Competitive Programming
- Description
- Curriculum
- FAQ
- Reviews
Ready to take your programming skills to the next level? In this course, which will help both novice and advanced programmers alike, you will dominate the algorithms and data structures necessary to do well in contests and to gain a competitive edge over other candidates in software interviews.
There are many tricks which are gained through experience and competitive programmers have a sixth sense when it comes to breaking problems down into the building blocks that make up a solution and which many are reluctant to share. Here I will let you in on the techniques and the applications that are useful for the field, focusing on real problems and how they are solved, while giving you an intuition on what is going on under the hood and why these ideas work.
From dynamic programming to graph algorithms and backtracking, you will get to practise and feel confident about many topics, learning advanced concepts such as union-find disjoint sets, tries and game theory without feeling lost, and to apply new content as soon as you learn it, with over 100 suggested problems, both from past olympiads and online judges and some created by me specifically for this course. All of them come with detailed solutions. With this course, you will be ready to participate in online contests and informatics olympiads, and will have the experience necessary to continue advancing in this field. Are you ready to take this big step in your journey?
-
1About the InstructorVideo lesson
-
2Course PrerequisitesText lesson
-
3Course StructureVideo lesson
-
4What is an Online Judge?Video lesson
-
5Online Judge VerdictsText lesson
-
6Types of Problem in Programming ContestsVideo lesson
-
7I/O for Competitive ProgrammingText lesson
-
8Welcome ContestText lesson
-
9Hints and SolutionsText lesson
-
10Introduction to Section 2 and the Horse Race ProblemVideo lesson
-
11Sorting in C++ Using Custom ComparatorsVideo lesson
-
12Structs and Overloading Comparison OperatorsVideo lesson
-
13Merge SortVideo lesson
-
14QuickSortVideo lesson
-
15DNA Sorting ProblemVideo lesson
-
16Count SortVideo lesson
-
17Football ProblemVideo lesson
-
18Bucket SortVideo lesson
-
19Bucket Sort Solution to the Football ProblemVideo lesson
-
20Problem Set 1Text lesson
-
21Hints and SolutionsText lesson
-
29Stacks: bracket matchingVideo lesson
-
30QueuesVideo lesson
-
31Hash Tables and Hash Sets IntroductionVideo lesson
-
32Unordered (Hash) Sets and Maps in C++Video lesson
-
33Ordered Sets and MapsVideo lesson
-
34DictionariesVideo lesson
-
35Coordinate compressionVideo lesson
-
36Custom Comparators for STL Data StructuresVideo lesson
-
37Problem Set 3Text lesson
-
38Hints and SolutionsText lesson
-
39Hash FunctionsVideo lesson
-
40Extra: Handling CollisionsVideo lesson
-
41Rabin-Karp Case Study: Pattern SearchVideo lesson
-
42Rabin-Karp Case Study: Polynomial Rolling Hash FunctionsVideo lesson
-
43Rabin-Karp Case Study: Sliding Window TechniqueVideo lesson
-
44Sliding Window Technique: Subarray SumsVideo lesson
-
45Problem Set 4Text lesson
-
46Hints and SolutionsText lesson
-
47What are Greedy Algorithms?Video lesson
-
48Greedy Coin ChangeVideo lesson
-
49Demonstrating Correctness Under Contest Time PressureVideo lesson
-
50Timetable ProblemVideo lesson
-
51Interval CoveringVideo lesson
-
52Sort -> SolveVideo lesson
-
53Problem Set 5Text lesson
-
54Hints and SolutionsText lesson
-
55Complete Search IntroductionVideo lesson
-
56Backtracking IntroductionVideo lesson
-
57Backtracking: PermutationsVideo lesson
-
58Backtracking: SubsetsVideo lesson
-
59Pruning: Card GameVideo lesson
-
60Iterative Complete SearchVideo lesson
-
61Problem Set 6Text lesson
-
62Hints and SolutionsText lesson
-
68Top-Down FibonacciVideo lesson
-
69Bottom-Up FibonacciVideo lesson
-
70How to Recognise and Approach DP ProblemsVideo lesson
-
71Maximum One-Dimensional Range SumVideo lesson
-
72Space OptimisationVideo lesson
-
73Optimal Coin Change 1Video lesson
-
74Optimal Coin Change 2Video lesson
-
75Backtracking to Find SolutionsVideo lesson
-
76Prefix SumsVideo lesson
-
77Horses Problem RevisitedVideo lesson
-
78Horses AddendumVideo lesson
-
79Problem Set 8Text lesson
-
80Hints and SolutionsText lesson
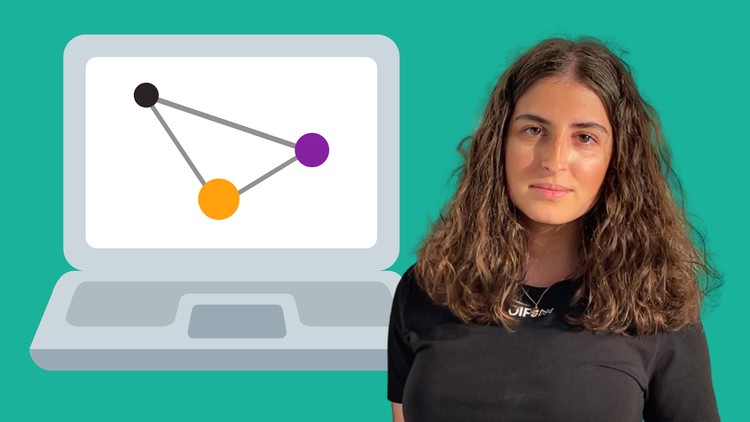
External Links May Contain Affiliate Links read more