for us. Check it out.
-
136 Analysis 2: Filter Text from Stdin via basic regular expressionVideo lesson
-
14Lesson 3 Getting Started:Survey(if not taken):Text lesson
Please take a moment to take this survey: https://docs.google.com/forms/d/e/1FAIpQLSfk7bEgb11c3men0VRSpV4Euwx70dNqbL4DsL4qLDE1BKQfrw/viewform?usp=sf_link
Also here is the link to join discord group: https://discord.gg/2FZE72sT3P
-
15Lesson 4: Understand how getters and setters work in JavaVideo lesson
In this video, you will work alongside to create a simple Machine class to prepare a plain java code. Legacy based, but still, you will learn how to write the functions and more importantly see how constructors were used in this example first hand. You may think this lesson as the first step into object oriented programming, a good refresher for some friends. Please use onecompiler or any similar online compiler to write code on these lessons.
https://onecompiler.com/
See the completed code below in Resources tab.
-
16Lesson 4 Part 2: Apply how getters and setters in your own program.Video lesson
This is the part where we will add the functions/methods. Apply in your own program and compare results. Good luck.
For example, why don't you create another class other than Machine. Name your own program such as Cars, Animals or Shapes. and so on. Good luck.
See the completed code below in Resources tab.
-
17Introduce yourself, return name and job and high school numberQuiz
-
18ResourceText lesson
See the shared link to view the completed code.
-
19Solve this for starters. Easy task.Quiz
-
20IsLetter function: First part of the lesson. Note: these 3 lessons are togetherVideo lesson
Character class contains methods such as isLetter or digit. We will begin utilizing isLetter in this lesson. Please apply and make your own code during or after watching the lessons. Repetition in writing code and improving your existing code are the keys to mastering a decent programming foundation. Good luck.
-
21Find and Separate charactersVideo lesson
In the second part of the video, we continue looking at the data and separate the characters given the index. At the end of the video or while you watch it, please apply the code and practice.
-
22Lecture 8: Get count of given values. Last part of the lesson in the section.Video lesson
-
23Section 3 Quiz - checkupQuiz
Check for understanding.
-
24ResourceText lesson
-
25Find middle value of given integer numbers.Video lesson
Trial and error is what keeps me going. It's the natural existence of learning hands on. On this lesson, I would like you to try out coding as you watch the video or begin trial after the lesson. It is also imperative to make your own variation of the code to cover more use cases. On this one, we looked at two main use cases: finding the middle value of odd and even numbers. Good luck with your practice. See you on the next lesson.
-
26Find middle value of given decimal numbers part 1Video lesson
-
27Find middle value of given decimal numbers part 2Video lesson
-
28ResourceText lesson
Check out the resources. Hope that helps.
-
29Count DuplicatesVideo lesson
Finding the count of each element in given lists.
-
30Sum of n values - Solution in Java and JavaScriptVideo lesson
While we get the sum of all numbers, there was a Math solution that only works for consecutive numbers. So that was the epic failure realized, you will see success nearing the end, duh, that was something :)
-
31Resources and the basic idea for the bonus lessonText lesson
-
32Find missing number in given list of numbers that are consecutiveVideo lesson
Find missing number has one requirement that should be mentioned. Numbers are either consecutive and it can also be between a low and a high numbers. For example 1-10, used in our lesson.
-
33ResourceText lesson
Check out the completed code for your reference. Hope that helps.
-
34Binary numbers and measure distance between 1s while counting 0s.Video lesson
How to use ampersand to check for odd and even numbers.
As in binary case, take 0 as even and 1 as odd to utilize ampersand in the comparison.
Next, figure out how to convert numbers into binary format.
Apply how to use Math.max(var1, var2); // that returns higher value
-
35ResourceText lesson
Check out the Binary Gap Link in the external resources part.
-
36Count vowels of given raw dataVideo lesson
create your own random object data that consists of letters, numbers, characters and be sure to include a few vowels for testing purposes.
create your countVowel( ) method and isVowel( )
create your toOnePiece( ) method, learn it then own it.
Once you are able to add all data values as a string type,
now you may utilize the charAt() function to check for isVowel( )
for example: add a for loop then inside the loop:
if( isVowel(onepiece.charAt(i))){ }
now you can increment the count by 1.
Finally print the count value.
Practice this code a few times and you will be mastering it. Make it better and make it your own.
-
37ResourceText lesson
-
38Keep Unique Chars: Remove repeated characters of a stringVideo lesson
you are given a text that has duplicated values; aabbccdd
create a new string object and set it to your new function: removeDuplicates()
create the function/method now:
removeDuplicates(String text){ }
inside the function above, do the following,
initialize a new string empty.
create a for loop and follow the video lesson to complete the logic.
Learn more about negating in if-then-else block. I highly recomment your own trial and error rather than searching for more resources. You will see one example in this lesson. See if you can make your own logic from it. Take it and own it. Good luck.
-
39Keep on Coding: Reverse string + Reverse words backwardsVideo lesson
Step 1 and 2: Solve by one liner code.
Step 3 and 4: Solve by a while loop
Step 4: Solve by a for loop
Step 5: Reverse given text word by word.
You can see the final code here: https://onecompiler.com/java/3xn69dqas
Downloadable resource is the same as the link above. If it does not work, copy and paste the web address in a browser:
https://onecompiler.com/java/3xn69dqas
That should do the trick. Thank you for your hard work.
-
40ResourceText lesson
You may find the code link here: https://onecompiler.com/java/3xn69dqas
You may also find it in the External Resources.
-
41Part 1 : Swap values in arrays lowest to highest via Temp VariableVideo lesson
-
42Part 2 : Swap even and odd, make left fill with evven, make right fill with oddVideo lesson
-
43Part 3 Swap and sort. Even &odd values sorted from smallest to largestVideo lesson
-
44ResourceText lesson
See original codes for your reference.
-
45Part 1: Reverse int value and int or char arrayVideo lesson
Thanks for listening and watching.
Once you are done here,
Check out the next lesson about ArrayList reversal of random objects.
-
46Part 2: Reverse ArrayList values. Disclaimer: Example used is not type safe.Video lesson
ArrayList reversal for multiple types via single method created for all object types. Although the method is compatible, note that it is not type safe for applications. You may only use this as a logic in your interview or if asked in your future company to perform such a trivial function for some reason /:
Thanks for doing your best. Check out bonus lessons as they may be added time to time.
-
47ResourceText lesson
-
50Success or Failure: An Equality check using Command Line tool.Video lesson
Given text that is passed as an argument compared to the other text set inside the code. Figure out how to compile java code and run after that. This code can run in other platforms that has java installed.
-
51Coding Chains - How to chain methods: Fluent Design Pattern - no resource sorryVideo lesson
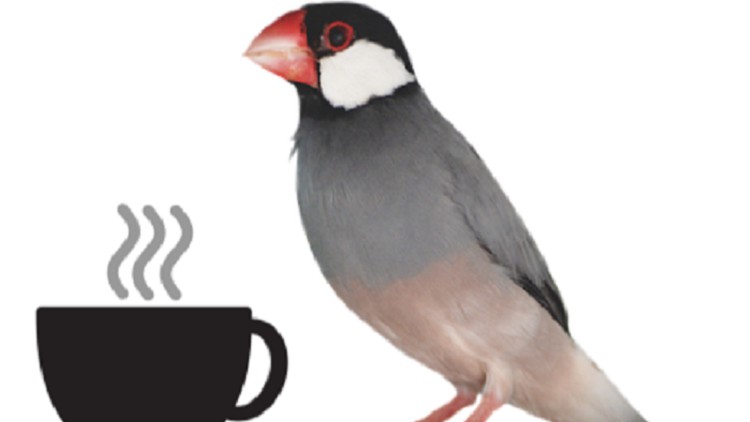
External Links May Contain Affiliate Links read more